制作一个天气预报APP,要求 1.界面根据自己爱好布局,美观大方。 2.天气信息从“http://t.weather.sojson.com/api/weather/city/101180101”获得,最后的数字为城市编码。 3.主页面中有三个单选按钮(分别显示自己喜欢的三个城市名称)。 4.单击按钮,将城市编码作为参数传递到第二个界面,在第二个界面中显示所选城市的三天内的天气信息,每天至少显示日期、最高气温、最低气温和天气类型等信息。 5.获取网络数据在子线程中完成。用代码表示出来
时间: 2023-06-16 15:07:03 浏览: 58
以下是一个简单的天气预报APP的代码示例,具体实现可能需要根据需求进行修改:
MainActivity.java
```java
public class MainActivity extends AppCompatActivity {
private RadioGroup cityRadioGroup;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
cityRadioGroup = findViewById(R.id.city_radio_group);
Button checkWeatherButton = findViewById(R.id.check_weather_button);
checkWeatherButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int cityCode = getSelectedCityCode();
Intent intent = new Intent(MainActivity.this, WeatherActivity.class);
intent.putExtra("city_code", cityCode);
startActivity(intent);
}
});
}
private int getSelectedCityCode() {
switch (cityRadioGroup.getCheckedRadioButtonId()) {
case R.id.beijing_radio_button:
return 101010100;
case R.id.shanghai_radio_button:
return 101020100;
case R.id.guangzhou_radio_button:
return 101280101;
default:
return 101010100; // 默认为北京
}
}
}
```
activity_main.xml
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<RadioGroup
android:id="@+id/city_radio_group"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<RadioButton
android:id="@+id/beijing_radio_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="北京" />
<RadioButton
android:id="@+id/shanghai_radio_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="上海" />
<RadioButton
android:id="@+id/guangzhou_radio_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="广州" />
</RadioGroup>
<Button
android:id="@+id/check_weather_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="查看天气" />
</LinearLayout>
```
WeatherActivity.java
```java
public class WeatherActivity extends AppCompatActivity {
private TextView cityNameTextView;
private TextView date1TextView;
private TextView date2TextView;
private TextView date3TextView;
private TextView high1TextView;
private TextView high2TextView;
private TextView high3TextView;
private TextView low1TextView;
private TextView low2TextView;
private TextView low3TextView;
private TextView type1TextView;
private TextView type2TextView;
private TextView type3TextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_weather);
cityNameTextView = findViewById(R.id.city_name_text_view);
date1TextView = findViewById(R.id.date1_text_view);
date2TextView = findViewById(R.id.date2_text_view);
date3TextView = findViewById(R.id.date3_text_view);
high1TextView = findViewById(R.id.high1_text_view);
high2TextView = findViewById(R.id.high2_text_view);
high3TextView = findViewById(R.id.high3_text_view);
low1TextView = findViewById(R.id.low1_text_view);
low2TextView = findViewById(R.id.low2_text_view);
low3TextView = findViewById(R.id.low3_text_view);
type1TextView = findViewById(R.id.type1_text_view);
type2TextView = findViewById(R.id.type2_text_view);
type3TextView = findViewById(R.id.type3_text_view);
int cityCode = getIntent().getIntExtra("city_code", 101010100); // 默认为北京
new WeatherTask().execute(cityCode);
}
private class WeatherTask extends AsyncTask<Integer, Void, String> {
@Override
protected String doInBackground(Integer... params) {
int cityCode = params[0];
String url = "http://t.weather.sojson.com/api/weather/city/" + cityCode;
try {
URLConnection connection = new URL(url).openConnection();
InputStream inputStream = connection.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder result = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
result.append(line);
}
return result.toString();
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
@Override
protected void onPostExecute(String result) {
if (result != null) {
try {
JSONObject json = new JSONObject(result);
JSONObject city = json.getJSONObject("cityInfo");
String cityName = city.getString("city");
cityNameTextView.setText(cityName);
JSONArray forecast = json.getJSONArray("data");
if (forecast.length() >= 3) {
JSONObject day1 = forecast.getJSONObject(0);
JSONObject day2 = forecast.getJSONObject(1);
JSONObject day3 = forecast.getJSONObject(2);
date1TextView.setText(day1.getString("date"));
date2TextView.setText(day2.getString("date"));
date3TextView.setText(day3.getString("date"));
high1TextView.setText(day1.getString("high"));
high2TextView.setText(day2.getString("high"));
high3TextView.setText(day3.getString("high"));
low1TextView.setText(day1.getString("low"));
low2TextView.setText(day2.getString("low"));
low3TextView.setText(day3.getString("low"));
type1TextView.setText(day1.getString("type"));
type2TextView.setText(day2.getString("type"));
type3TextView.setText(day3.getString("type"));
}
} catch (JSONException e) {
e.printStackTrace();
}
}
}
}
}
```
activity_weather.xml
```xml
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/city_name_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:textSize="24sp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:paddingTop="16dp"
android:paddingBottom="16dp">
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="日期" />
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="最高气温" />
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="最低气温" />
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="天气类型" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:paddingTop="8dp"
android:paddingBottom="8dp">
<TextView
android:id="@+id/date1_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/high1_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/low1_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/type1_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:paddingTop="8dp"
android:paddingBottom="8dp">
<TextView
android:id="@+id/date2_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/high2_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/low2_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/type2_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:paddingTop="8dp"
android:paddingBottom="8dp">
<TextView
android:id="@+id/date3_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/high3_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/low3_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/type3_text_view"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
</LinearLayout>
</LinearLayout>
</ScrollView>
```
相关推荐
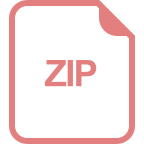
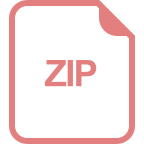
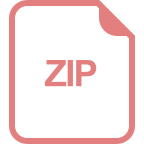















