linux itoa()
时间: 2023-06-17 22:07:08 浏览: 60
在 Linux 中,itoa() 函数通常不是标准库函数,因此需要自行实现。
下面是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
char *itoa(int num, char *str, int base)
{
int i = 0, isNegative = 0;
/* Handle 0 explicitly */
if (num == 0) {
str[i++] = '0';
str[i] = '\0';
return str;
}
/* Handle negative numbers */
if (num < 0 && base == 10) {
isNegative = 1;
num = -num;
}
/* Process individual digits */
while (num != 0) {
int rem = num % base;
str[i++] = (rem > 9) ? (rem - 10) + 'a' : rem + '0';
num /= base;
}
/* If number is negative, append '-' */
if (isNegative)
str[i++] = '-';
str[i] = '\0'; /* Append string terminator */
/* Reverse the string */
int j, len = i;
for (i = 0, j = len-1; i < j; i++, j--) {
char temp = str[i];
str[i] = str[j];
str[j] = temp;
}
return str;
}
```
使用示例:
```c
int main()
{
int num = -123;
char str[12];
itoa(num, str, 10);
printf("%s\n", str);
return 0;
}
```
输出:`-123`
相关推荐
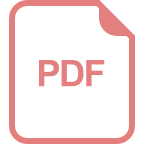









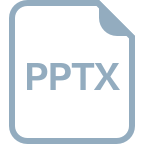