#pragma once #include <opencv2/core/core.hpp> #include <opencv2/highgui/highgui.hpp> #include <opencv2/imgproc/imgproc.hpp> #include<iostream> #include<vector> using namespace std; using namespace cv; // Lớp chứa các blob class Blob { public: int xmax, xmin, ymax, ymin, xcenter, ycenter; vector<Point> region; int width, height; int size; bool isInBlob(Point a) { if (a.x >= xmin - 2 && a.x <= xmax + 2 && a.y >= ymin - 2 && a.y <= ymax + 2) return true; return false; } void Add(Point a) { region.push_back(a); xmax = max(a.x, xmax); xmin = min(a.x, xmin); ymin = min(a.y, ymin); ymax = max(a.y, ymax); xcenter = (xmax + xmin) / 2; ycenter = (ymax + ymin) / 2; size = (xmax - xmin) * (ymax - ymin); width = xmax - xmin; height = ymax - ymin; } Blob() { xmax = ymax = xcenter = ycenter = size = 0; width = height = 0; xmin = ymin = INT_MAX; } ~Blob() {} };
时间: 2023-06-17 08:04:21 浏览: 90
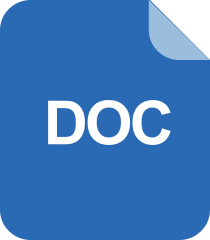
#pragma once用法
这段代码定义了一个名为 Blob 的类,用于存储图像中的 blob 区域的信息。Blob 类包含以下属性:
- xmax、xmin、ymax、ymin:blob 区域的最大/最小坐标值,用于计算 blob 区域的宽度和高度。
- xcenter、ycenter:blob 区域的中心点坐标。
- region:存储 blob 区域中的所有像素点坐标。
- width、height:blob 区域的宽度和高度。
- size:blob 区域的大小。
- Add(Point a):将一个像素点添加到 blob 区域中。
- isInBlob(Point a):判断一个像素点是否在 blob 区域中。
在实际使用中,可以使用该类来实现图像中的目标检测、分割等任务。
阅读全文
相关推荐
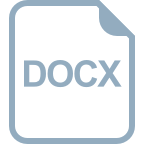
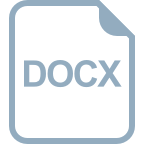
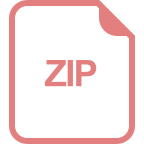
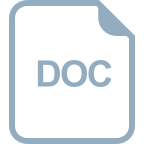
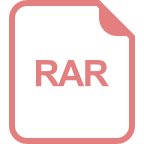
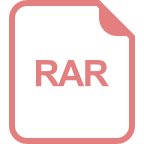
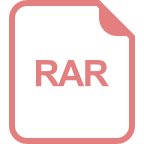
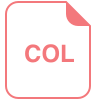
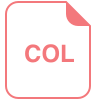
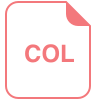


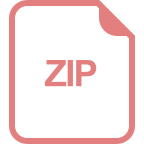
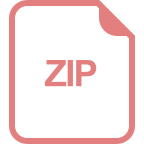