用二次探测在散列解决冲突建立哈希表并实现查找操作C语言代码
时间: 2024-02-17 11:05:31 浏览: 137
下面是使用二次探测法来解决哈希冲突并实现查找操作的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 11
typedef struct {
char* key;
char* value;
} entry;
entry* table[TABLE_SIZE];
int hash(char* key) {
int hash_value = 0;
for (int i = 0; i < strlen(key); i++) {
hash_value += key[i];
}
return hash_value % TABLE_SIZE;
}
void insert(char* key, char* value) {
int index = hash(key);
int i = 1;
while (table[index] != NULL) {
index = (hash(key) + i*i) % TABLE_SIZE;
i++;
}
entry* new_entry = (entry*) malloc(sizeof(entry));
new_entry->key = key;
new_entry->value = value;
table[index] = new_entry;
}
char* search(char* key) {
int index = hash(key);
int i = 1;
while (table[index] != NULL) {
if (strcmp(table[index]->key, key) == 0) {
return table[index]->value;
}
index = (hash(key) + i*i) % TABLE_SIZE;
i++;
}
return NULL;
}
int main() {
insert("apple", "red");
insert("banana", "yellow");
insert("grape", "purple");
printf("%s\n", search("apple"));
printf("%s\n", search("banana"));
printf("%s\n", search("grape"));
printf("%s\n", search("orange"));
return 0;
}
```
该代码中, `entry` 结构体表示哈希表中的每个条目,包含键和值。哈希表是一个指针数组,每个指针指向一个 `entry` 结构体。`hash` 函数根据键计算哈希值。`insert` 函数使用二次探测法解决哈希冲突,并将新的 `entry` 结构体插入哈希表中。`search` 函数根据键在哈希表中查找对应的值。
阅读全文
相关推荐
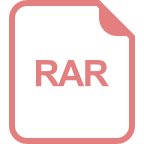



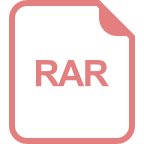
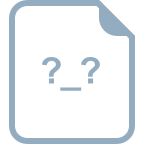
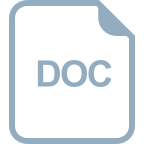
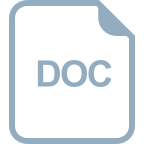





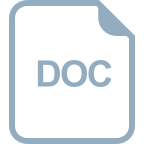
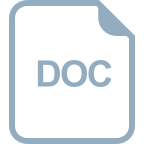
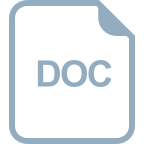