Linux itoa()
时间: 2024-09-02 08:02:50 浏览: 50
Linux中的itoa()函数是一个将整数转换成字符串的库函数,全称为integer to ASCII string,主要用于处理数字到字符序列的转换。它通常在头文件`<stdlib.h>`或`<string.h>`中定义,例如`itoa(value, buffer, base)`。这个函数接收三个参数:
1. `value`: 需要转换的整数值。
2. `buffer`: 存放转换结果的目标字符数组。
3. `base`: 可选,指定进制,默认为10(十进制)。其他值如2表示二进制,8表示八进制,16表示十六进制。
itoa()函数会尝试将`value`转换为指定进制的字符串,并存储在`buffer`中。如果转换成功,返回的是`buffer`的指针;如果转换失败(如`value`过大导致溢出),则通常会设置`buffer`的第一个元素为负数以表明错误状态,并返回NULL。
相关问题
linux itoa()
在 Linux 中,itoa() 函数通常不是标准库函数,因此需要自行实现。
下面是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
char *itoa(int num, char *str, int base)
{
int i = 0, isNegative = 0;
/* Handle 0 explicitly */
if (num == 0) {
str[i++] = '0';
str[i] = '\0';
return str;
}
/* Handle negative numbers */
if (num < 0 && base == 10) {
isNegative = 1;
num = -num;
}
/* Process individual digits */
while (num != 0) {
int rem = num % base;
str[i++] = (rem > 9) ? (rem - 10) + 'a' : rem + '0';
num /= base;
}
/* If number is negative, append '-' */
if (isNegative)
str[i++] = '-';
str[i] = '\0'; /* Append string terminator */
/* Reverse the string */
int j, len = i;
for (i = 0, j = len-1; i < j; i++, j--) {
char temp = str[i];
str[i] = str[j];
str[j] = temp;
}
return str;
}
```
使用示例:
```c
int main()
{
int num = -123;
char str[12];
itoa(num, str, 10);
printf("%s\n", str);
return 0;
}
```
输出:`-123`
linux的itoa
在Linux中,itoa函数是一个将整数转换为字符串的函数。然而,需要注意的是,itoa函数并不是标准C库中的函数,而是一种非标准的实现。在Linux中,通常使用sprintf函数来实现整数到字符串的转换。
sprintf函数是一个格式化输出函数,可以将不同类型的数据转换为字符串。它的原型如下:
```c
int sprintf(char *str, const char *format, ...);
```
其中,str是一个字符数组,用于存储转换后的字符串;format是一个格式化字符串,用于指定输出的格式;...表示可变参数,用于指定要转换的数据。
要将整数转换为字符串,可以使用以下代码:
```c
int num = 123;
char str[10];
sprintf(str, "%d", num);
```
在上述代码中,将整数num转换为字符串,并存储在字符数组str中。"%d"是格式化字符串,用于指定整数的输出格式。
需要注意的是,sprintf函数存在安全性问题,如果不正确地使用格式化字符串,可能会导致缓冲区溢出等问题。因此,在实际开发中,建议使用更安全的函数,如snprintf函数。
希望以上信息能够帮助到你!
阅读全文
相关推荐
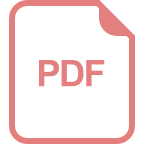
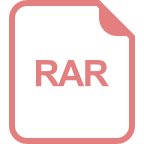
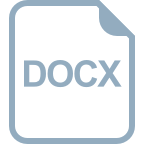














