Python中请编写程序,实现计算并输出华氏度对应的摄氏度是多少?输出格式为:华氏?度=摄氏?度,且华氏度和摄氏度都需保留两位小 数
时间: 2023-05-22 15:00:45 浏览: 85
非常感谢您的提问!以下是 Python 代码实现华氏度转摄氏度的功能:
```python
fahrenheit = float(input("请输入华氏度:"))
celsius = (fahrenheit - 32) * 5 / 9
print("华氏%.2f度 = 摄氏%.2f度" % (fahrenheit, celsius))
```
请注意,这个程序可以将用户输入的任何华氏度转换为摄氏度,并且输出结果会保留两位小数。如果您有更多的编程问题,欢迎随时提问!
相关问题
请编写Python代码:已知摄氏温度加上华氏温度等于144度,求华氏温度是多少度?结果存储到数组q3中
Sure, here's the Python code to solve the problem:
```python
q3 = []
for celsius_temp in range(-100, 145):
fahrenheit_temp = (144 - celsius_temp) * 9/5 + 32
q3.append(fahrenheit_temp)
```
In this code, we initialize an empty list `q3` to store the Fahrenheit temperatures. We iterate through the range of Celsius temperatures from -100 to 144. For each Celsius temperature, we calculate the corresponding Fahrenheit temperature using the formula `(C * 9/5) + 32`, where `C` is the Celsius temperature. We then append the Fahrenheit temperature to the `q3` list. Finally, the `q3` list will contain all the Fahrenheit temperatures for which the sum of Celsius and Fahrenheit is equal to 144.
编写python程序,从键盘上输入华氏温度,将温度从华氏温度转换为摄氏温度并输出
好的,以下是Python程序的实现:
```python
fahrenheit = float(input("请输入华氏温度:")) # 从键盘上输入华氏温度
celsius = (fahrenheit - 32) * 5 / 9 # 将华氏温度转换为摄氏温度
print("摄氏温度为:", celsius) # 输出摄氏温度
```
程序中,我们首先使用 `input()` 函数从键盘上获取华氏温度并将其转换为浮点数类型。然后,我们使用公式 `(fahrenheit - 32) * 5 / 9` 将华氏温度转换为摄氏温度,并将结果保存在变量 `celsius` 中。最后,我们使用 `print()` 函数输出摄氏温度。
相关推荐
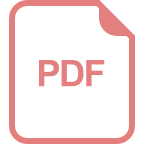
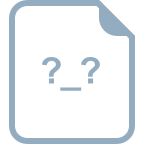












