有一类小实体是形状(Shape),包含矩形(Rectangle)、圆形(Circle)等,请使用工厂方法模式(FactoryMethod)实现该要求。 1)画出类结构图。 2)采用面向对象编程语言实现该工厂方法模式,并写测试程序main(进行测试。
时间: 2024-02-21 15:59:01 浏览: 82
以下是该工厂方法模式的类结构图:
```
+----------------------+ +------------------+
| ShapeFactory |------------>| Shape |
+----------------------+ +------------------+
|+ createShape(type) | |+ draw() |
+----------------------+ |+ getType() |
+------------------+
/_\
|
|
+------------------+ +------------------+
| Rectangle | | Circle |
+------------------+ +------------------+
|+ draw() | |+ draw() |
|+ getType() | |+ getType() |
+------------------+ +------------------+
```
以下是使用 Java 编程语言实现该工厂方法模式的示例代码:
```java
// Shape 接口
public interface Shape {
void draw();
String getType();
}
// Rectangle 类
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle.");
}
@Override
public String getType() {
return "Rectangle";
}
}
// Circle 类
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle.");
}
@Override
public String getType() {
return "Circle";
}
}
// ShapeFactory 类
public class ShapeFactory {
public Shape createShape(String type) {
if (type.equalsIgnoreCase("Rectangle")) {
return new Rectangle();
} else if (type.equalsIgnoreCase("Circle")) {
return new Circle();
}
return null;
}
}
// 测试程序 main
public class Main {
public static void main(String[] args) {
ShapeFactory factory = new ShapeFactory();
Shape shape1 = factory.createShape("Rectangle");
System.out.println("Shape 1 type: " + shape1.getType());
shape1.draw();
Shape shape2 = factory.createShape("Circle");
System.out.println("Shape 2 type: " + shape2.getType());
shape2.draw();
}
}
```
运行测试程序 main,输出结果如下:
```
Shape 1 type: Rectangle
Drawing a rectangle.
Shape 2 type: Circle
Drawing a circle.
```
阅读全文
相关推荐
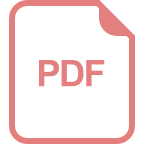
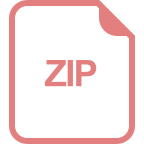










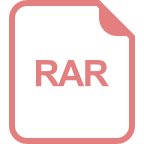
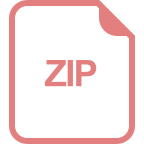
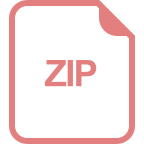
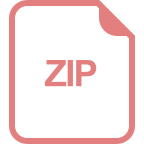
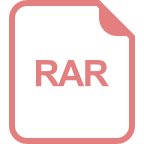
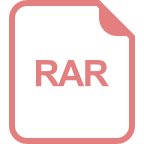