if (hcan->State == HAL_CAN_STATE_READY) { /* Change CAN peripheral state */ hcan->State = HAL_CAN_STATE_LISTENING; /* Request leave initialisation */ CLEAR_BIT(hcan->Instance->MCR, CAN_MCR_INRQ); /* Get tick */ tickstart = HAL_GetTick(); /* Wait the acknowledge */ while ((hcan->Instance->MSR & CAN_MSR_INAK) != 0U) { /* Check for the Timeout */ if ((HAL_GetTick() - tickstart) > CAN_TIMEOUT_VALUE) { /* Update error code */ hcan->ErrorCode |= HAL_CAN_ERROR_TIMEOUT; /* Change CAN state */ hcan->State = HAL_CAN_STATE_ERROR; return HAL_ERROR; } } /* Reset the CAN ErrorCode */ hcan->ErrorCode = HAL_CAN_ERROR_NONE; /* Return function status */ return HAL_OK; } else { /* Update error code */ hcan->ErrorCode |= HAL_CAN_ERROR_NOT_READY; return HAL_ERROR; 什么作用
时间: 2024-04-04 17:35:20 浏览: 31
这段代码是用于等待CAN总线进入"Listening"状态的函数,函数名可能是`HAL_CAN_WaitForListning()`或者类似的。首先,它检查CAN总线是否处于"Ready"状态,如果不是,则返回错误代码。如果总线已经准备好,则将其状态更改为"Listening",并且请求CAN控制器离开初始化模式。然后等待CAN控制器进入"Active"模式,以便可以开始发送和接收CAN帧。如果在规定时间内CAN控制器未能成功进入"Active"模式,则返回一个超时错误码和错误状态。如果成功进入"Active"模式,则重置错误代码并返回成功状态。
相关问题
基于stm32f103的can数据解析
CAN(Controller Area Network)是一种高速、鲁棒性强的串行通信协议,广泛应用于汽车、工业控制等领域。在STM32F103芯片中,有两个CAN控制器,可以通过CAN总线进行通信。
CAN数据解析过程包括:接收CAN数据、解析CAN数据、处理CAN数据。其中,接收CAN数据是通过CAN总线接收到的数据,解析CAN数据是将CAN数据转换为可读的数据格式,处理CAN数据是根据解析出的数据进行相应的操作。
以下是基于STM32F103的CAN数据解析的步骤:
1. 初始化CAN控制器
首先需要初始化CAN控制器,设置好CAN的时序、波特率等参数。可以使用STM32CubeMX进行配置,也可以手动编写代码进行配置。以下是使用STM32CubeMX进行配置的代码:
```
/* Configure the CAN peripheral */
hcan.Instance = CAN1;
hcan.Init.Prescaler = 5;
hcan.Init.Mode = CAN_MODE_NORMAL;
hcan.Init.SyncJumpWidth = CAN_SJW_1TQ;
hcan.Init.TimeSeg1 = CAN_BS1_13TQ;
hcan.Init.TimeSeg2 = CAN_BS2_2TQ;
hcan.Init.TimeTriggeredMode = DISABLE;
hcan.Init.AutoBusOff = DISABLE;
hcan.Init.AutoWakeUp = DISABLE;
hcan.Init.AutoRetransmission = ENABLE;
hcan.Init.ReceiveFifoLocked = DISABLE;
hcan.Init.TransmitFifoPriority = DISABLE;
if (HAL_CAN_Init(&hcan) != HAL_OK)
{
Error_Handler();
}
/* Configure the CAN filters */
CAN_FilterTypeDef can_filter;
can_filter.FilterMode = CAN_FILTERMODE_IDMASK;
can_filter.FilterScale = CAN_FILTERSCALE_32BIT;
can_filter.FilterIdHigh = 0x0000;
can_filter.FilterIdLow = 0x0000;
can_filter.FilterMaskIdHigh = 0x0000;
can_filter.FilterMaskIdLow = 0x0000;
can_filter.FilterFIFOAssignment = CAN_FILTER_FIFO0;
can_filter.FilterActivation = ENABLE;
can_filter.SlaveStartFilterBank = 14;
if (HAL_CAN_ConfigFilter(&hcan, &can_filter) != HAL_OK)
{
Error_Handler();
}
```
2. 接收CAN数据
在CAN控制器初始化完成后,就可以开始接收CAN数据了。可以使用HAL_CAN_Receive函数进行数据接收。
```
CAN_RxHeaderTypeDef rx_header;
uint8_t rx_data[8];
if (HAL_CAN_GetRxFifoFillLevel(&hcan, CAN_RX_FIFO0) > 0)
{
if (HAL_CAN_Receive(&hcan, CAN_RX_FIFO0, &rx_header, rx_data) == HAL_OK)
{
// 数据接收成功,进行数据解析
}
}
```
3. 解析CAN数据
在接收到CAN数据后,需要对数据进行解析,将CAN数据转换为可读的数据格式。解析CAN数据的方法与具体的CAN协议有关,需要根据实际情况进行调整。
以CANopen协议为例,解析CAN数据的方法如下:
```
uint16_t cob_id;
uint8_t data[8];
uint8_t data_len;
// 解析COB-ID
cob_id = (rx_header.StdId & 0x7FF);
// 解析数据长度
data_len = rx_header.DLC;
// 解析数据
for (int i = 0; i < data_len; i++)
{
data[i] = rx_data[i];
}
// 进行数据处理
// ...
```
4. 处理CAN数据
在解析出CAN数据后,需要根据具体的需求进行相应的处理。处理方式可以是将数据显示在LCD屏幕上、将数据上传到服务器、将数据存储到SD卡中等。
例如,在使用CANopen协议进行通信时,可以根据解析出的数据进行相应的控制操作:
```
uint16_t cob_id;
uint8_t data[8];
uint8_t data_len;
// 解析COB-ID
cob_id = (rx_header.StdId & 0x7FF);
// 解析数据长度
data_len = rx_header.DLC;
// 解析数据
for (int i = 0; i < data_len; i++)
{
data[i] = rx_data[i];
}
// 进行数据处理
switch (cob_id)
{
case 0x200:
// 处理接收到的数据
break;
case 0x201:
// 处理接收到的数据
break;
// ...
default:
break;
}
```
以上就是基于STM32F103的CAN数据解析的步骤。具体的实现方法可以根据实际情况进行修改。
stm32f7777 以太网代码
以下是基于STM32F7777的以太网代码示例:
```c
#include "stm32f7xx_hal.h"
#include "stm32746g_discovery.h"
#include "lwip/opt.h"
#include "lwip/init.h"
#include "lwip/netif.h"
#include "lwip/timeouts.h"
#include "netif/etharp.h"
#include "ethernetif.h"
/* Private variables ---------------------------------------------------------*/
ETH_HandleTypeDef heth;
ETH_TxPacketConfig TxConfig;
/* Private function prototypes -----------------------------------------------*/
void SystemClock_Config(void);
static void MX_GPIO_Init(void);
static void MX_ETH_Init(void);
/* Private functions ---------------------------------------------------------*/
int main(void)
{
/* MCU Configuration--------------------------------------------------------*/
/* Reset of all peripherals, Initializes the Flash interface and the Systick. */
HAL_Init();
/* Configure the system clock */
SystemClock_Config();
/* Initialize all configured peripherals */
MX_GPIO_Init();
MX_ETH_Init();
/* Initilialize the LwIP stack */
lwip_init();
/* Add the network interface (IPv4/IPv6) with default settings */
netif_add(&gnetif, NULL, NULL, NULL, NULL, ðernetif_init, ðernet_input);
/* Registers the default network interface */
netif_set_default(&gnetif);
/* When the netif is fully configured this function must be called */
netif_set_up(&gnetif);
/* Infinite loop */
for (;;)
{
/* Handle LwIP timeouts */
sys_check_timeouts();
/* Poll for received packets and process them */
ethernetif_input(&gnetif);
}
}
/**
* @brief ETH Initialization Function
* @param None
* @retval None
*/
static void MX_ETH_Init(void)
{
/* USER CODE BEGIN ETH_Init 0 */
/* USER CODE END ETH_Init 0 */
/* USER CODE BEGIN ETH_Init 1 */
/* USER CODE END ETH_Init 1 */
heth.Instance = ETH;
heth.Init.AutoNegotiation = ETH_AUTONEGOTIATION_ENABLE;
heth.Init.PhyAddress = LAN8742A_PHY_ADDRESS;
heth.Init.MACAddr[0] = 0x00;
heth.Init.MACAddr[1] = 0x80;
heth.Init.MACAddr[2] = 0xE1;
heth.Init.MACAddr[3] = 0x00;
heth.Init.MACAddr[4] = 0x00;
heth.Init.MACAddr[5] = 0x00;
heth.Init.RxMode = ETH_RXINTERRUPT_MODE;
heth.Init.ChecksumMode = ETH_CHECKSUM_BY_HARDWARE;
heth.Init.MediaInterface = ETH_MEDIA_INTERFACE_RMII;
/* USER CODE BEGIN MACADDRESS */
/* USER CODE END MACADDRESS */
if (HAL_ETH_Init(&heth) != HAL_OK)
{
Error_Handler();
}
/* USER CODE BEGIN ETH_Init 2 */
/* USER CODE END ETH_Init 2 */
}
/**
* @brief System Clock Configuration
* @retval None
*/
void SystemClock_Config(void)
{
RCC_OscInitTypeDef RCC_OscInitStruct = {0};
RCC_ClkInitTypeDef RCC_ClkInitStruct = {0};
/** Initializes the RCC Oscillators according to the specified parameters
* in the RCC_OscInitTypeDef structure.
*/
RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSE;
RCC_OscInitStruct.HSEState = RCC_HSE_ON;
RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON;
RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSE;
RCC_OscInitStruct.PLL.PLLM = 25;
RCC_OscInitStruct.PLL.PLLN = 400;
RCC_OscInitStruct.PLL.PLLP = RCC_PLLP_DIV2;
RCC_OscInitStruct.PLL.PLLQ = 8;
if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK)
{
Error_Handler();
}
/** Initializes the CPU, AHB and APB buses clocks
*/
RCC_ClkInitStruct.ClockType = RCC_CLOCKTYPE_HCLK|RCC_CLOCKTYPE_SYSCLK
|RCC_CLOCKTYPE_PCLK1|RCC_CLOCKTYPE_PCLK2;
RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK;
RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1;
RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV4;
RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV2;
if (HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_6) != HAL_OK)
{
Error_Handler();
}
}
/**
* @brief GPIO Initialization Function
* @param None
* @retval None
*/
static void MX_GPIO_Init(void)
{
/* GPIO Ports Clock Enable */
__HAL_RCC_GPIOJ_CLK_ENABLE();
}
/* Relevant functions for Ethernet driver */
void HAL_ETH_MspInit(ETH_HandleTypeDef* ethHandle)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
if(ethHandle->Instance==ETH)
{
/* USER CODE BEGIN ETH_MspInit 0 */
/* USER CODE END ETH_MspInit 0 */
/* Peripheral clock enable */
__HAL_RCC_ETH_CLK_ENABLE();
__HAL_RCC_GPIOA_CLK_ENABLE();
__HAL_RCC_GPIOC_CLK_ENABLE();
__HAL_RCC_GPIOG_CLK_ENABLE();
/**ETH GPIO Configuration
PA1 ------> ETH_REF_CLK
PA2 ------> ETH_MDIO
PA7 ------> ETH_CRS_DV
PC1 ------> ETH_MDC
PC4 ------> ETH_RXD0
PC5 ------> ETH_RXD1
PG11 ------> ETH_TX_EN
PG13 ------> ETH_TXD0
PG14 ------> ETH_TXD1
*/
GPIO_InitStruct.Pin = GPIO_PIN_1|GPIO_PIN_2|GPIO_PIN_7;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF11_ETH;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.Pin = GPIO_PIN_1|GPIO_PIN_4|GPIO_PIN_5;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF11_ETH;
HAL_GPIO_Init(GPIOC, &GPIO_InitStruct);
GPIO_InitStruct.Pin = GPIO_PIN_11|GPIO_PIN_13|GPIO_PIN_14;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF11_ETH;
HAL_GPIO_Init(GPIOG, &GPIO_InitStruct);
/* USER CODE BEGIN ETH_MspInit 1 */
/* USER CODE END ETH_MspInit 1 */
}
}
void HAL_ETH_MspDeInit(ETH_HandleTypeDef* ethHandle)
{
if(ethHandle->Instance==ETH)
{
/* USER CODE BEGIN ETH_MspDeInit 0 */
/* USER CODE END ETH_MspDeInit 0 */
/* Peripheral clock disable */
__HAL_RCC_ETH_CLK_DISABLE();
/**ETH GPIO Configuration
PA1 ------> ETH_REF_CLK
PA2 ------> ETH_MDIO
PA7 ------> ETH_CRS_DV
PC1 ------> ETH_MDC
PC4 ------> ETH_RXD0
PC5 ------> ETH_RXD1
PG11 ------> ETH_TX_EN
PG13 ------> ETH_TXD0
PG14 ------> ETH_TXD1
*/
HAL_GPIO_DeInit(GPIOA, GPIO_PIN_1|GPIO_PIN_2|GPIO_PIN_7);
HAL_GPIO_DeInit(GPIOC, GPIO_PIN_1|GPIO_PIN_4|GPIO_PIN_5);
HAL_GPIO_DeInit(GPIOG, GPIO_PIN_11|GPIO_PIN_13|GPIO_PIN_14);
/* USER CODE BEGIN ETH_MspDeInit 1 */
/* USER CODE END ETH_MspDeInit 1 */
}
}
/**
* @brief Retargets the C library printf function to the USART.
* @param None
* @retval None
*/
PUTCHAR_PROTOTYPE
{
/* Place your implementation of fputc here */
/* e.g. write a character to the USART */
HAL_UART_Transmit(&huart3, (uint8_t *)&ch, 1, HAL_MAX_DELAY);
return ch;
}
/* Relevant functions for LwIP stack */
void ethernetif_input(struct netif *netif)
{
err_t err;
struct pbuf *p;
/* move received packet into a new pbuf */
err = ethernetif_recv(netif, &p);
/* if no error occured, send packet */
if (err == ERR_OK)
{
/* pass all packets to upper layer */
netif->input(p, netif);
}
else
{
/* Free buffer */
pbuf_free(p);
}
}
/* Relevant functions for LwIP stack */
void HAL_ETH_RxCpltCallback(ETH_HandleTypeDef *heth)
{
/* Frame received */
ethernetif_input(&gnetif);
/* Clear the Eth DMA Rx IT pending bits */
__HAL_ETH_DMA_CLEAR_IT(heth, ETH_DMA_IT_R);
/* Resume DMA reception */
HAL_ETH_Receive_IT(heth);
}
/* Relevant functions for LwIP stack */
void HAL_ETH_TxCpltCallback(ETH_HandleTypeDef *heth)
{
/* Clear the Eth DMA Tx IT pending bits */
__HAL_ETH_DMA_CLEAR_IT(heth, ETH_DMA_IT_T);
/* Process Unlocked */
osSemaphoreRelease(s_xSemaphore);
}
/* Relevant functions for LwIP stack */
void HAL_ETH_ErrorCallback(ETH_HandleTypeDef *heth)
{
/* Stop the transmission process */
HAL_ETH_TransmitStop(&heth);
/* Flush Transmit FIFO */
HAL_ETH_FlushTransmitFIFO(&heth);
/* Resume DMA reception */
HAL_ETH_Receive_IT(&heth);
}
/* Relevant functions for LwIP stack */
void HAL_ETH_MspInit(ETH_HandleTypeDef* ethHandle)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
if(ethHandle->Instance==ETH)
{
/* USER CODE BEGIN ETH_MspInit 0 */
/* USER CODE END ETH_MspInit 0 */
/* Peripheral clock enable */
__HAL_RCC_ETH_CLK_ENABLE();
__HAL_RCC_GPIOA_CLK_ENABLE();
__HAL_RCC_GPIOC_CLK_ENABLE();
__HAL_RCC_GPIOG_CLK_ENABLE();
/**ETH GPIO Configuration
PA1 ------> ETH_REF_CLK
PA2 ------> ETH_MDIO
PA7 ------> ETH_CRS_DV
PC1 ------> ETH_MDC
PC4 ------> ETH_RXD0
PC5 ------> ETH_RXD1
PG11 ------> ETH_TX_EN
PG13 ------> ETH_TXD0
PG14 ------> ETH_TXD1
*/
GPIO_InitStruct.Pin = GPIO_PIN_1|GPIO_PIN_2|GPIO_PIN_7;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF11_ETH;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.Pin = GPIO_PIN_1|GPIO_PIN_4|GPIO_PIN_5;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF11_ETH;
HAL_GPIO_Init(GPIOC, &GPIO_InitStruct);
GPIO_InitStruct.Pin = GPIO_PIN_11|GPIO_PIN_13|GPIO_PIN_14;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF11_ETH;
HAL_GPIO_Init(GPIOG, &GPIO_InitStruct);
/* USER CODE BEGIN ETH_MspInit 1 */
/* USER CODE END ETH_MspInit 1 */
}
}
/* Relevant functions for LwIP stack */
void HAL_ETH_MspDeInit(ETH_HandleTypeDef* ethHandle)
{
if(ethHandle->Instance==ETH)
{
/* USER CODE BEGIN ETH_MspDeInit 0 */
/* USER CODE END ETH_MspDeInit 0 */
/* Peripheral clock disable */
__HAL_RCC_ETH_CLK_DISABLE();
/**ETH GPIO Configuration
PA1 ------> ETH_REF_CLK
PA2 ------> ETH_MDIO
PA7 ------> ETH_CRS_DV
PC1 ------> ETH_MDC
PC4 ------> ETH_RXD0
PC5 ------> ETH_RXD1
PG11 ------> ETH_TX_EN
PG13 ------> ETH_TXD0
PG14 ------> ETH_TXD1
*/
HAL_GPIO_DeInit(GPIOA, GPIO_PIN_1|GPIO_PIN_2|GPIO_PIN_7);
HAL_GPIO_DeInit(GPIOC, GPIO_PIN_1|GPIO_PIN_4|GPIO_PIN_5);
HAL_GPIO_DeInit(GPIOG, GPIO_PIN_11|GPIO_PIN_13|GPIO_PIN_14);
/* USER CODE BEGIN ETH_MspDeInit 1 */
/* USER CODE END ETH_MspDeInit 1 */
}
}
/* Relevant functions for LwIP stack */
void HAL_TIM_PeriodElapsedCallback(TIM_HandleTypeDef *htim)
{
/* Handle timeouts */
sys_check_timeouts();
}
/* Relevant functions for LwIP stack */
void Error_Handler(void)
{
/* User can add his own implementation to report the HAL error return state */
while(1)
{
}
}
/* Relevant functions for LwIP stack */
void assert_failed(uint8_t* file, uint32_t line)
{
/* User can add his own implementation to report the file name and line number,
ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */
/* Infinite loop */
while (1)
{
}
}
```
相关推荐






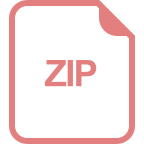
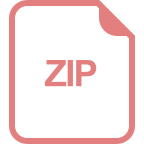