485轮询主从通讯c语言代码
时间: 2023-08-31 11:27:34 浏览: 248
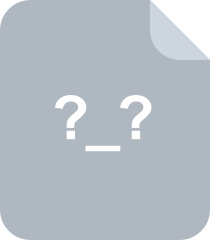
485通信源码
### 回答1:
轮询主从通讯是指在主从通信中,主设备通过不断地轮询从设备的状态,以实现数据传输的一种通信方式。下面是一个基本的C语言示例代码,用于实现轮询主从通信:
主设备代码:
```c
#include <stdio.h>
#include <stdbool.h>
#define SLAVE_READY 0x01 // 从设备准备好的状态码
int main() {
bool slave_ready = false;
while (!slave_ready) {
// 轮询从设备状态
// 假设通过I2C总线通信
int slave_status = i2c_read_status();
if (slave_status == SLAVE_READY) {
slave_ready = true;
printf("Slave device is ready.\n");
}
}
// 向从设备发送数据
// 假设通过I2C总线通信
i2c_send_data();
return 0;
}
```
从设备代码:
```c
#include <stdio.h>
#define SLAVE_READY 0x01 // 从设备准备好的状态码
int main() {
// 从设备准备好后发送状态码
// 假设通过I2C总线通信
i2c_send_status(SLAVE_READY);
// 接收主设备发送的数据
// 假设通过I2C总线通信
i2c_receive_data();
return 0;
}
```
需要注意的是,上述示例代码只是一个简单的示例,实际情况可能会更加复杂。具体实现方式还需根据具体的通信协议和硬件设备进行调整。
### 回答2:
485轮询主从通讯是一种在嵌入式系统中常见的通讯方式,它适用于主设备(主机)和从设备(从机)之间的通讯。下面是一个基于C语言的485轮询主从通讯代码示例:
```
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int fd;
struct termios serial_config;
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("无法打开串口设备");
return -1;
}
// 配置串口
tcgetattr(fd, &serial_config);
cfsetispeed(&serial_config, B9600); // 设置输入波特率为9600
cfsetospeed(&serial_config, B9600); // 设置输出波特率为9600
serial_config.c_cflag |= (CLOCAL | CREAD); // 启用接收和本地模式
serial_config.c_cflag &= ~PARENB; // 禁用校验位
serial_config.c_cflag &= ~CSTOPB; // 设置停止位为1
serial_config.c_cflag &= ~CSIZE; // 清除原有字节大小
serial_config.c_cflag |= CS8; // 设置字节大小为8位
tcsetattr(fd, TCSANOW, &serial_config);
// 读取和写入数据
unsigned char send_data = 0x01;
unsigned char receive_data;
while (1) {
// 发送数据
write(fd, &send_data, 1);
// 接收数据
read(fd, &receive_data, 1);
// 处理接收到的数据
printf("接收到的数据:%d\n", receive_data);
// 等待适当时间
usleep(1000);
}
// 关闭串口设备
close(fd);
return 0;
}
```
以上的代码是一个简单的485轮询主从通讯的示例。它首先打开串口设备`/dev/ttyS0`,然后配置串口的波特率、校验位、停止位和字节大小等参数。接下来,在一个无限循环中,它将一个字节的数据发送给从设备,然后接收从设备返回的数据,并处理接收到的数据。最后,它等待一段时间后再次发送数据,进入下一轮的轮询。在实际使用中,你需要根据具体的硬件和通讯协议进行调整和优化。
### 回答3:
485轮询主从通讯是一种基于C语言的主从通信方式,其代码示例如下:
主节点(Master Node)代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/i2c.h>
#include <linux/i2c-dev.h>
#define SLAVE_ADDRESS 0x20
int main() {
int file;
char buffer[1];
char data[4];
file = open("/dev/i2c-1", O_RDWR); // 打开I2C文件
if(file < 0) {
perror("Failed to open the I2C device");
return -1;
}
if(ioctl(file, I2C_SLAVE, SLAVE_ADDRESS) < 0) {
perror("Failed to acquire bus access and/or talk to slave");
return -1;
}
while(1) {
// 从从节点读取数据
if(read(file, buffer, sizeof(buffer)) != sizeof(buffer)) {
perror("Failed to read from slave");
return -1;
}
// 处理数据
// ...
// 向从节点发送数据
if(write(file, data, sizeof(data)) != sizeof(data)) {
perror("Failed to write to slave");
return -1;
}
usleep(500000); // 500ms延时
}
close(file);
return 0;
}
```
从节点(Slave Node)代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/i2c.h>
#include <linux/i2c-dev.h>
#define SLAVE_ADDRESS 0x20
int main() {
int file;
char buffer[1];
char data[4];
file = open("/dev/i2c-1", O_RDWR); // 打开I2C文件
if(file < 0) {
perror("Failed to open the I2C device");
return -1;
}
if(ioctl(file, I2C_SLAVE, SLAVE_ADDRESS) < 0) {
perror("Failed to acquire bus access and/or talk to slave");
return -1;
}
while(1) {
// 从主节点读取数据
if(read(file, buffer, sizeof(buffer)) != sizeof(buffer)) {
perror("Failed to read from master");
return -1;
}
// 处理数据
// ...
// 向主节点发送数据
if(write(file, data, sizeof(data)) != sizeof(data)) {
perror("Failed to write to master");
return -1;
}
usleep(500000); // 500ms延时
}
close(file);
return 0;
}
```
以上是基于C语言的485轮询主从通讯的代码示例。主节点通过打开I2C设备文件并设置从节点地址进行通信,循环中首先从从节点读取数据,处理数据后再向从节点发送数据。从节点的操作与主节点类似,只不过读写的对象倒置。通过循环和延时,可以实现主从节点之间的轮询通讯。请注意,以上代码仅为示例,实际应用中需根据具体使用环境和需求进行修改。
阅读全文
相关推荐

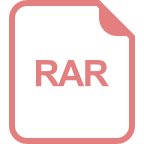
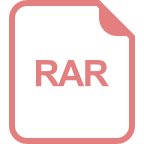
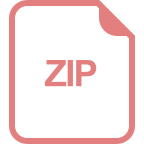
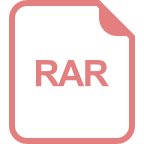
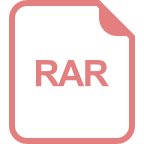
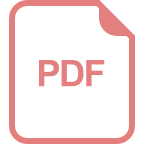
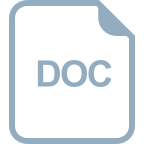
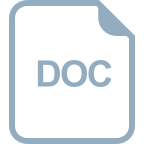
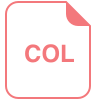

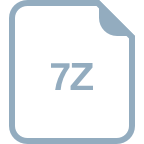
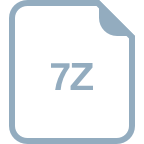