解释一下这段代码import json import pytest import requests from pytest_xlsx.file import XlsxItem def pytest_xlsx_run_step(item: XlsxItem): session: requests.Session = item.usefixtures['session'] # fixture step = item.current_step # 读取excel内容 resp = session.request( # 发送请求 method=step["标记"], url=step["接口地址"], headers=json.loads(step["请求头"]), data=step["参数"] ) assert resp.status_code == step["状态码"] # 断言 return True @pytest.fixture def session(): return requests.Session()
时间: 2023-06-03 21:05:18 浏览: 160
这段代码引入了三个模块:json、pytest和requests,以及一个pytest_xlsx的XlsxItem类。其中,XlsxItem类是用于对Excel文件内容进行解析和操作的类。
在函数def pytest_xlsx_run_step(item: XlsxItem)中,从item.usefixtures['session']中获取了一个请求会话的session对象。这个session对象可以用于在测试过程中进行网络请求和数据交互。
相关问题
Pytest+requests+allure+excel+log+mail如何实现接口自动化
要实现接口自动化,可以按照以下步骤进行:
1. 安装pytest、requests、allure、openpyxl、logbook、smtplib等Python库。
2. 创建一个测试用例文件,使用pytest框架编写测试用例,使用requests库发送接口请求,使用openpyxl库读取Excel文件中的测试数据。
3. 使用allure框架生成测试报告,可以通过命令行或pytest.ini配置文件进行配置。
4. 使用logbook库记录测试过程中的日志信息,可以将日志保存到文件或发送到邮件。
5. 使用smtplib库发送测试结果邮件,可以将测试报告、日志等信息一并发送。
下面是一个简单的示例代码,可以根据自己的需求进行修改:
```
import pytest
import requests
import openpyxl
import allure
import logbook
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
# 创建日志记录器
logger = logbook.Logger('API Test')
# 读取Excel文件中的测试数据
def read_test_data(filename, sheetname):
wb = openpyxl.load_workbook(filename)
sheet = wb[sheetname]
data = []
for row in sheet.iter_rows(min_row=2):
case = {}
case['name'] = row[0].value
case['url'] = row[1].value
case['method'] = row[2].value
case['headers'] = eval(row[3].value)
case['params'] = eval(row[4].value)
case['expected'] = eval(row[5].value)
data.append(case)
return data
# 发送接口请求
def send_request(url, method, headers, params):
if method == 'get':
res = requests.get(url, headers=headers, params=params)
elif method == 'post':
res = requests.post(url, headers=headers, json=params)
else:
logger.error('Invalid request method')
res = None
return res
# 编写测试用例
@allure.feature('API Test')
@pytest.mark.parametrize('case', read_test_data('test_data.xlsx', 'Sheet1'))
def test_api(case):
logger.info(f"Test case: {case['name']}")
with allure.step(f"Send {case['method'].upper()} request to {case['url']}"):
res = send_request(case['url'], case['method'], case['headers'], case['params'])
with allure.step('Verify response'):
assert res.json() == case['expected']
# 生成测试报告
@pytest.fixture(scope='session', autouse=True)
def attach_report():
yield
allure_cmd = 'allure generate allure-results --clean -o allure-report'
os.system(allure_cmd)
# 发送邮件
def send_mail():
# 邮件内容
msg = MIMEMultipart()
msg['Subject'] = 'API Test Report'
msg['From'] = 'sender@example.com'
msg['To'] = 'receiver@example.com'
text = MIMEText('Please check the attachment for API test report')
msg.attach(text)
with open('allure-report.zip', 'rb') as f:
report = MIMEApplication(f.read(), 'zip')
report.add_header('Content-Disposition', 'attachment', filename='API Test Report.zip')
msg.attach(report)
# 发送邮件
smtp_server = 'smtp.example.com'
smtp_port = 25
smtp_user = 'sender@example.com'
smtp_password = 'password'
server = smtplib.SMTP(smtp_server, smtp_port)
server.login(smtp_user, smtp_password)
server.sendmail('sender@example.com', 'receiver@example.com', msg.as_string())
server.quit()
# 在测试结束后发送邮件
def pytest_terminal_summary(terminalreporter):
if terminalreporter.failed:
logger.error('API Test failed')
else:
logger.info('API Test passed')
send_mail()
```
这个示例代码中,使用了pytest框架编写测试用例,使用allure框架生成测试报告,使用logbook库记录日志信息,使用smtplib库发送邮件。在编写测试用例时,使用了openpyxl库读取Excel文件中的测试数据,使用requests库发送接口请求。在测试结束后,通过pytest_terminal_summary钩子发送邮件,将测试报告、日志等信息发送给指定的邮箱。
python+requests+excel+pytest+allure接口自动化
Python是一种广泛使用的编程语言,因其易学易用、灵活性和可扩展性而备受欢迎。requests是Python的一个库,它提供了一种简单且易于使用的方式来发送HTTP请求。pytest是Python的另一个库,它提供了一种用于编写和运行测试的框架。allure是一个测试报告生成工具,可以为测试结果提供美观和易读的报告。
在接口自动化测试中,可以使用Python的requests库来发送HTTP请求,并使用pytest框架来编写和运行测试。可以使用Excel来存储测试数据、预期结果和实际结果。使用allure工具可以生成美观的测试报告。
以下是使用Python requests、pytest和allure进行接口自动化测试的一般步骤:
1. 安装Python、requests、pytest和allure
2. 创建一个Excel文件,输入测试数据、预期结果和实际结果
3. 创建一个pytest测试文件,并使用requests库发送HTTP请求,比较预期结果和实际结果
4. 在pytest测试文件中添加allure装饰器,以便生成测试报告
5. 运行pytest测试文件并生成allure测试报告
例如,以下是一个使用Python requests、pytest和allure进行接口自动化测试的示例代码:
```python
import requests
import pytest
import allure
import openpyxl
@allure.title("测试接口")
@pytest.mark.parametrize("test_input, expected", [(1, "success"), (2, "fail")])
def test_api(test_input, expected):
# 从Excel文件中获取测试数据和预期结果
wb = openpyxl.load_workbook("testdata.xlsx")
sheet = wb.active
test_data = sheet.cell(row=test_input, column=1).value
expected_result = sheet.cell(row=test_input, column=2).value
# 发送HTTP请求
response = requests.get("http://example.com/api", params=test_data)
actual_result = response.text
# 比较预期结果和实际结果
assert actual_result == expected_result, f"预期结果:{expected_result},实际结果:{actual_result}"
# 添加allure描述
allure.attach("请求参数", str(test_data))
allure.attach("预期结果", str(expected_result))
allure.attach("实际结果", str(actual_result))
```
在上面的代码中,使用了pytest的parametrize装饰器来传递测试数据和预期结果。使用openpyxl库从Excel文件中获取测试数据和预期结果。使用requests库发送HTTP请求并比较预期结果和实际结果。使用allure的装饰器来添加测试描述。最后,运行pytest测试文件并生成allure测试报告。
阅读全文
相关推荐
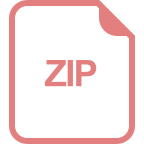
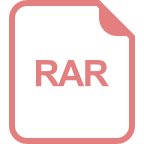
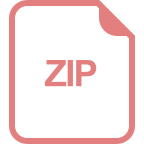
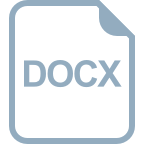
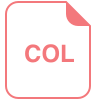
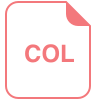
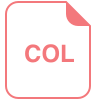
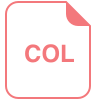
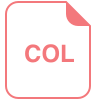
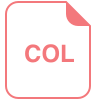
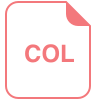
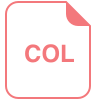
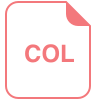


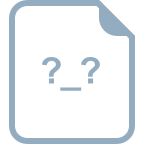
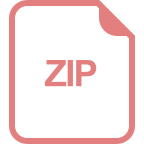
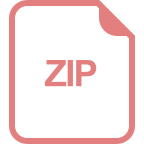