kotlin中怎么把list打印出来
时间: 2023-10-02 13:04:23 浏览: 96
在Kotlin中,您可以使用以下方法打印List的内容:
```kotlin
fun main() {
val list = listOf("apple", "banana", "orange")
println(list)
}
```
在此示例中,我们首先创建了一个包含三个字符串元素的List对象。然后,我们使用println()函数打印List对象。由于Kotlin具有良好的类型推断功能,因此可以直接将List对象传递给println()函数,而无需使用任何格式化或迭代器等。
当然,如果您需要更复杂的格式化或处理逻辑,您可以使用forEach()函数或for循环迭代List并执行所需的操作。
相关问题
kotlin中怎么把list转成jsonobject打印出来
要将Kotlin中的List转换为JSONObject,您需要使用Kotlin的标准库中提供的JSON库。以下是一个示例代码,说明如何将List转换为JSONObject并将其打印出来:
```kotlin
import org.json.JSONObject
fun main() {
val list = listOf("apple", "banana", "orange")
val jsonObject = JSONObject()
jsonObject.put("fruits", list)
println(jsonObject.toString())
}
```
在此示例中,我们首先创建了一个包含三个字符串元素的List对象。然后,我们创建了一个空的JSONObject对象,并将List添加到该对象中作为名为“fruits”的属性。最后,我们使用toString()函数将JSONObject对象转换为字符串并打印它。
请注意,这只是一个简单的示例,实际应用中代码可能需要更复杂的逻辑。
kotlin控制list每10条打印
您可以使用Kotlin的扩展函数来实现这个需求。以下是一个示例代码:
```kotlin
fun <T> List<T>.printEveryN(n: Int) {
require(n > 0) { "n should be positive" }
this.chunked(n).forEachIndexed { index, chunk ->
println("Batch ${index + 1}:")
chunk.forEach { println(it) }
}
}
```
使用示例:
```kotlin
val list = listOf(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18)
list.printEveryN(10)
```
输出:
```
Batch 1:
1
2
3
4
5
6
7
8
9
10
Batch 2:
11
12
13
14
15
16
17
18
```
这个函数接受一个参数`n`,表示每n个元素打印一次。函数首先检查参数`n`是否为正数,然后使用`chunked`函数将原始列表分成多个子列表,每个子列表包含`n`个元素。最后,使用`forEachIndexed`函数遍历每个子列表,并在控制台上打印它们的元素。
相关推荐
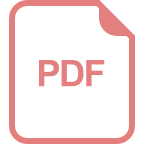












