使用springboot项目实现了图片的上传,并且图片名字随机生成,前端怎么获取???
时间: 2024-03-14 09:43:51 浏览: 23
可以在后端上传图片成功后,将图片的随机生成的名字返回给前端,前端可以通过接口返回的数据来获取图片名字,然后通过拼接图片的访问路径来获取图片。具体实现可以参考以下步骤:
1. 在上传图片成功后,将随机生成的图片名字返回给前端,可以在返回的json数据中添加一个字段来存储图片名字。
2. 前端通过接口返回的数据获取图片名字,例如可以使用axios来发送请求,并在请求成功后获取到图片名字。
3. 在前端页面中,使用拼接的方式来获取图片,例如可以使用类似下面的代码:
```
<img src="http://localhost:8080/images/{{imageName}}" />
```
其中,{{imageName}}是获取到的图片名字,在后端存储图片的时候需要将图片存储在指定的路径下,并且需要设置好静态资源的访问路径,这样前端才能通过拼接路径来获取图片。
相关问题
springboot如何整合腾讯云cos实现上传文件?
可以使用腾讯云官方提供的Java SDK,具体步骤如下:
1. 在pom.xml文件中引入腾讯云cos-java-sdk-v5依赖:
```xml
<dependency>
<groupId>com.qcloud</groupId>
<artifactId>cos_api</artifactId>
<version>5.6.19</version>
</dependency>
```
2. 创建腾讯云cos的配置类:
```java
@Configuration
public class TencentCosConfig {
@Value("${tencent.cos.secretId}")
private String secretId;
@Value("${tencent.cos.secretKey}")
private String secretKey;
@Value("${tencent.cos.region}")
private String region;
@Value("${tencent.cos.bucketName}")
private String bucketName;
@Bean
public COSCredentials cosCredentials() {
return new BasicCOSCredentials(secretId, secretKey);
}
@Bean
public ClientConfig clientConfig() {
ClientConfig clientConfig = new ClientConfig();
clientConfig.setRegion(new Region(region));
return clientConfig;
}
@Bean
public COSClient cosClient() {
return new COSClient(cosCredentials(), clientConfig());
}
@Bean
public String bucketName() {
return bucketName;
}
}
```
其中,secretId和secretKey是腾讯云提供的访问密钥,region是存储桶所在的地域,bucketName是存储桶的名称。可以在配置文件中配置这些变量,这里用@Value注解获取。
3. 在上传文件的Controller中注入cosClient和bucketName,实现文件上传方法:
```java
@RestController
public class FileController {
@Autowired
private COSClient cosClient;
@Autowired
private String bucketName;
@PostMapping("/uploadFile")
public String uploadFile(@RequestParam("file") MultipartFile file) throws Exception {
ObjectMetadata objectMetadata = new ObjectMetadata();
objectMetadata.setContentLength(file.getSize());
objectMetadata.setContentType(file.getContentType());
String fileName = UUID.randomUUID().toString() + "." + FilenameUtils.getExtension(file.getOriginalFilename());
PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, fileName, file.getInputStream(), objectMetadata);
cosClient.putObject(putObjectRequest);
return "https://" + bucketName + ".cos." + "region" + ".myqcloud.com/" + fileName;
}
}
```
这里上传文件的方式为MultipartFile类型,使用Apache Commons IO工具类获取文件后缀名,并用UUID生成随机文件名。然后创建PutObjectRequest对象,调用cosClient的putObject方法上传文件,最后将文件URL返回给前端。
希望以上信息能对你有所帮助。
使用springboot+vue实现验证码登录验证的前后端代码
前端代码:
```vue
<template>
<div>
<el-form :model="form" label-width="100px" ref="form">
<el-form-item label="手机号码" prop="phone">
<el-input v-model="form.phone" placeholder="请输入手机号码"></el-input>
</el-form-item>
<el-form-item label="验证码" prop="captcha">
<el-input v-model="form.captcha" placeholder="请输入验证码" style="width: 200px"></el-input>
<el-button type="primary" @click="sendCaptcha" :disabled="isSending">{{ captchaText }}</el-button>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm">提交</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
import { getCaptcha, login } from '@/api/user'
export default {
data() {
return {
form: {
phone: '',
captcha: ''
},
captchaText: '获取验证码',
isSending: false
}
},
methods: {
sendCaptcha() {
if (!this.form.phone) {
this.$message.error('请输入手机号码')
return
}
if (this.isSending) {
return
}
this.isSending = true
let count = 60
const interval = setInterval(() => {
count--
if (count <= 0) {
clearInterval(interval)
this.captchaText = '重新获取'
this.isSending = false
} else {
this.captchaText = `${count}s后重新获取`
}
}, 1000)
getCaptcha(this.form.phone).then(() => {
this.$message.success('验证码已发送')
}).catch(() => {
clearInterval(interval)
this.captchaText = '重新获取'
this.isSending = false
})
},
submitForm() {
this.$refs.form.validate(valid => {
if (valid) {
login(this.form.phone, this.form.captcha).then(() => {
this.$router.push('/')
}).catch(error => {
this.$message.error(error.message)
})
}
})
}
}
}
</script>
```
后端代码:
```java
@RestController
@RequestMapping("/api/user")
public class UserController {
@Autowired
private RedisUtil redisUtil;
@PostMapping("/captcha")
public ResponseEntity<Object> getCaptcha(@RequestParam String phone) {
// 生成4位随机验证码
String captcha = String.valueOf(new Random().nextInt(8999) + 1000);
// 将验证码保存到redis中,有效期5分钟
redisUtil.set(phone, captcha, 5 * 60);
// TODO 发送短信验证码
return ResponseEntity.ok().build();
}
@PostMapping("/login")
public ResponseEntity<Object> login(@RequestParam String phone, @RequestParam String captcha) {
// 从redis中获取验证码
String redisCaptcha = (String) redisUtil.get(phone);
if (StringUtils.isBlank(redisCaptcha)) {
throw new BusinessException("验证码已失效,请重新获取");
}
if (!StringUtils.equals(redisCaptcha, captcha)) {
throw new BusinessException("验证码错误");
}
// TODO 验证手机号码是否已注册
// TODO 如果未注册,则自动注册
// TODO 生成token返回给前端
return ResponseEntity.ok().build();
}
}
```
相关推荐
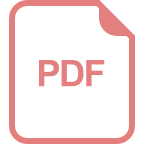
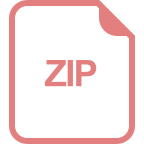
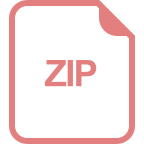
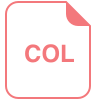
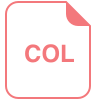
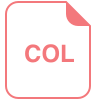
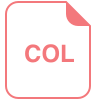
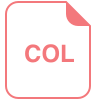







