使用stm32f407vet6的spi1接口连接ADS131A04,PA3为drdy引脚,ADS131A04基于标准库函数的驱动软件怎么写?
时间: 2024-02-23 12:59:49 浏览: 172
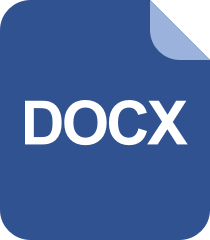
如何编译设备串口通讯SPI驱动程序
首先,需要在stm32f407vet6上配置SPI1接口。以下是基于标准库函数的SPI1配置代码:
```c
// Enable GPIOA clock
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// Enable SPI1 clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1, ENABLE);
// Configure SPI1 pins
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// Connect SPI1 pins to AF5
GPIO_PinAFConfig(GPIOA, GPIO_PinSource5, GPIO_AF_SPI1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource6, GPIO_AF_SPI1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource7, GPIO_AF_SPI1);
// Configure SPI1
SPI_InitTypeDef SPI_InitStruct;
SPI_InitStruct.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStruct.SPI_Mode = SPI_Mode_Master;
SPI_InitStruct.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStruct.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStruct.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStruct.SPI_NSS = SPI_NSS_Soft;
SPI_InitStruct.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_256;
SPI_InitStruct.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStruct.SPI_CRCPolynomial = 7;
SPI_Init(SPI1, &SPI_InitStruct);
// Enable SPI1
SPI_Cmd(SPI1, ENABLE);
```
接下来需要编写ADS131A04的驱动程序。以下是基于标准库函数的ADS131A04驱动程序:
```c
#include "stm32f4xx.h"
#define ADS131A04_CS_LOW() GPIO_ResetBits(GPIOA, GPIO_Pin_4)
#define ADS131A04_CS_HIGH() GPIO_SetBits(GPIOA, GPIO_Pin_4)
void ADS131A04_Init(void)
{
// Enable GPIOA clock
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// Configure ADS131A04 DRDY pin
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_3;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// Configure ADS131A04 CS pin
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_4;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
ADS131A04_CS_HIGH();
}
void ADS131A04_ReadData(uint8_t* data)
{
// Wait for DRDY pin to go low
while(GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_3) == Bit_SET);
// Set CS pin low to select ADS131A04
ADS131A04_CS_LOW();
// Send read command
SPI_I2S_SendData(SPI1, 0x01);
while(SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) == SET);
// Read data
for(int i = 0; i < 8; i++)
{
SPI_I2S_SendData(SPI1, 0x00);
while(SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) == SET);
data[i] = SPI_I2S_ReceiveData(SPI1);
}
// Set CS pin high to deselect ADS131A04
ADS131A04_CS_HIGH();
}
```
在上面的驱动程序中,我们首先初始化了ADS131A04的DRDY和CS引脚。然后,当DRDY引脚变为低电平时,我们将CS引脚设置为低电平,向ADS131A04发送读取命令,并读取8个字节的数据。最后,我们将CS引脚设置为高电平,取消选中ADS131A04。
阅读全文
相关推荐
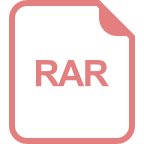
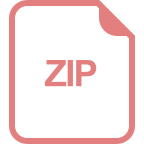

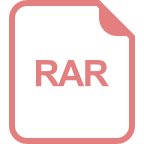
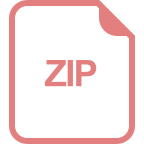
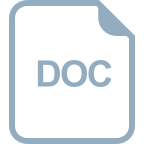
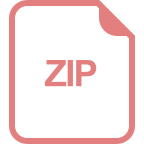
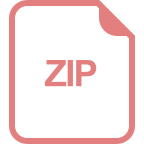
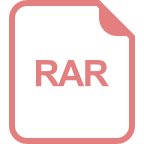
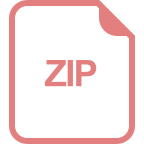
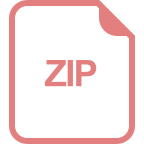
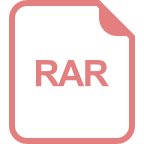
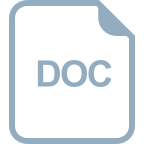
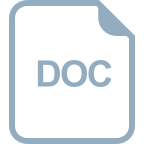


