java写一个别踩白块
时间: 2023-12-20 14:07:39 浏览: 134
好的,别踩白块是一款经典的小游戏,下面给出一个使用Java语言编写的简单版别踩白块游戏的代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class DontTapTheWhiteTile extends JPanel implements KeyListener {
private static final long serialVersionUID = 1L;
private static final int ROWS = 4;
private static final int COLS = 4;
private static final int TILE_SIZE = 100;
private static final int BORDER_WIDTH = 10;
private static final int SCREEN_WIDTH = COLS * (TILE_SIZE + BORDER_WIDTH) + BORDER_WIDTH;
private static final int SCREEN_HEIGHT = ROWS * (TILE_SIZE + BORDER_WIDTH) + BORDER_WIDTH;
private int[][] tiles;
private int currentRow;
private int currentCol;
private boolean gameOver;
public DontTapTheWhiteTile() {
tiles = new int[ROWS][COLS];
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
tiles[row][col] = (row * COLS + col) % 2; // 0 for black, 1 for white
}
}
currentRow = ROWS - 1;
currentCol = 0;
gameOver = false;
setPreferredSize(new Dimension(SCREEN_WIDTH, SCREEN_HEIGHT));
addKeyListener(this);
setFocusable(true);
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
if (tiles[row][col] == 0) { // black tile
g.setColor(Color.BLACK);
} else { // white tile
g.setColor(Color.WHITE);
}
g.fillRect(col * (TILE_SIZE + BORDER_WIDTH) + BORDER_WIDTH,
row * (TILE_SIZE + BORDER_WIDTH) + BORDER_WIDTH, TILE_SIZE, TILE_SIZE);
}
}
}
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_LEFT) {
if (currentCol > 0) {
if (tiles[currentRow][currentCol - 1] == 1) { // stepped on white tile
gameOver = true;
} else {
currentCol--;
}
}
} else if (e.getKeyCode() == KeyEvent.VK_RIGHT) {
if (currentCol < COLS - 1) {
if (tiles[currentRow][currentCol + 1] == 1) { // stepped on white tile
gameOver = true;
} else {
currentCol++;
}
}
} else if (e.getKeyCode() == KeyEvent.VK_UP) {
if (currentRow > 0) {
if (tiles[currentRow - 1][currentCol] == 1) { // stepped on white tile
gameOver = true;
} else {
currentRow--;
}
}
} else if (e.getKeyCode() == KeyEvent.VK_DOWN) {
if (currentRow < ROWS - 1) {
if (tiles[currentRow + 1][currentCol] == 1) { // stepped on white tile
gameOver = true;
} else {
currentRow++;
}
}
}
repaint();
if (gameOver) {
removeKeyListener(this);
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
public static void main(String[] args) {
JFrame frame = new JFrame("Don't Tap The White Tile");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
DontTapTheWhiteTile panel = new DontTapTheWhiteTile();
frame.getContentPane().add(panel);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
panel.requestFocusInWindow();
}
}
```
这个游戏的界面是一个4x4的格子,格子上随机摆放着黑色和白色的方块。玩家通过控制一个小人在格子上移动,避免踩到白色方块。玩家可以通过上、下、左、右键控制小人移动。如果小人踩到了白色方块,游戏结束。
这个游戏的实现是一个继承了JPanel的类。在这个类中,我们通过维护一个二维数组来表示格子上的方块,并在paintComponent方法中根据方块的颜色来绘制格子。玩家的移动通过实现KeyListener接口中的方法来响应,如果玩家踩到了白色方块,就设置gameOver标志为true,并从面板上移除KeyListener,停止游戏。
阅读全文
相关推荐
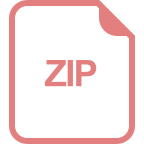
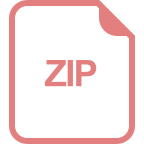
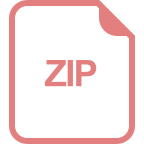
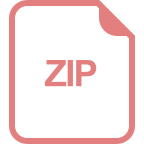
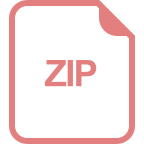