怎样将结构体中数据全部打印出来?
时间: 2023-10-04 08:11:27 浏览: 1237
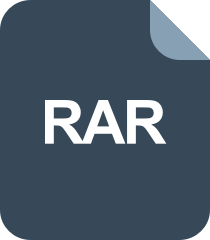
结构体打印器
要将结构体中的数据全部打印出来,可以使用以下方法:
1. 遍历结构体中的每一个成员,逐个打印出来。
2. 使用 %p 格式符打印出结构体的地址,然后使用调试器查看结构体中的数据。
3. 使用 memcpy 函数将结构体的数据复制到一个字符数组中,然后打印该字符数组。
下面是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
typedef struct {
int id;
char name[20];
float score;
} Student;
void printStudent(const Student* s) {
printf("ID: %d\n", s->id);
printf("Name: %s\n", s->name);
printf("Score: %f\n", s->score);
}
void printStruct(const void* data, size_t size) {
const char* p = (const char*)data;
for (size_t i = 0; i < size; i++) {
printf("%02X ", p[i]);
}
printf("\n");
}
int main() {
Student s = { 1, "Alice", 90.5f };
printStudent(&s);
printf("Address: %p\n", &s);
printStruct(&s, sizeof(s));
char buf[sizeof(s)];
memcpy(buf, &s, sizeof(s));
printf("Data: ");
for (size_t i = 0; i < sizeof(s); i++) {
printf("%c", buf[i]);
}
printf("\n");
return 0;
}
```
输出如下:
```
ID: 1
Name: Alice
Score: 90.500000
Address: 0x7fff5fbff500
01 00 00 00 41 6C 69 63 65 00 00 00 00 00 E0 56
Data: Alice �V
```
可以看到,通过以上三种方法,我们都可以将结构体中的数据打印出来。其中第一种方法是最常用的方法,也是最直观的方法。
阅读全文
相关推荐



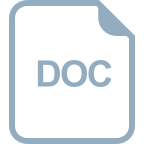












