Linux设计一个程序实现以下功能: 父进程创建两个子进程,父子进程间通过消息队列实现进程通信。父进程发送10条信息,分别为message1、messsage2……message10,子进程1从消息队列上读取奇数次消息,即message1、messsage3……message9,子进程2从消息队列上读取偶数次消息,即message2、messsage4……message10。 提示:可以使用不同的type将奇偶次消息区分。
时间: 2024-02-13 18:04:03 浏览: 69
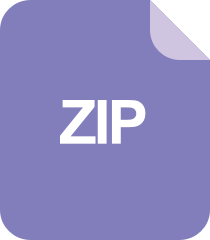
利用消息队列实现进程间通信
以下是一个实现上述功能的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 100
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main()
{
key_t key;
int msgid;
int i;
pid_t pid1, pid2;
struct msgbuf msg;
// 创建消息队列
key = ftok(".", 'm');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 创建子进程1
pid1 = fork();
if (pid1 == -1) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid1 == 0) { // 子进程1
for (i = 1; i <= 10; i += 2) {
msgrcv(msgid, &msg, MSG_SIZE, i, 0);
printf("Child process 1 received message: %s\n", msg.mtext);
}
exit(EXIT_SUCCESS);
}
// 创建子进程2
pid2 = fork();
if (pid2 == -1) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid2 == 0) { // 子进程2
for (i = 2; i <= 10; i += 2) {
msgrcv(msgid, &msg, MSG_SIZE, i, 0);
printf("Child process 2 received message: %s\n", msg.mtext);
}
exit(EXIT_SUCCESS);
}
// 父进程发送消息
for (i = 1; i <= 10; i++) {
strcpy(msg.mtext, "message");
strcat(msg.mtext, itoa(i, 10));
msg.mtype = i;
msgsnd(msgid, &msg, MSG_SIZE, 0);
}
// 等待子进程结束
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
// 删除消息队列
msgctl(msgid, IPC_RMID, NULL);
return 0;
}
```
在上述代码中,我们使用了系统调用 `msgget` 创建一个消息队列,并使用 `msgrcv` 和 `msgsnd` 分别实现了进程间的通信。父进程通过 `msgsnd` 发送了10条消息,并通过不同的 `mtype` 区分了奇偶数消息。子进程1和子进程2 分别通过 `msgrcv` 从消息队列上读取奇数次消息和偶数次消息,然后输出到控制台上。
需要注意的是,在使用 `ftok` 创建 `key` 时,我们指定了当前目录 (".") 作为路径,'m' 作为标识符,这样可以确保在不同的进程中使用相同的路径和标识符创建消息队列。
阅读全文
相关推荐
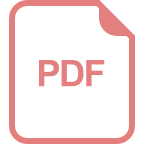
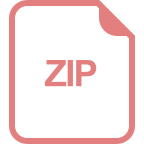
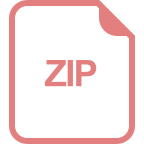
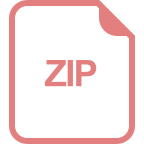
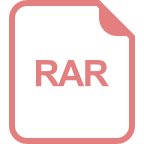
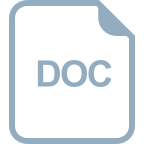
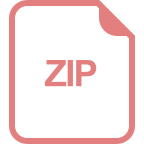
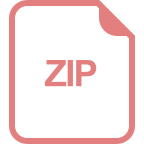
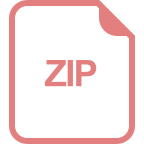