const http = require('http'); const fs = require('fs'); const path = require('path'); const server = http.createServer((req, res) => { // 获取请求的 URL const url = req.url; // 根据请求的 URL 返回对应的文件内容 if (url === '/login') { const filePath = path.join(__dirname, 'login.html'); fs.readFile(filePath, (err, data) => { if (err) { res.writeHead(404, {'Content-Type': 'text/html'}); res.end('404 Not Found'); } else { res.writeHead(200, {'Content-Type': 'text/html'}); res.end(data); } }); } else if (url === '/regist') { const filePath = path.join(__dirname, 'regist.html'); fs.readFile(filePath, (err, data) => { if (err) { res.writeHead(404, {'Content-Type': 'text/html'}); res.end('404 Not Found'); } else { res.writeHead(200, {'Content-Type': 'text/html'}); res.end(data); } }); } else if (url === '/index') { const filePath = path.join(__dirname, 'index.html'); fs.readFile(filePath, (err, data) => { if (err) { res.writeHead(404, {'Content-Type': 'text/html'}); res.end('404 Not Found'); } else { res.writeHead(200, {'Content-Type': 'text/html'}); res.end(data); } }); } else { res.writeHead(404, {'Content-Type': 'text/html'}); res.end('404 Not Found'); } }); server.listen(3000, () => { console.log('Server is running at http://localhost:3000'); });
时间: 2024-03-07 11:53:33 浏览: 22
这段代码是一个使用 Node.js 创建 HTTP 服务器的例子。这个服务器可以返回不同 URL 对应的 HTML 文件内容。具体来说,它做了以下几件事情:
1. 引入 `http`、`fs` 和 `path` 模块。
2. 使用 `http.createServer()` 方法创建一个 HTTP 服务器。
3. 在服务器的回调函数中,获取请求的 URL。
4. 根据 URL 返回对应的 HTML 文件内容。如果文件不存在,则返回 404 错误。
5. 使用 `server.listen()` 方法让服务器监听 3000 端口,并在控制台输出运行信息。
可以看到,这个例子的服务器比较简单,只能返回三个 HTML 文件的内容,如果请求的 URL 不是这三个文件,就返回 404 错误。在实际应用中,我们可能需要更加复杂的服务器逻辑,例如处理 POST 请求、处理 JSON 数据等等。不过这个例子可以帮助我们理解 Node.js 创建 HTTP 服务器的基本步骤。
相关问题
const path = require('path')
### 回答1:
const path = require('path') 是 Node.js 中的一个模块,用于处理文件路径。它提供了一些方法,如 path.join() 和 path.resolve(),可以帮助我们创建和操作文件路径。在使用 Node.js 进行文件操作时,经常会用到这个模块。
### 回答2:
const path = require('path')是Node.js中的一个模块,用来处理文件路径。在Node.js中,文件路径通常使用斜杠(/)作为分隔符。但是不同的操作系统中,分隔符可能不同,比如在Windows中,文件路径使用反斜杠(\)作为分隔符。
path模块提供了一些方法,可以让我们不用担心不同操作系统的分隔符问题,也可以方便地处理文件路径。
path模块的主要方法包括:
1. path.join():将多个路径拼接成一个完整的路径。例如,path.join('/foo', 'bar', 'baz/asdf', 'quux', '..')返回'/foo/bar/baz/asdf'。
2. path.resolve():将相对路径解析为绝对路径。例如,path.resolve('/foo/bar', './baz')返回'/foo/bar/baz'。
3. path.dirname():返回路径中的目录名。例如,path.dirname('/foo/bar/baz/asdf/quux.txt')返回'/foo/bar/baz/asdf'。
4. path.basename():返回路径中的文件名。例如,path.basename('/foo/bar/baz/asdf/quux.txt')返回'quux.txt'。
5. path.extname():返回路径中的扩展名。例如,path.extname('/foo/bar/baz/asdf/quux.txt')返回'.txt'。
除了这些基本方法,path模块还提供了一些其他方法,例如:path.parse()将路径解析为对象的形式,path.format()将路径对象还原为字符串的形式等。
总之,const path = require('path')是Node.js中的一个核心模块,用来处理文件路径,可以让我们更方便地操作文件。如果我们需要在Node.js应用程序中处理文件路径,使用path模块是一个很好的选择。
### 回答3:
const path = require('path')是Node.js中常用的模块之一,它的主要作用是简化操作文件路径的过程,方便开发者在处理文件路径时避免出错。
当我们在使用Node.js编写代码时,常常需要操作文件路径,这时候就可以引入path模块。这个模块中提供了很多有用的方法,比如join()、resolve()、dirname()、basename()和extname()等,这些方法可以帮助我们在不同操作系统环境下操作文件路径。
例如,在Windows环境下,路径需要使用反斜杠(\)作为分隔符,而在Linux和Mac OS X环境下则需要使用斜杠(/)作为分隔符。使用path模块提供的join()方法,我们可以通过代码自动根据不同操作系统的规则生成正确的文件路径。这对于在开发过程中避免出现问题,提高代码的可移植性和可读性都十分重要。
除了提供了一些实用方法外,path模块还具有一些特殊的属性。比如,path.sep表示当前操作系统的路径分隔符,path.delimiter表示当前操作系统的环境变量分隔符,path.basename()方法可以获取文件名等等。这些属性和方法的使用能够在开发过程中提高效率和准确性,避免出现因操作系统不同而导致的问题。
总之,const path = require('path')是一个十分实用的Node.js模块,它能够帮助开发者简化处理文件路径的过程,提高代码的可移植性和可读性,同时也避免出现因操作系统不同而导致的问题。
const express = require('express'); const app = express(); const http = require('http').createServer(app); const io = require('socket.io')(http);
这段代码使用了 Express 和 Socket.IO 库创建了一个基于 Node.js 的 Web 应用程序,并创建了一个 HTTP 服务器实例。其中,Express 是一个常用的 Web 开发框架,可以帮助我们更方便地创建 Web 应用程序。Socket.IO 是一个支持实时双向通信的 JavaScript 库,可以在客户端和服务器之间进行实时通信。
首先,我们通过 `const express = require('express')` 引入了 Express 库,并创建了一个 Express 应用程序实例,存储在 `app` 变量中。然后,我们通过 `const http = require('http').createServer(app)` 创建了一个 HTTP 服务器实例,并将 `app` 应用程序实例作为参数传递给 `createServer()` 方法。这样,我们就可以通过这个 HTTP 服务器实例来监听客户端请求,并响应这些请求。
接下来,我们使用 `const io = require('socket.io')(http)` 创建了一个 Socket.IO 实例,并将 `http` 服务器实例作为参数传递给 `socket.io()` 方法。这样,我们就可以在客户端和服务器之间建立实时的双向通信,并使用 Socket.IO 提供的 API 进行数据传输和事件监听。
相关推荐
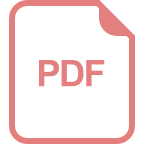
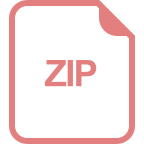
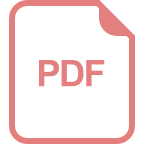













