写出用c++语言的数组来实现贪吃蛇游戏,并用多文件结构管理程序的代码
时间: 2024-03-16 08:42:14 浏览: 23
好的,下面是一个用C++语言的数组来实现贪吃蛇游戏,并用多文件结构管理程序的示例代码:
main.cpp
```cpp
#include <iostream>
#include "snake.h"
#include "food.h"
#include "game.h"
using namespace std;
int main() {
Game game;
game.run();
return 0;
}
```
snake.h
```cpp
#ifndef SNAKE_H
#define SNAKE_H
#include <deque>
class Snake {
public:
Snake(int x, int y);
void move(int dx, int dy);
void grow();
bool checkCollision(int x, int y);
std::pair<int, int> getHead();
std::deque<std::pair<int, int>> getBody();
private:
std::deque<std::pair<int, int>> body;
};
#endif
```
snake.cpp
```cpp
#include "snake.h"
Snake::Snake(int x, int y) {
body.push_back(std::make_pair(x, y));
}
void Snake::move(int dx, int dy) {
int x = body.front().first + dx;
int y = body.front().second + dy;
body.push_front(std::make_pair(x, y));
body.pop_back();
}
void Snake::grow() {
body.push_back(body.back());
}
bool Snake::checkCollision(int x, int y) {
for (auto &p : body) {
if (p.first == x && p.second == y) {
return true;
}
}
return false;
}
std::pair<int, int> Snake::getHead() {
return body.front();
}
std::deque<std::pair<int, int>> Snake::getBody() {
return body;
}
```
food.h
```cpp
#ifndef FOOD_H
#define FOOD_H
#include <utility>
class Food {
public:
Food(int w, int h);
std::pair<int, int> getLocation();
void respawn();
private:
std::pair<int, int> location;
int width;
int height;
};
#endif
```
food.cpp
```cpp
#include "food.h"
#include <cstdlib>
#include <ctime>
Food::Food(int w, int h) : width(w), height(h) {
srand(time(NULL));
respawn();
}
std::pair<int, int> Food::getLocation() {
return location;
}
void Food::respawn() {
int x, y;
do {
x = rand() % width;
y = rand() % height;
} while (x == location.first && y == location.second);
location = std::make_pair(x, y);
}
```
game.h
```cpp
#ifndef GAME_H
#define GAME_H
#include "snake.h"
#include "food.h"
class Game {
public:
Game();
void run();
private:
const int width = 30;
const int height = 20;
Snake snake;
Food food;
bool gameOver;
void draw();
};
#endif
```
game.cpp
```cpp
#include "game.h"
#include <iostream>
#include <conio.h>
using namespace std;
Game::Game() : snake(width / 2, height / 2), food(width, height), gameOver(false) {}
void Game::run() {
while (!gameOver) {
draw();
if (_kbhit()) {
char ch = _getch();
switch (ch) {
case 'a':
snake.move(-1, 0);
break;
case 'd':
snake.move(1, 0);
break;
case 'w':
snake.move(0, -1);
break;
case 's':
snake.move(0, 1);
break;
case 'q':
gameOver = true;
break;
}
}
if (snake.checkCollision(food.getLocation().first, food.getLocation().second)) {
snake.grow();
food.respawn();
}
}
cout << "Game over!" << endl;
}
void Game::draw() {
system("cls");
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (i == 0 || i == height - 1 || j == 0 || j == width - 1) {
cout << "#";
} else {
bool isSnake = false;
for (auto &p : snake.getBody()) {
if (p.first == j && p.second == i) {
cout << "O";
isSnake = true;
break;
}
}
if (!isSnake && food.getLocation().first == j && food.getLocation().second == i) {
cout << "@";
} else if (!isSnake) {
cout << " ";
}
}
}
cout << endl;
}
}
```
注意:这份代码可能与您的系统不兼容,因为它使用了Windows系统特有的conio.h库来获取键盘输入。如果您的系统不支持此库,您可以使用其他库或自己实现获取键盘输入的功能。
相关推荐
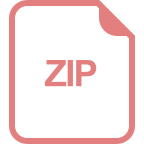
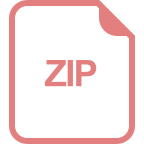















