编写一个学生和教师的数据输入和显示程序。学生数据有编号、姓名、性别、年龄、系别和成绩,教师数据有编号、姓名、性别、年龄、职称和部门。要求将编号、姓名、性别、年龄的输入和输出设计成一个类Person,并作为学生类Student和教师类Teacher的基类
时间: 2023-12-22 11:19:21 浏览: 76
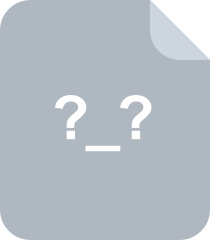
编写一个学生和教师数据输入和显示程序
以下是基于您的要求编写的程序:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
int id;
string name;
char gender;
int age;
public:
void input() {
cout << "请输入编号:";
cin >> id;
cout << "请输入姓名:";
cin >> name;
cout << "请输入性别(M/F):";
cin >> gender;
cout << "请输入年龄:";
cin >> age;
}
void display() {
cout << "编号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
}
};
class Student : public Person {
private:
string department;
double score;
public:
void input() {
Person::input();
cout << "请输入系别:";
cin >> department;
cout << "请输入成绩:";
cin >> score;
}
void display() {
Person::display();
cout << "系别:" << department << endl;
cout << "成绩:" << score << endl;
}
};
class Teacher : public Person {
private:
string title;
string department;
public:
void input() {
Person::input();
cout << "请输入职称:";
cin >> title;
cout << "请输入部门:";
cin >> department;
}
void display() {
Person::display();
cout << "职称:" << title << endl;
cout << "部门:" << department << endl;
}
};
int main() {
Student s;
cout << "请输入学生信息:" << endl;
s.input();
cout << endl << "学生信息如下:" << endl;
s.display();
cout << endl;
Teacher t;
cout << "请输入教师信息:" << endl;
t.input();
cout << endl << "教师信息如下:" << endl;
t.display();
return 0;
}
```
程序中定义了一个基类`Person`,包含编号、姓名、性别和年龄四个属性,同时也提供了输入和显示这四个属性的方法。然后通过继承`Person`类,定义了学生类`Student`和教师类`Teacher`,在这两个类中分别添加了系别、成绩和职称、部门两个属性,同时也重载了输入和显示方法。在`main`函数中,分别实例化了一个`Student`对象`s`和一个`Teacher`对象`t`,并调用了它们的输入和显示方法。
您可以根据需要对程序进行修改和完善。
阅读全文
相关推荐
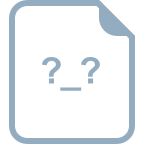









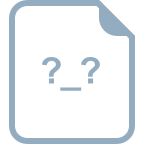
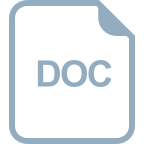

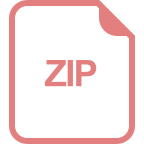
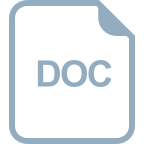
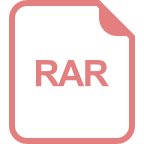