有一类小实体是形状(Shape),包含矩形 (Rectangle)、圆形(Circle)等,请使用工厂方法模式(FactoryMethod)实现该要求
时间: 2023-11-06 22:05:42 浏览: 64
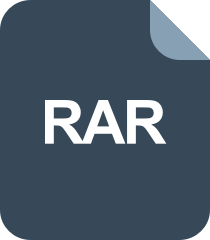
工厂方法模式(FactoryMethod)
好的,我了解了您的要求。以下是使用工厂方法模式实现小实体形状的示例代码:
首先,我们需要定义一个抽象的 Shape 类,用于表示所有形状的基本属性和方法:
```python
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def draw(self):
pass
```
然后,我们可以定义具体的形状类,例如矩形(Rectangle)和圆形(Circle):
```python
class Rectangle(Shape):
def draw(self):
print("Inside Rectangle::draw() method.")
class Circle(Shape):
def draw(self):
print("Inside Circle::draw() method.")
```
接下来,我们需要定义一个 Shape 工厂类,用于根据不同的参数创建不同的形状实例:
```python
class ShapeFactory:
def get_shape(self, shape_type):
if shape_type == 'rectangle':
return Rectangle()
elif shape_type == 'circle':
return Circle()
else:
return None
```
最后,我们可以通过 Shape 工厂类来创建不同的形状实例:
```python
factory = ShapeFactory()
shape1 = factory.get_shape('rectangle')
shape1.draw()
shape2 = factory.get_shape('circle')
shape2.draw()
```
输出结果分别为:
```
Inside Rectangle::draw() method.
Inside Circle::draw() method.
```
这就是使用工厂方法模式实现小实体形状的示例代码。希望对您有帮助!
阅读全文
相关推荐
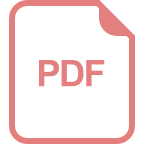









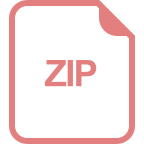
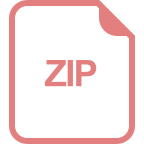
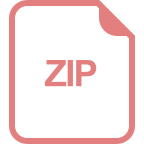
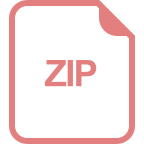
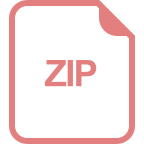
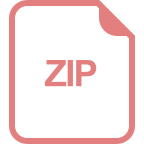
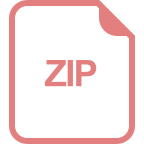