public InputStream getInputStreamFromDcs(String urlStr) throws IOException { URL url = new URL(urlStr); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setConnectTimeout(3 * 1000); //防止屏蔽程序抓取而返回403错误 conn.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 5.0; Windows NT; DigExt)"); //得到输入流 InputStream inputStream = conn.getInputStream(); return inputStream; } 这段代码是干嘛的
时间: 2024-04-23 20:25:21 浏览: 127
这段代码是定义了一个`getInputStreamFromDcs()`方法,用于获取一个URL地址所指向资源的输入流`InputStream`对象。方法实现包括以下几个步骤:
1. 将`urlStr`字符串转换为一个`URL`对象
2. 调用`openConnection()`方法获取该URL地址的`HttpURLConnection`连接对象
3. 设置连接超时时间为3秒
4. 设置请求头信息,模拟浏览器请求,避免被屏蔽
5. 调用`getInputStream()`方法获取输入流对象
6. 将该输入流返回
该方法通常用于从网络上下载文件或资源,可以通过该输入流读取到网络上的资源内容,例如图片、文本、二进制文件等。
相关问题
springboot根据uRL下载文件到本地
可以使用SpringBoot来实现根据URL下载文件到本地的功能。以下是一个示例代码:
```java
@GetMapping("/download")
public void download(@RequestParam("url") String urlStr, HttpServletResponse response) throws IOException {
URL url = new URL(urlStr);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 5.0; Windows NT; DigExt)");
try (InputStream inputStream = conn.getInputStream();
OutputStream outputStream = new FileOutputStream("路径/文件名")) {
byte[] buffer = new byte<span class="em">1</span><span class="em">2</span>
#### 引用[.reference_title]
- *1* [SpringBoot实现通过url下载pdf到本地](https://blog.csdn.net/MCmango/article/details/113937695)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [Vue+SpringBoot根据url返回文件流给前端下载文件(多个图片zip下载)](https://blog.csdn.net/zcl520sy/article/details/130142189)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
一个url地址返回的是一个流,请使用java代码实现获取返回的流的文件名并将流文件写到服务器的/home/temp目录中
### 回答1:
首先,你需要使用 Java 的 `URL` 类来打开一个连接到指定 URL 的连接,然后使用 `URLConnection` 类的 `getInputStream` 方法来获取输入流。
下面是一个例子:
```java
URL url = new URL("http://www.example.com/file.txt");
URLConnection connection = url.openConnection();
InputStream stream = connection.getInputStream();
```
然后,你可以使用 Java 的 `BufferedReader` 类来读取输入流中的数据。
接下来,你可以使用 Java 的 `FileOutputStream` 类来将读取到的数据写入文件中。
下面是一个例子:
```java
BufferedReader reader = new BufferedReader(new InputStreamReader(stream));
String line;
while ((line = reader.readLine()) != null) {
// do something with the line
}
reader.close();
// 获取文件名
String fileName = connection.getHeaderField("Content-Disposition");
if (fileName != null) {
fileName = fileName.replaceFirst("(?i)^.*filename=\"?([^\"]+)\"?.*$", "$1");
} else {
// 如果文件名不存在,则使用默认文件名
fileName = "default.txt";
}
// 将流文件写入服务器的 /home/temp 目录中
FileOutputStream outputStream = new FileOutputStream("/home/temp/" + fileName);
while ((line = reader.readLine()) != null) {
outputStream.write(line.getBytes());
}
outputStream.close();
```
请注意,在上面的例子中,我们使用了正则表达式来解析文件名,这是因为 `Content-Disposition` 头部的格式可能会有所不同。你也可以使用其他方法来获取文件名。
### 回答2:
可以使用以下代码实现获取返回流的文件名并将其写到服务器指定目录下:
```java
import java.io.*;
import java.net.*;
public class FileDownloader {
public static void main(String[] args) throws IOException {
String urlStr = "http://example.com/sample.pdf"; // 替换为需要下载的URL地址
String saveFolder = "/home/temp/"; // 文件保存目录,替换为服务器指定目录
// 创建URL对象
URL url = new URL(urlStr);
// 打开URL连接
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
// 获取文件名
String fileName = getFileName(conn);
// 创建文件输出流
FileOutputStream fos = new FileOutputStream(saveFolder + fileName);
// 获取输入流
InputStream is = conn.getInputStream();
// 设置缓冲区大小
byte[] buffer = new byte[1024];
int len;
// 将输入流写入文件输出流
while ((len = is.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
// 关闭输入流、输出流
fos.close();
is.close();
System.out.println("文件下载完成!");
}
// 获取文件名
private static String getFileName(HttpURLConnection conn) {
// 获取Content-Disposition响应头字段
String disposition = conn.getHeaderField("Content-Disposition");
String fileName = "";
if (disposition != null) {
int index = disposition.indexOf("filename=");
if (index > 0) {
fileName = disposition.substring(index + 10, disposition.length() - 1);
}
} else {
// 未返回Content-Disposition字段时,从URL中提取文件名
fileName = conn.getURL().getFile();
fileName = fileName.substring(fileName.lastIndexOf("/") + 1);
}
try {
// 解码文件名
fileName = URLDecoder.decode(fileName, "UTF-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return fileName;
}
}
```
该代码通过URL对象打开连接,并使用HttpURLConnection获取输入流。同时,通过获取Content-Disposition响应头字段或从URL中提取文件名,确定文件名并进行解码。最后,该代码通过文件输出流将输入流写入文件。
你需要将`urlStr`替换为需要下载的URL地址,并将`saveFolder`替换为服务器指定的目录路径。
阅读全文
相关推荐
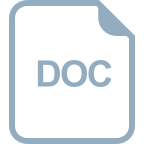
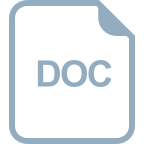
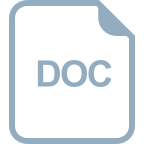

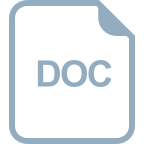
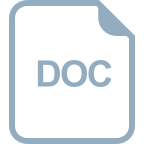
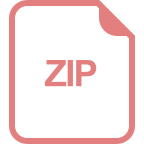
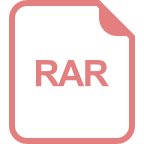
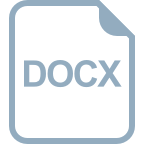
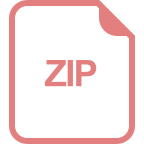
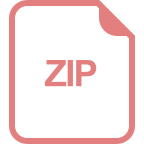
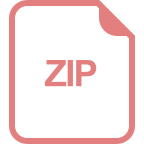