用unity写一个迷宫代码出来
时间: 2023-05-21 08:05:32 浏览: 228
好的,以下是一个简单的 Unity 迷宫生成器代码(基于深度优先搜索):
```c#
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MazeGenerator : MonoBehaviour
{
public int width, height;
public GameObject wallPrefab;
public float wallLength = 1;
private bool[,] visited;
private List<GameObject> walls = new List<GameObject>();
void Start()
{
visited = new bool[width, height];
GenerateMaze(0, 0);
// 删除外墙
DestroyWall(0, height/2);
DestroyWall(width/2, 0);
DestroyWall(width-1, height/2);
DestroyWall(width/2, height-1);
}
void GenerateMaze(int x, int y)
{
visited[x, y] = true;
// 随机排序方向
List<int> directions = new List<int>() {0, 1, 2, 3};
Shuffle(directions);
foreach (int direction in directions)
{
int nx = x, ny = y;
if (direction == 0) nx += 1;
else if (direction == 1) nx -= 1;
else if (direction == 2) ny += 1;
else if (direction == 3) ny -= 1;
// 如果新的位置在范围内且未访问,拆掉两格之间的墙,递归生成
if (nx >= 0 && nx < width && ny >= 0 && ny < height && !visited[nx, ny])
{
GameObject wall = Instantiate(wallPrefab, transform);
if (direction == 0) // right
{
wall.transform.position = new Vector3(x*wallLength + wallLength/2, 0, y*wallLength);
wall.transform.localScale = new Vector3(wallLength, wallLength*2, 1);
}
else if (direction == 1) // left
{
wall.transform.position = new Vector3(x*wallLength - wallLength/2, 0, y*wallLength);
wall.transform.localScale = new Vector3(wallLength, wallLength*2, 1);
}
else if (direction == 2) // up
{
wall.transform.position = new Vector3(x*wallLength, 0, y*wallLength + wallLength/2);
wall.transform.localScale = new Vector3(1, wallLength*2, wallLength);
}
else if (direction == 3) // down
{
wall.transform.position = new Vector3(x*wallLength, 0, y*wallLength - wallLength/2);
wall.transform.localScale = new Vector3(1, wallLength*2, wallLength);
}
walls.Add(wall);
GenerateMaze(nx, ny);
}
}
}
void DestroyWall(int x, int y)
{
walls.Remove(walls.Find(wall =>
wall.transform.position == new Vector3(x*wallLength, 0, y*wallLength)));
Destroy(walls[walls.Count-1]);
}
static void Shuffle<T>(List<T> list)
{
for (int i = list.Count - 1; i > 0; i--)
{
int j = Random.Range(0, i+1);
T temp = list[i];
list[i] = list[j];
list[j] = temp;
}
}
}
```
在 Unity 中使用此代码创建一个空 GameObject,并为其添加 MazeGenerator 脚本,调整其参数以更改迷宫大小和墙体长度。生成的迷宫将自动删除四个外侧墙,并生成 Unity GameObjects 表示每一堵墙。
阅读全文
相关推荐

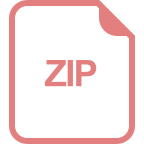
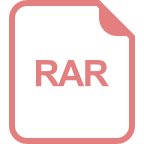
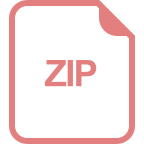
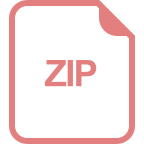
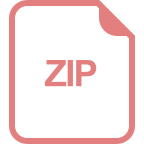
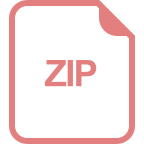
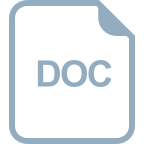
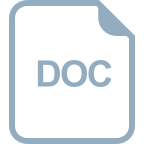
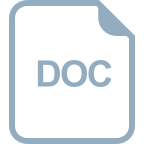
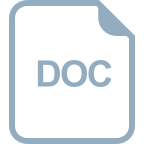
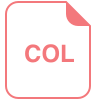
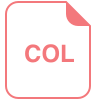
