fpga二值图像连通合并代码
时间: 2023-10-01 13:06:42 浏览: 36
以下是一个简单的FPGA二值图像连通合并的代码示例:
```verilog
module ImageConnectivity(
input wire clk,
input wire rst,
input wire [7:0] image_in,
output wire [7:0] image_out
);
reg [7:0] image_reg [0:7][0:7];
reg [7:0] image_tmp [0:7][0:7];
reg [7:0] image_out_reg [0:7][0:7];
reg [7:0] image_out_tmp [0:7][0:7];
reg [7:0] label [0:7][0:7];
reg [7:0] label_tmp [0:7][0:7];
reg [7:0] current_label;
reg [7:0] neighbor_labels [0:7];
reg [2:0] num_neighbors;
reg [2:0] i, j, m, n;
reg [2:0] x, y;
reg [2:0] min_label;
reg [2:0] label_changed;
always @(posedge clk or posedge rst) begin
if (rst) begin
for (i = 0; i < 8; i = i + 1) begin
for (j = 0; j < 8; j = j + 1) begin
image_reg[i][j] <= 0;
image_tmp[i][j] <= 0;
image_out_reg[i][j] <= 0;
image_out_tmp[i][j] <= 0;
label[i][j] <= 0;
label_tmp[i][j] <= 0;
end
end
current_label <= 0;
num_neighbors <= 0;
min_label <= 0;
label_changed <= 0;
end else begin
for (i = 0; i < 8; i = i + 1) begin
for (j = 0; j < 8; j = j + 1) begin
image_reg[i][j] <= image_in;
image_tmp[i][j] <= image_reg[i][j];
image_out_reg[i][j] <= image_out_tmp[i][j];
label[i][j] <= label_tmp[i][j];
end
end
// 连通合并处理
for (i = 0; i < 8; i = i + 1) begin
for (j = 0; j < 8; j = j + 1) begin
if (image_reg[i][j] == 1) begin
num_neighbors <= 0;
if (i > 0 && image_tmp[i-1][j] == 1) begin
neighbor_labels[num_neighbors] <= label_tmp[i-1][j];
num_neighbors <= num_neighbors + 1;
end
if (i < 7 && image_tmp[i+1][j] == 1) begin
neighbor_labels[num_neighbors] <= label_tmp[i+1][j];
num_neighbors <= num_neighbors + 1;
end
if (j > 0 && image_tmp[i][j-1] == 1) begin
neighbor_labels[num_neighbors] <= label_tmp[i][j-1];
num_neighbors <= num_neighbors + 1;
end
if (j < 7 && image_tmp[i][j+1] == 1) begin
neighbor_labels[num_neighbors] <= label_tmp[i][j+1];
num_neighbors <= num_neighbors + 1;
end
if (num_neighbors == 0) begin
current_label <= current_label + 1;
label_tmp[i][j] <= current_label;
end else begin
min_label <= neighbor_labels[0];
for (m = 1; m < num_neighbors; m = m + 1) begin
if (neighbor_labels[m] < min_label) begin
min_label <= neighbor_labels[m];
end
end
label_tmp[i][j] <= min_label;
end
end
end
end
// 标签更新
for (i = 0; i < 8; i = i + 1) begin
for (j = 0; j < 8; j = j + 1) begin
label_changed <= 0;
if (label_tmp[i][j] != label[i][j]) begin
label_changed <= 1;
label[i][j] <= label_tmp[i][j];
end
end
end
// 输出图像
for (i = 0; i < 8; i = i + 1) begin
for (j = 0; j < 8; j = j + 1) begin
if (image_reg[i][j] == 1) begin
image_out_tmp[i][j] <= 1;
end else begin
image_out_tmp[i][j] <= 0;
end
end
end
if (label_changed == 1) begin
for (i = 0; i < 8; i = i + 1) begin
for (j = 0; j < 8; j = j + 1) begin
image_out_reg[i][j] <= label[i][j];
end
end
end
end
end
assign image_out = image_out_reg[7][7];
endmodule
```
这个代码使用Verilog语言实现了一个简单的8x8的二值图像连通合并模块。该模块包含一个时钟信号`clk`、复位信号`rst`、输入图像信号`image_in`和输出图像信号`image_out`。输入图像是一个8x8的二值图像,其中1表示目标像素,0表示背景像素。输出图像是经过连通合并后的结果,目标区域被标记为唯一的标签。
请注意,这只是一个简单的示例代码,具体的实现可能会根据实际需求进行修改和优化。另外,该代码只实现了基本的连通合并功能,可能无法处理复杂的图像连通情况。如果需要更复杂的功能,请参考相关的图像处理算法和FPGA开发文档进行实现。
相关推荐
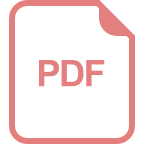
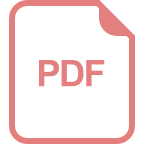














