定义一个课程类course,要求所有数据成员定义成private,理解类的定义和使用。 (1)使用#define定义课程容量MAXCAP (2) 定义课程类的属性:课程号id、课程名name、教室loc(可选)、课程容量cap 教师tea、学生stu、已有学生数量stucnt和节次time(可选); (提示:教室loc和教师tea使用前面定义类的对象,学生stu使用学生类数组指针) (3) 定义总课程数crscnt (提示:使用静态变量,在构造函数中加1) (4)定义无参和有参的构造函数;(提示:初始化stu数组,长度为cap) (5)定义拷贝构造函数(提示:初始化stu数组,长度为cap,并将参数的数据成员值 复制到数据成员中) (6)定义析构函数,delete释放stu数组资源,输出课程的简单信息:(7) 定义课程类的功能: 增加选课的学生booladdstu(conststudent&s);(提示:给数组元素赋值,增加stucnt)录入课程内所有学生的分数boolsetcoursescore):(提示:访问stu数组,通过cin录入所有选课学生的分数) 获取班级平均分double averagescore): 求班级最高分的同学student topstu():成绩排序void sort bubble; 输出班级所有同学的信息和成绩voidstuinfo输出班级的课程信息voidcourscinfo0); (8)在main函数,继续声明两个course的变量,使用这些成员函数进行计算。
时间: 2023-07-15 12:14:17 浏览: 39
以下是课程类course的定义:
```cpp
#include<iostream>
#include<string>
using namespace std;
#define MAXCAP 50
class student {
private:
string name;
int score;
public:
student() {}
student(string n, int s) : name(n), score(s) {}
friend istream& operator>>(istream& in, student& s) {
in >> s.name >> s.score;
return in;
}
friend ostream& operator<<(ostream& out, const student& s) {
out << s.name << '\t' << s.score;
return out;
}
};
class course {
private:
static int crscnt;
string id;
string name;
string loc;
int cap;
string tea;
student* stu;
int stucnt;
string time;
public:
course() : cap(MAXCAP), stu(new student[MAXCAP]), stucnt(0) {
crscnt++;
}
course(string i, string n, string l, int c, string t, string tm) :
id(i), name(n), loc(l), cap(c), tea(t), stu(new student[c]), stucnt(0), time(tm) {
crscnt++;
}
course(const course& c) :
id(c.id), name(c.name), loc(c.loc), cap(c.cap), tea(c.tea), stu(new student[c.cap]), stucnt(c.stucnt), time(c.time) {
for (int i = 0; i < c.stucnt; i++) {
stu[i] = c.stu[i];
}
}
~course() {
cout << "Course " << id << " (" << name << ") is released." << endl;
delete[] stu;
}
bool addstu(const student& s) {
if (stucnt < cap) {
stu[stucnt++] = s;
return true;
}
else {
return false;
}
}
bool setcoursescore() {
for (int i = 0; i < stucnt; i++) {
cin >> stu[i];
}
return true;
}
double averagescore() {
double sum = 0;
for (int i = 0; i < stucnt; i++) {
sum += stu[i].score;
}
return sum / stucnt;
}
student topstu() {
int maxscore = 0;
student top;
for (int i = 0; i < stucnt; i++) {
if (stu[i].score > maxscore) {
maxscore = stu[i].score;
top = stu[i];
}
}
return top;
}
void sortbubble() {
for (int i = 0; i < stucnt - 1; i++) {
for (int j = 0; j < stucnt - i - 1; j++) {
if (stu[j].score < stu[j + 1].score) {
swap(stu[j], stu[j + 1]);
}
}
}
}
void stuinfo() {
cout << "Student List:" << endl;
for (int i = 0; i < stucnt; i++) {
cout << stu[i] << endl;
}
}
void courscinfo() {
cout << "Course Info:" << endl;
cout << "ID: " << id << endl;
cout << "Name: " << name << endl;
cout << "Location: " << loc << endl;
cout << "Capacity: " << cap << endl;
cout << "Teacher: " << tea << endl;
cout << "Time: " << time << endl;
}
};
int course::crscnt = 0;
int main() {
course c1("C001", "Math", "Room 101", 50, "Tom", "Monday 7-9");
course c2("C002", "English", "Room 102", 40, "Jerry", "Tuesday 1-3");
student s1("John", 90);
student s2("Lucy", 80);
student s3("Mike", 85);
c1.addstu(s1);
c1.addstu(s2);
c2.addstu(s2);
c2.addstu(s3);
c1.setcoursescore();
c2.setcoursescore();
cout << "Course 1 Average Score: " << c1.averagescore() << endl;
cout << "Course 2 Average Score: " << c2.averagescore() << endl;
cout << "Course 1 Top Student: " << c1.topstu() << endl;
cout << "Course 2 Top Student: " << c2.topstu() << endl;
c1.sortbubble();
c2.sortbubble();
c1.stuinfo();
c2.stuinfo();
c1.courscinfo();
c2.courscinfo();
return 0;
}
```
相关推荐
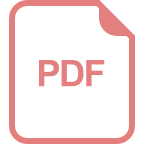
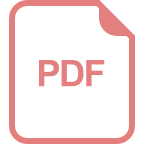















