怎样用android制作一个天气预报APP及代码实现。要求1.界面根据自己爱好布局,美观大方。 2.天气信息从“http://t.weather.sojson.com/api/weather/city/101180101”获得,最后的数字为城市编码。 3.主页面中有三个单选按钮(分别显示自己喜欢的三个城市名称)。 4.单击按钮,将城市编码作为参数传递到第二个界面,在第二个界面中显示所选城市的三天内的天气信息,每天至少显示日期、最高气温、最低气温和天气类型等信息。 5.获取网络数据在子线程中完成。
时间: 2023-06-14 08:07:35 浏览: 192
制作一个天气预报APP需要使用Android Studio进行开发,以下是实现步骤:
1. 创建Android Studio项目,选择Empty Activity模板。
2. 在项目的build.gradle文件中添加以下依赖:
```
implementation 'com.squareup.okhttp3:okhttp:4.9.0'
implementation 'com.google.code.gson:gson:2.8.6'
```
3. 在布局文件中添加三个单选按钮,一个Button按钮,一个TextView控件和一个RecyclerView控件。
4. 使用OkHttp库进行网络请求,获取天气信息并解析JSON数据。以下是获取天气信息的代码:
```java
private void getWeatherInfo(String cityCode) {
String url = "http://t.weather.sojson.com/api/weather/city/" + cityCode;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(url).build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
e.printStackTrace();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
if (response.isSuccessful()) {
String json = response.body().string();
Gson gson = new Gson();
WeatherInfo weatherInfo = gson.fromJson(json, WeatherInfo.class);
runOnUiThread(() -> {
showWeatherInfo(weatherInfo);
});
}
}
});
}
```
5. 在第一个界面中,根据用户的选择,传递对应的城市编码到第二个界面。
```java
button.setOnClickListener(view -> {
String cityCode = "";
if (radioButton1.isChecked()) {
cityCode = "101180101";
} else if (radioButton2.isChecked()) {
cityCode = "101200101";
} else if (radioButton3.isChecked()) {
cityCode = "101010100";
}
Intent intent = new Intent(MainActivity.this, WeatherActivity.class);
intent.putExtra("cityCode", cityCode);
startActivity(intent);
});
```
6. 在第二个界面中,根据接收到的城市编码,显示该城市未来三天的天气信息。以下是显示天气信息的代码:
```java
private void showWeatherInfo(WeatherInfo weatherInfo) {
List<Forecast> forecastList = weatherInfo.getData().getForecast();
WeatherAdapter adapter = new WeatherAdapter(forecastList);
recyclerView.setAdapter(adapter);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
String cityName = weatherInfo.getCityInfo().getCity();
String updateTime = weatherInfo.getTime();
String info = String.format("%s 更新时间:%s", cityName, updateTime);
textView.setText(info);
}
```
7. 在RecyclerView中显示天气信息,可以使用一个自定义的Adapter,并在Adapter中实现ViewHolder。以下是Adapter的代码:
```java
public class WeatherAdapter extends RecyclerView.Adapter<WeatherAdapter.ViewHolder> {
private List<Forecast> forecastList;
public WeatherAdapter(List<Forecast> forecastList) {
this.forecastList = forecastList;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_weather, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
Forecast forecast = forecastList.get(position);
String date = forecast.getDate();
String high = forecast.getHigh();
String low = forecast.getLow();
String type = forecast.getType();
String info = String.format("%s %s ~ %s %s", date, high, low, type);
holder.textView.setText(info);
}
@Override
public int getItemCount() {
return forecastList.size();
}
public static class ViewHolder extends RecyclerView.ViewHolder {
private TextView textView;
public ViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(R.id.textView);
}
}
}
```
完成以上步骤后,即可成功制作一个天气预报APP。
相关推荐
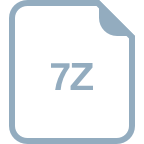
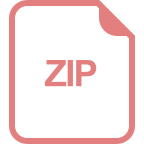














