merged_array = np.column_stack((array1, array2))例子
时间: 2024-03-05 19:54:45 浏览: 73
好的,假设`array1`为`[1, 2, 3]`,`array2`为`[4, 5, 6]`,那么使用`np.column_stack()`函数将它们合并后的结果为:
```python
merged_array = np.column_stack((array1, array2))
print(merged_array)
```
输出结果为:
```
array([[1, 4],
[2, 5],
[3, 6]])
```
其中,第一列为`array1`的元素,第二列为`array2`的元素,它们按列合并成了一个2列3行的数组`merged_array`。
相关问题
python:T_w, M_sw, M_cw = TT * np.ones((68446, 1)), MM * np.ones((68446, 1)), SS * np.ones((68446 1)),怎么把这三个合成一个(68446,3)的ndarray,并获取ndarray的第一个数组
你可以使用 `numpy` 库中的 `column_stack` 函数将三个一维数组按列合并成一个二维数组,然后使用索引获取第一个数组,代码如下:
```
import numpy as np
T_w, M_sw, M_cw = TT * np.ones((68446, 1)), MM * np.ones((68446, 1)), SS * np.ones((68446, 1))
merged_array = np.column_stack((T_w, M_sw, M_cw))
first_array = merged_array[:, 0] # 获取第一个数组
print(first_array)
```
这样就可以将 `T_w`、`M_sw` 和 `M_cw` 合并成一个大小为 `(68446, 3)` 的名为 `merged_array` 的二维数组,并获取其中的第一个数组 `first_array`。
python:怎么把这三个合成一个大小是(68446,3)的ndarray:T_w, M_sw, M_cw = TT * np.ones((cood.shape[0], 1)), MM * np.ones((cood.shape[0], 1)), SS * np.ones((cood.shape[0], 1))
你可以使用 `numpy` 库中的 `column_stack` 函数将三个一维数组按列合并成一个二维数组:
```
import numpy as np
T_w, M_sw, M_cw = TT * np.ones((cood.shape[0], 1)), MM * np.ones((cood.shape[0], 1)), SS * np.ones((cood.shape[0], 1))
merged_array = np.column_stack((T_w, M_sw, M_cw))
```
这样就可以将 `T_w`、`M_sw` 和 `M_cw` 合并成一个大小为 `(68446, 3)` 的名为 `merged_array` 的二维数组。
阅读全文
相关推荐
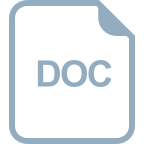
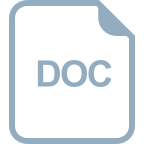
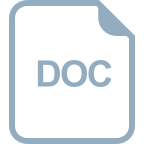



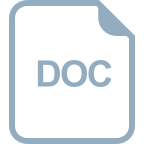
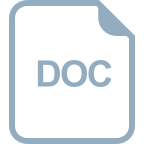
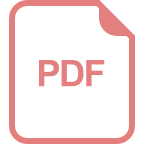
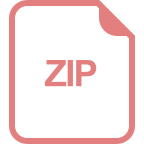
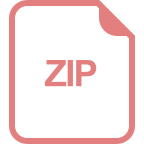
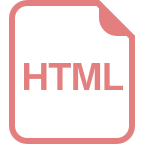
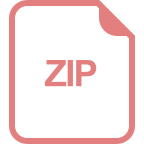
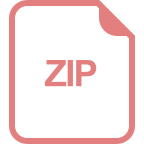
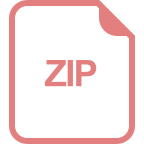
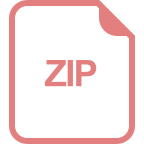