vue 数据库 登录界面_vue实现的上传图片到数据库并显示到页面功能示例
时间: 2023-08-12 20:05:45 浏览: 80
好的,我可以为您提供一个简单的示例。首先,您需要一个具有上传图片功能的表单,如下所示:
```html
<template>
<div>
<form @submit.prevent="onSubmit">
<input type="file" ref="fileInput" @change="onFileChange">
<button type="submit">上传</button>
</form>
<img :src="imageUrl" v-if="imageUrl" alt="上传的图片">
</div>
</template>
```
接下来,您可以在 Vue 组件中实现上传和显示图片的功能。在 `methods` 中定义 `onFileChange` 方法来处理选定的文件,使用 `FileReader` API 将文件读入内存,并将其存储在 `imageData` 变量中。然后,您可以使用 `axios` 库将图像数据发送到服务器并将其保存到数据库中。最后,在 `onSubmit` 方法中,您将使用 `imageUrl` 变量来显示上传的图像。
```javascript
<template>
<div>
<form @submit.prevent="onSubmit">
<input type="file" ref="fileInput" @change="onFileChange">
<button type="submit">上传</button>
</form>
<img :src="imageUrl" v-if="imageUrl" alt="上传的图片">
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
imageData: null,
imageUrl: null
}
},
methods: {
onFileChange(event) {
const file = event.target.files[0];
const reader = new FileReader();
reader.onload = (e) => {
this.imageData = e.target.result;
};
reader.readAsDataURL(file);
},
onSubmit() {
// 将 imageData 发送到服务器并将其保存到数据库中
axios.post('/api/upload', { imageData: this.imageData }).then((response) => {
this.imageUrl = response.data.imageUrl;
}).catch((error) => {
console.log(error);
});
}
}
}
</script>
```
在服务器端,您需要将接收到的图像数据解码并将其保存到数据库中。以下是一个 Node.js Express 路由的示例:
```javascript
const express = require('express');
const router = express.Router();
const multer = require('multer');
const upload = multer();
router.post('/upload', upload.single('imageData'), (req, res) => {
const imageData = req.file.buffer.toString('base64');
// 将 imageData 保存到数据库中
const imageUrl = 'data:image/png;base64,' + imageData;
res.json({ imageUrl });
});
module.exports = router;
```
请注意,此示例仅用于演示目的,并且并不是最佳实践。在实际应用中,您需要更多的验证和错误处理来确保安全性和可靠性。
阅读全文
相关推荐
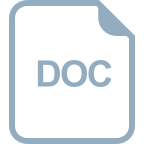
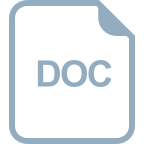
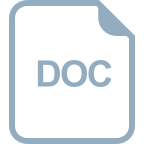
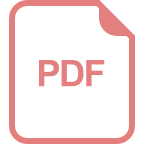
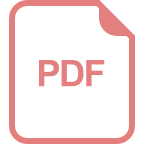


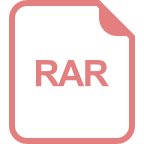
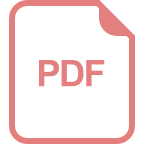
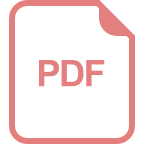
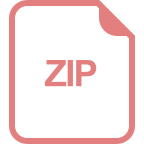
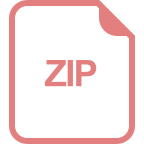
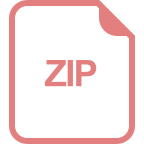
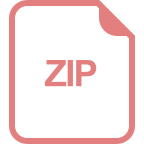
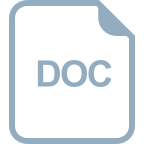



