八叉树体素网格可视化python代码
时间: 2023-07-03 09:03:43 浏览: 180
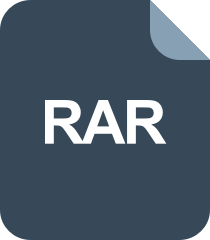
八叉树代码
以下是一个简单的八叉树体素网格可视化的 Python 代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
class Octree:
def __init__(self, min_point, max_point, depth=0, max_depth=5):
self.min_point = min_point
self.max_point = max_point
self.depth = depth
self.max_depth = max_depth
self.children = [None]*8
self.is_leaf = True
self.points = []
def insert(self, point):
if self.depth == self.max_depth:
self.points.append(point)
return
idx = self.get_octant_idx(point)
if self.children[idx] is None:
self.children[idx] = self.create_octant(idx)
self.is_leaf = False
self.children[idx].insert(point)
def create_octant(self, idx):
x, y, z = self.get_octant_center(idx)
min_point = np.array([x, y, z])
max_point = np.array([self.max_point[0] if idx & 4 else x,
self.max_point[1] if idx & 2 else y,
self.max_point[2] if idx & 1 else z])
return Octree(min_point, max_point, self.depth+1, self.max_depth)
def get_octant_center(self, idx):
x = (self.min_point[0] + self.max_point[0])/2.0
y = (self.min_point[1] + self.max_point[1])/2.0
z = (self.min_point[2] + self.max_point[2])/2.0
if idx & 4:
x = (x + self.max_point[0])/2.0
else:
x = (x + self.min_point[0])/2.0
if idx & 2:
y = (y + self.max_point[1])/2.0
else:
y = (y + self.min_point[1])/2.0
if idx & 1:
z = (z + self.max_point[2])/2.0
else:
z = (z + self.min_point[2])/2.0
return x, y, z
def get_octant_idx(self, point):
idx = 0
if point[0] > (self.min_point[0] + self.max_point[0])/2.0:
idx |= 4
if point[1] > (self.min_point[1] + self.max_point[1])/2.0:
idx |= 2
if point[2] > (self.min_point[2] + self.max_point[2])/2.0:
idx |= 1
return idx
def get_leaf_nodes(self):
if self.is_leaf:
return [self]
leaf_nodes = []
for child in self.children:
if child is not None:
leaf_nodes.extend(child.get_leaf_nodes())
return leaf_nodes
def plot_cube(cube_definition, ax):
x = [cube_definition[0][0], cube_definition[1][0], cube_definition[2][0], cube_definition[3][0],
cube_definition[0][0], cube_definition[4][0], cube_definition[5][0], cube_definition[1][0]]
y = [cube_definition[0][1], cube_definition[1][1], cube_definition[2][1], cube_definition[3][1],
cube_definition[0][1], cube_definition[4][1], cube_definition[5][1], cube_definition[1][1]]
z = [cube_definition[0][2], cube_definition[1][2], cube_definition[2][2], cube_definition[3][2],
cube_definition[0][2], cube_definition[4][2], cube_definition[5][2], cube_definition[1][2]]
verts = [list(zip(x, y, z))]
ax.add_collection3d(Poly3DCollection(verts, facecolors='blue', linewidths=1, edgecolors='r', alpha=.25))
def plot_octree(node, ax):
if node.is_leaf:
return
for child in node.children:
if child is not None:
plot_cube([(child.min_point[0], child.min_point[1], child.min_point[2]),
(child.max_point[0], child.min_point[1], child.min_point[2]),
(child.max_point[0], child.max_point[1], child.min_point[2]),
(child.min_point[0], child.max_point[1], child.min_point[2]),
(child.min_point[0], child.min_point[1], child.max_point[2]),
(child.max_point[0], child.min_point[1], child.max_point[2]),
(child.max_point[0], child.max_point[1], child.max_point[2]),
(child.min_point[0], child.max_point[1], child.max_point[2])], ax)
plot_octree(child, ax)
def main():
points = np.random.rand(100, 3)
min_point = np.min(points, axis=0)
max_point = np.max(points, axis=0)
octree = Octree(min_point, max_point, max_depth=5)
for point in points:
octree.insert(point)
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
plot_octree(octree, ax)
ax.scatter(points[:,0], points[:,1], points[:,2], c='r', marker='o')
ax.set_xlabel('X Label')
ax.set_ylabel('Y Label')
ax.set_zlabel('Z Label')
plt.show()
if __name__ == '__main__':
main()
```
这个代码使用 matplotlib 库绘制了八叉树体素网格,并在其中添加了随机生成的点。您可以根据需要调整点的数量和八叉树的深度。
阅读全文
相关推荐
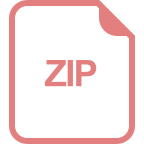


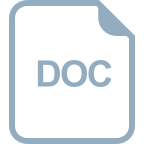

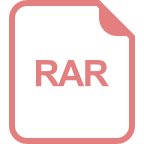
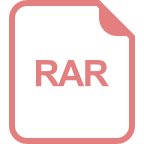
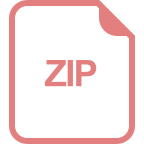
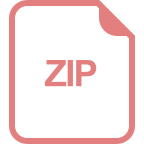
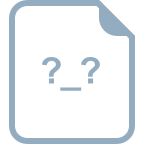

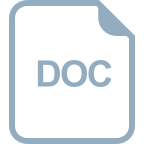
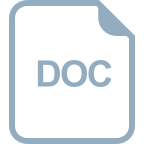



