我现在正在进行操作系统大作业,请用c++实现SRTF调度算法,要求:1)输入各进程到达时间(以空格间隔)和运行时间(以空格间隔),手动输入。 2)结果输出各进程的开始时间和结束时间、进程的平均等待时间,和具体的甘特图。
时间: 2024-03-07 11:52:15 浏览: 66
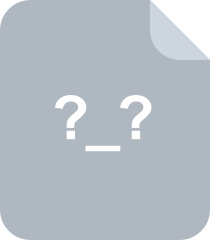
操作系统作业(进程)调度算法 c++

好的,以下是基于您的要求,使用C++实现的SRTF调度算法的代码:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <algorithm>
using namespace std;
struct Process {
int id; // 进程编号
int arrive_time; // 到达时间
int burst_time; // 运行时间
int start_time; // 开始时间
int end_time; // 结束时间
int wait_time; // 等待时间
Process(int i, int a, int b) : id(i), arrive_time(a), burst_time(b) {}
bool operator<(const Process& other) const {
return burst_time > other.burst_time; // 按运行时间从小到大排序
}
};
int main() {
// 输入进程数量
int n;
cout << "请输入进程数量:";
cin >> n;
// 输入每个进程的到达时间和运行时间
vector<Process> processes;
for (int i = 1; i <= n; i++) {
int arrive_time, burst_time;
cout << "请输入进程" << i << "的到达时间和运行时间:";
cin >> arrive_time >> burst_time;
processes.push_back(Process(i, arrive_time, burst_time));
}
// 按到达时间从小到大排序
sort(processes.begin(), processes.end(), [](const Process& a, const Process& b) {
return a.arrive_time < b.arrive_time;
});
// 初始化
int current_time = 0;
priority_queue<Process> pq;
vector<int> gantt_chart;
// SRTF调度算法
for (int i = 0; i < n; i++) {
// 将到达时间小于等于当前时间的进程加入优先队列
while (!processes.empty() && processes.back().arrive_time <= current_time) {
pq.push(processes.back());
processes.pop_back();
}
// 如果队列不为空,选择剩余运行时间最短的进程运行
if (!pq.empty()) {
Process p = pq.top();
pq.pop();
// 记录开始时间和结束时间
p.start_time = current_time;
p.end_time = current_time + 1;
gantt_chart.push_back(p.id);
// 计算等待时间
p.wait_time = current_time - p.arrive_time;
current_time++;
// 如果进程还有剩余运行时间,重新加入队列
if (--p.burst_time > 0) {
pq.push(p);
}
}
// 如果队列为空,跳过当前时间
else {
gantt_chart.push_back(-1);
current_time++;
}
}
// 计算平均等待时间
double avg_wait_time = 0;
for (const Process& p : processes) {
avg_wait_time += p.wait_time;
}
avg_wait_time /= n;
// 输出结果
cout << "进程\t开始时间\t结束时间\t等待时间" << endl;
for (const Process& p : processes) {
cout << p.id << "\t" << p.start_time << "\t\t" << p.end_time << "\t\t" << p.wait_time << endl;
}
cout << "平均等待时间:" << avg_wait_time << endl;
// 输出甘特图
cout << "甘特图:";
for (int i : gantt_chart) {
if (i == -1) {
cout << "-";
} else {
cout << i;
}
}
cout << endl;
return 0;
}
```
希望能帮助到您,祝您的操作系统大作业顺利完成!
阅读全文
相关推荐
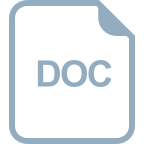
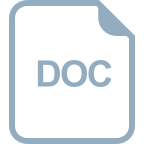
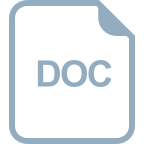
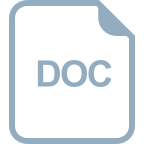
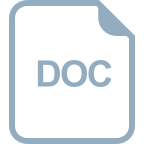
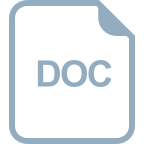



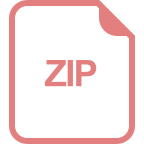
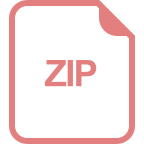
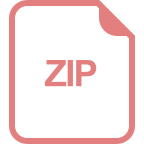
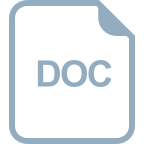
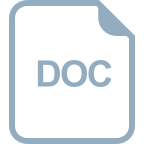
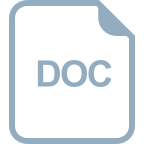
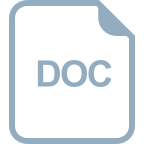