使用C语言实现光谱角监督分类算法
时间: 2023-12-09 12:07:03 浏览: 90
光谱角监督分类算法是一种常用的遥感图像分类方法,可以利用遥感图像中每个像元的光谱特征来进行分类。下面是使用C语言实现光谱角监督分类算法的大致步骤:
1. 读取遥感图像数据,获取像素点的光谱信息。
2. 对光谱进行归一化处理,使得每个波段的数据范围都在[0,1]之间。
3. 定义类别标签,将像素点按照类别进行标记。
4. 计算每个像素点与各个类别之间的光谱角,选择最小的光谱角对应的类别作为该像素点的分类结果。
5. 将分类结果保存到文件中,并可视化分类结果。
下面是一个简单的代码示例,演示了如何使用C语言实现光谱角监督分类算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define NUM_BANDS 10 // 光谱波段数
#define NUM_CLASSES 5 // 类别数
// 定义像素结构体,包含光谱信息和类别标签
struct Pixel {
float spectrum[NUM_BANDS]; // 光谱信息
int label; // 类别标签
};
// 计算两个向量之间的光谱角
float spectral_angle(struct Pixel pixel, float *centroid) {
float dot_product = 0;
float norm1 = 0;
float norm2 = 0;
for(int i = 0; i < NUM_BANDS; i++) {
dot_product += pixel.spectrum[i] * centroid[i];
norm1 += pixel.spectrum[i] * pixel.spectrum[i];
norm2 += centroid[i] * centroid[i];
}
norm1 = sqrt(norm1);
norm2 = sqrt(norm2);
float angle = acos(dot_product / (norm1 * norm2));
return angle;
}
int main() {
// 读取遥感图像数据,获取像素点的光谱信息和类别标签
struct Pixel *pixels = (struct Pixel*)malloc(sizeof(struct Pixel) * NUM_PIXELS);
// ...
// 对光谱进行归一化处理
for(int i = 0; i < NUM_BANDS; i++) {
float max = 0;
float min = 1;
for(int j = 0; j < NUM_PIXELS; j++) {
if(pixels[j].spectrum[i] > max) {
max = pixels[j].spectrum[i];
}
if(pixels[j].spectrum[i] < min) {
min = pixels[j].spectrum[i];
}
}
for(int j = 0; j < NUM_PIXELS; j++) {
pixels[j].spectrum[i] = (pixels[j].spectrum[i] - min) / (max - min);
}
}
// 计算类别的光谱质心
float centroids[NUM_CLASSES][NUM_BANDS];
// ...
// 对每个像素点进行分类
for(int i = 0; i < NUM_PIXELS; i++) {
float min_angle = INFINITY;
int min_label = -1;
for(int j = 0; j < NUM_CLASSES; j++) {
float angle = spectral_angle(pixels[i], centroids[j]);
if(angle < min_angle) {
min_angle = angle;
min_label = j;
}
}
pixels[i].label = min_label;
}
// 将分类结果保存到文件中
FILE *fp = fopen("result.txt", "w");
for(int i = 0; i < NUM_PIXELS; i++) {
fprintf(fp, "%d ", pixels[i].label);
}
fclose(fp);
// 可视化分类结果
// ...
return 0;
}
```
需要注意的是,这只是一个简单的示例代码,实际上光谱角监督分类算法还有很多细节需要处理,例如类别的初始化、光谱质心的选择等。此外,对于大规模的遥感图像数据,还需要考虑如何优化算法的效率和内存占用。
阅读全文
相关推荐
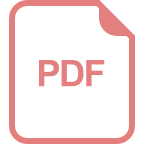
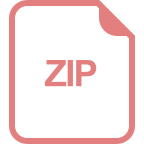
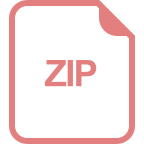
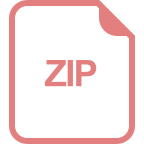
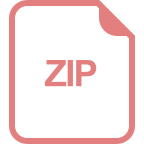
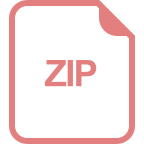
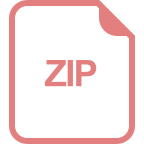
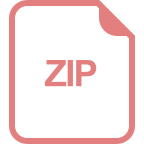
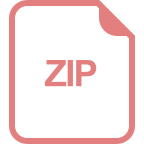
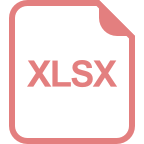
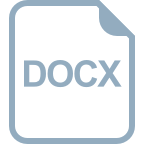
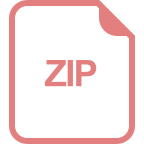
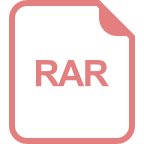
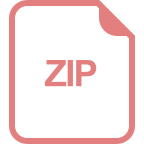
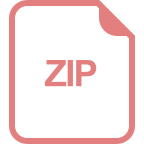