编写C语言程序,创建子进程并实现父子进程间的通信。
时间: 2024-04-29 10:26:21 浏览: 328
可以使用fork()函数创建子进程,使用pipe()函数或者共享内存实现父子进程间的通信。下面是一个简单的示例程序:
```
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int fd[2];
pid_t pid;
if (pipe(fd) == -1) {
perror("pipe");
exit(1);
}
// 创建子进程
pid = fork();
if (pid < 0) {
perror("fork");
exit(1);
} else if (pid == 0) {
// 子进程读取父进程发送的消息
char buf[1024];
close(fd[1]);
while (read(fd[0], buf, sizeof(buf)) > 0) {
printf("[Child] Received message: %s", buf);
}
close(fd[0]);
printf("[Child] Exit.\n");
exit(0);
} else {
// 父进程发送消息给子进程
char *msg = "Hello, child!\n";
close(fd[0]);
write(fd[1], msg, strlen(msg));
close(fd[1]);
printf("[Parent] Sent message: %s", msg);
}
return 0;
}
```
该程序通过pipe()函数创建了一个管道fd,使用fork()函数创建了一个子进程pid。父进程使用write()函数向管道写入消息,子进程使用read()函数从管道读取并输出消息。注意要先关闭管道中未使用的一端(子进程关闭fd[1],父进程关闭fd[0])。
阅读全文
相关推荐
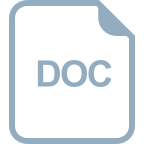
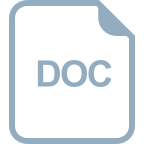
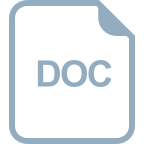



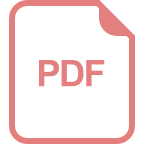
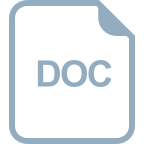
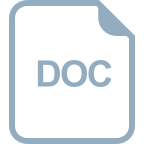
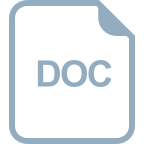
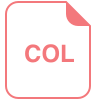
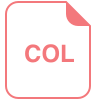






