无法连接到 wss://127.0.0.1:8200/websocket
时间: 2023-10-05 22:14:29 浏览: 226
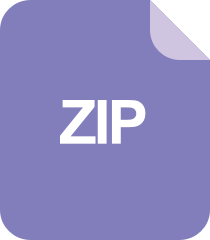
websocket 解决链接失败问题
这个错误通常是由于无法连接到本地主机上运行的 WebSocket 服务器引起的。你可以尝试以下步骤来解决这个问题:
1. 确认 WebSocket 服务器是否已启动并正在运行。你可以通过检查服务器的日志来确认。
2. 检查你的网络连接是否正常。尝试连接到其他 WebSocket 服务器,看看是否也会遇到相同的问题。
3. 检查你的防火墙设置,确保允许来自本地主机上的 WebSocket 服务器的传入连接。
4. 检查 WebSocket 客户端的代码,确保你正确地指定了 WebSocket 服务器的地址和端口号。
如果这些步骤都没有解决问题,可以尝试重新安装 WebSocket 服务器或与其开发者联系以获取帮助。
阅读全文
相关推荐
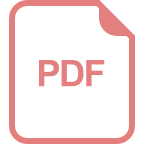
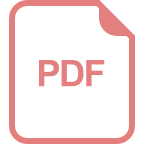

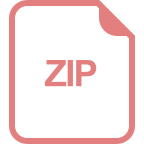
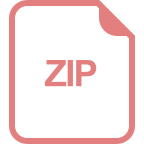
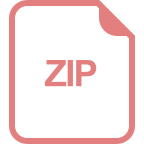
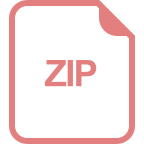
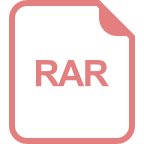
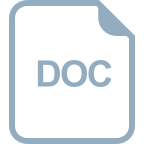
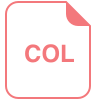
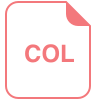
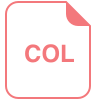
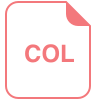
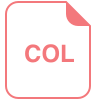



