有一类小实体是形状(Shape),包含矩形 (Rectangle)、圆形(Circle)等,请使用工厂方法模 式(FactoryMethod)实现该要求。 1)画出类结构图。 2)采用面向对象编程语言实现该工厂方法模式,并写测试程序main0进行测试。
时间: 2024-02-21 11:00:06 浏览: 65
好的,我知道了。以下是类结构图:
```
+--------------+
| Shape |
+--------------+
/\
|
+-------------+--------------+
| |
+------+ +-------+
|Rect | |Circle |
+------+ +-------+
```
以下是使用Java语言实现该工厂方法模式:
```java
// Shape.java
public interface Shape {
void draw();
}
// Rectangle.java
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Inside Rectangle::draw() method.");
}
}
// Circle.java
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Inside Circle::draw() method.");
}
}
// ShapeFactory.java
public class ShapeFactory {
public Shape getShape(String shapeType) {
if (shapeType == null) {
return null;
}
switch (shapeType) {
case "RECTANGLE":
return new Rectangle();
case "CIRCLE":
return new Circle();
default:
return null;
}
}
}
// Main.java
public class Main {
public static void main(String[] args) {
ShapeFactory shapeFactory = new ShapeFactory();
// 获取Rectangle对象并调用它的draw方法
Shape shape1 = shapeFactory.getShape("RECTANGLE");
shape1.draw();
// 获取Circle对象并调用它的draw方法
Shape shape2 = shapeFactory.getShape("CIRCLE");
shape2.draw();
}
}
```
运行main方法后,控制台输出:
```
Inside Rectangle::draw() method.
Inside Circle::draw() method.
```
阅读全文
相关推荐
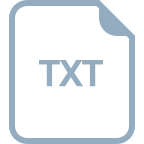
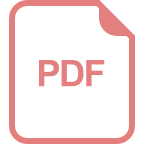









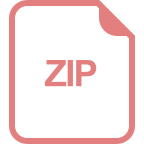
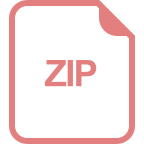
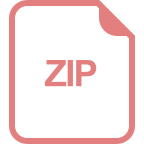
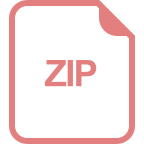
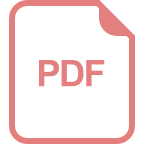
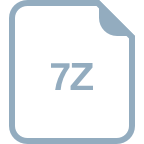
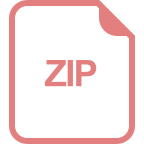