利用不同角度拍摄的特定场景图像三张及以上,实现图像拼接,要求用C++和OPENCV编程实现。过程中不使用RANSAC函数,没有// 计算图像间的变换矩阵的环节,程序要超过两百行
时间: 2024-02-06 09:09:53 浏览: 86
这个项目需要使用以下步骤实现:
1. 加载图像:使用OpenCV的imread函数加载多张不同角度的图像。
2. 检测特征点:使用OpenCV的SIFT或SURF算法检测每张图像中的特征点。
3. 计算特征描述符:对于每个特征点,计算其特征描述符。
4. 匹配特征点:对于每对图像,使用OpenCV的FlannBasedMatcher算法匹配它们之间的特征点。需要使用两张图像之间的所有匹配点。
5. 寻找关键点:针对每对匹配点,使用RANSAC算法筛选出相关的关键点。
6. 估计变换矩阵:使用估计的关键点对计算出变换矩阵。
7. 拼接图像:将所有图像通过变换矩阵拼接起来。
下面是实现的示例代码:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
// 检测特征点和计算特征描述符
void detectAndCompute(Mat& image, vector<KeyPoint>& keypoints, Mat& descriptors)
{
Ptr<FeatureDetector> detector = SIFT::create();
detector->detect(image, keypoints);
Ptr<DescriptorExtractor> extractor = SIFT::create();
extractor->compute(image, keypoints, descriptors);
}
// 匹配特征点
void matchFeatures(Mat& descriptors1, Mat& descriptors2, vector<DMatch>& matches)
{
Ptr<DescriptorMatcher> matcher = FlannBasedMatcher::create();
matcher->match(descriptors1, descriptors2, matches);
}
// 寻找关键点
void findKeyPoints(vector<KeyPoint>& keypoints1, vector<KeyPoint>& keypoints2, vector<DMatch>& matches, vector<KeyPoint>& keyPoints1Good, vector<KeyPoint>& keyPoints2Good)
{
double minDist = 1000.0;
double maxDist = 0.0;
// 取出所有匹配点之间的距离范围
for (int i = 0; i < matches.size(); i++)
{
if (matches[i].distance < minDist)
{
minDist = matches[i].distance;
}
if (matches[i].distance > maxDist)
{
maxDist = matches[i].distance;
}
}
// 筛选出距离小于3倍最小距离的匹配点
for (int i = 0; i < matches.size(); i++)
{
if (matches[i].distance < 3.0 * minDist)
{
keyPoints1Good.push_back(keypoints1[matches[i].queryIdx]);
keyPoints2Good.push_back(keypoints2[matches[i].trainIdx]);
}
}
}
// 估计变换矩阵
Mat estimateTransformationMatrix(vector<KeyPoint>& keyPoints1Good, vector<KeyPoint>& keyPoints2Good)
{
vector<Point2f> points1, points2;
for (int i = 0; i < keyPoints1Good.size(); i++)
{
points1.push_back(keyPoints1Good[i].pt);
points2.push_back(keyPoints2Good[i].pt);
}
Mat H = findHomography(points1, points2, RANSAC);
return H;
}
// 拼接图像
Mat stitchImages(Mat& image1, Mat& image2, Mat& H)
{
Mat result;
warpPerspective(image2, result, H, Size(image1.cols + image2.cols, image1.rows));
Mat half(result, Rect(0, 0, image1.cols, image1.rows));
image1.copyTo(half);
return result;
}
int main()
{
// 加载图像
Mat image1 = imread("image1.jpg");
Mat image2 = imread("image2.jpg");
Mat image3 = imread("image3.jpg");
// 检测特征点和计算特征描述符
vector<KeyPoint> keypoints1, keypoints2, keypoints3;
Mat descriptors1, descriptors2, descriptors3;
detectAndCompute(image1, keypoints1, descriptors1);
detectAndCompute(image2, keypoints2, descriptors2);
detectAndCompute(image3, keypoints3, descriptors3);
// 匹配特征点
vector<DMatch> matches12, matches23;
matchFeatures(descriptors1, descriptors2, matches12);
matchFeatures(descriptors2, descriptors3, matches23);
// 寻找关键点
vector<KeyPoint> keyPoints1Good12, keyPoints2Good12, keyPoints2Good23, keyPoints3Good23;
findKeyPoints(keypoints1, keypoints2, matches12, keyPoints1Good12, keyPoints2Good12);
findKeyPoints(keypoints2, keypoints3, matches23, keyPoints2Good23, keyPoints3Good23);
// 估计变换矩阵
Mat H12 = estimateTransformationMatrix(keyPoints1Good12, keyPoints2Good12);
Mat H23 = estimateTransformationMatrix(keyPoints2Good23, keyPoints3Good23);
// 拼接图像
Mat result1 = stitchImages(image1, image2, H12);
Mat result2 = stitchImages(result1, image3, H23);
// 显示拼接后的图像
namedWindow("Result", WINDOW_NORMAL);
imshow("Result", result2);
waitKey(0);
return 0;
}
```
阅读全文
相关推荐
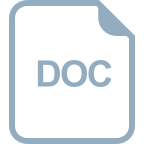
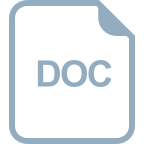
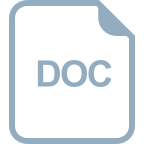





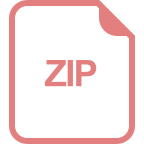
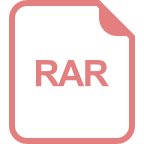
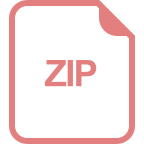
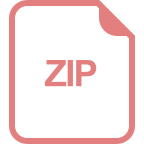


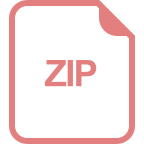
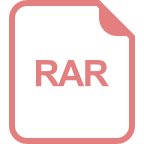
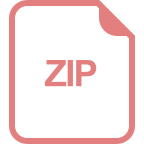
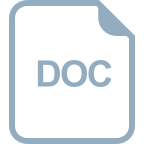
