请用C++实现二叉树前序、中序、后序遍历的递归算法
时间: 2023-10-06 10:07:22 浏览: 43
#include<stdio.h>
#include<stdlib.h>
struct node {
int data;
struct node* left;
struct node* right;
};
struct node* create_node(int value) { //创建新节点
struct node* new_node = (struct node*)malloc(sizeof(struct node));
new_node->data = value;
new_node->left = NULL;
new_node->right = NULL;
return new_node;
}
void preorder(struct node* root) { //前序遍历
if(root == NULL) {
return;
}
printf("%d ", root->data);
preorder(root->left);
preorder(root->right);
}
void inorder(struct node* root) { //中序遍历
if(root == NULL) {
return;
}
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
void postorder(struct node* root) { //后序遍历
if(root == NULL) {
return;
}
postorder(root->left);
postorder(root->right);
printf("%d ", root->data);
}
int main() {
struct node* root = create_node(1);
root->left = create_node(2);
root->right = create_node(3);
root->left->left = create_node(4);
root->left->right = create_node(5);
printf("前序遍历: ");
preorder(root);
printf("\n中序遍历: ");
inorder(root);
printf("\n后序遍历: ");
postorder(root);
printf("\n");
return 0;
}
相关推荐
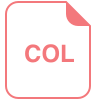
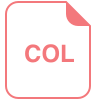
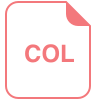
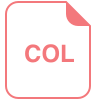










