android实现一个使用和风天气APL来自主获得获取天气预报功能的代码和布局文件
时间: 2024-02-19 11:00:59 浏览: 165
以下是一个简单的示例代码和布局文件,使用和风天气API获取天气预报信息:
1. 在 AndroidManifest.xml 文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.INTERNET"/>
```
2. 在 build.gradle 文件中添加以下依赖项:
```groovy
implementation 'com.squareup.okhttp3:okhttp:4.9.1'
implementation 'com.google.code.gson:gson:2.8.6'
```
3. 创建一个布局文件,例如 activity_main.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp">
<TextView
android:id="@+id/tv_city"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="City"
android:textSize="24sp"
android:textStyle="bold"/>
<TextView
android:id="@+id/tv_weather"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/tv_city"
android:textSize="18sp"/>
<TextView
android:id="@+id/tv_temperature"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/tv_weather"
android:textSize="18sp"/>
</RelativeLayout>
```
4. 在 MainActivity.java 文件中添加以下代码:
```java
import android.os.Bundle;
import android.util.Log;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import com.google.gson.Gson;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import java.io.IOException;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private TextView tvCity;
private TextView tvWeather;
private TextView tvTemperature;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvCity = findViewById(R.id.tv_city);
tvWeather = findViewById(R.id.tv_weather);
tvTemperature = findViewById(R.id.tv_temperature);
// Replace YOUR_API_KEY with your own API key from https://dev.heweather.com/
String apiKey = "YOUR_API_KEY";
String city = "Beijing"; // Replace with your own city name
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://devapi.qweather.com/v7/weather/now?key=" + apiKey + "&location=" + city)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
Log.e(TAG, "Failed to get weather data", e);
}
@Override
public void onResponse(Response response) throws IOException {
String json = response.body().string();
Log.d(TAG, json);
WeatherResponse weatherResponse = new Gson().fromJson(json, WeatherResponse.class);
runOnUiThread(() -> {
tvCity.setText(weatherResponse.getLocation().getName());
tvWeather.setText(weatherResponse.getNow().getText());
tvTemperature.setText(weatherResponse.getNow().getTemp() + "℃");
});
}
});
}
}
```
5. 创建一个类 WeatherResponse.java,用于解析 API 响应:
```java
public class WeatherResponse {
private Location location;
private Now now;
public Location getLocation() {
return location;
}
public void setLocation(Location location) {
this.location = location;
}
public Now getNow() {
return now;
}
public void setNow(Now now) {
this.now = now;
}
public static class Location {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
public static class Now {
private String text;
private String temp;
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public String getTemp() {
return temp;
}
public void setTemp(String temp) {
this.temp = temp;
}
}
}
```
这个示例代码使用 OkHttpClient 发起 HTTP 请求,并使用 Gson 库解析 JSON 响应。在 onResponse 回调中,我们更新 UI 界面显示城市名、天气状态和温度。
请注意:这个示例代码仅供参考,实际项目中需要根据自己的需求进行修改和完善。
阅读全文
相关推荐
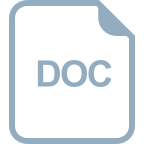
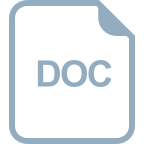
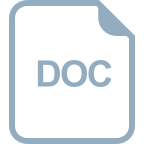


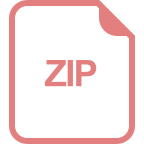
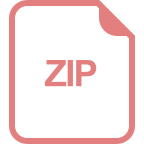
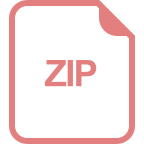
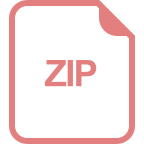
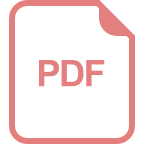
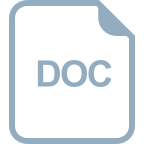







