对DAGM进行缺陷检测的python代码
时间: 2023-08-10 22:09:04 浏览: 46
好的,以下是一个使用 TensorFlow 对 DAGM 数据集进行缺陷检测的 Python 代码示例:
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# 加载数据集
train_data = np.load('dagm_train_data.npy')
train_labels = np.load('dagm_train_labels.npy')
test_data = np.load('dagm_test_data.npy')
test_labels = np.load('dagm_test_labels.npy')
# 创建模型
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(16, (3, 3), activation='relu', input_shape=(128, 128, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(32, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# 编译模型
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
# 训练模型
history = model.fit(train_data, train_labels, epochs=10, batch_size=32, validation_data=(test_data, test_labels))
# 评估模型
loss, accuracy = model.evaluate(test_data, test_labels)
print('Test accuracy:', accuracy)
# 绘制训练和验证准确率曲线
plt.plot(history.history['accuracy'], label='accuracy')
plt.plot(history.history['val_accuracy'], label='val_accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.ylim([0.5, 1])
plt.legend(loc='lower right')
plt.show()
```
在这个例子中,我们使用了 DAGM 数据集来训练和测试模型。我们创建了一个包含三个卷积层和两个全连接层的神经网络,并使用了 relu 激活函数和二进制交叉熵损失函数来训练模型。训练数据和测试数据都以 numpy 数组的形式加载,并保存在 npy 文件中。在训练过程中,我们使用了验证数据来检查模型的泛化能力。最后,我们评估了模型的准确性,并绘制了训练和验证准确率曲线。请注意,这只是一个简单的示例代码,实际上您需要根据您的具体问题来调整模型的架构和参数。
相关推荐
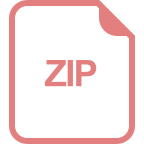
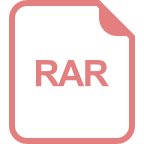
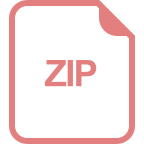

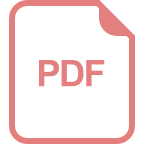
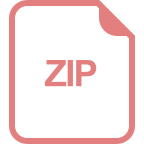
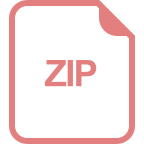
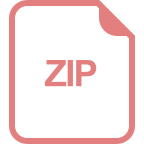
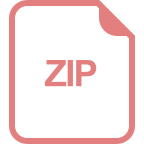
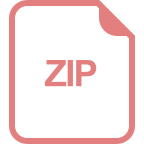
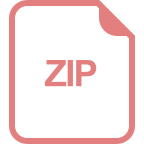
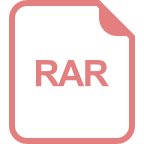
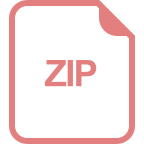