经过TCP/IP协议栈发送802.1Q报文
时间: 2023-07-20 11:33:08 浏览: 144
要经过TCP/IP协议栈发送802.1Q报文,需要在数据包中添加802.1Q VLAN标签,并将其发送到网络接口。以下是一个简单的示例程序,演示如何使用TCP/IP协议栈发送带有802.1Q VLAN标签的数据包:
```c++
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <arpa/inet.h>
#include <net/ethernet.h>
#include <net/if.h>
#include <netinet/in.h>
#include <netinet/ip.h>
#include <netinet/tcp.h>
#include <netinet/udp.h>
#include <netinet/ip_icmp.h>
#include <linux/if_packet.h>
#include <linux/if_ether.h>
#include <linux/if_vlan.h>
int main()
{
int sock = socket(PF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
if (sock < 0) {
perror("socket");
exit(1);
}
char ifname[IFNAMSIZ] = "eth0";
struct ifreq ifr;
strncpy(ifr.ifr_name, ifname, IFNAMSIZ - 1);
if (ioctl(sock, SIOCGIFINDEX, &ifr) < 0) {
perror("ioctl");
exit(1);
}
struct sockaddr_ll addr;
memset(&addr, 0, sizeof(addr));
addr.sll_family = AF_PACKET;
addr.sll_ifindex = ifr.ifr_ifindex;
// 构造802.1Q VLAN标签
struct vlan_tag {
uint16_t tci;
uint16_t ether_type;
} __attribute__((packed));
struct vlan_tag vlan;
vlan.tci = htons(0x100);
vlan.ether_type = htons(ETH_P_IP);
// 构造IP数据包
char ip_packet[ETH_FRAME_LEN];
struct iphdr *iph = (struct iphdr *)ip_packet;
iph->version = 4;
iph->ihl = 5;
iph->tos = 0;
iph->tot_len = htons(sizeof(struct iphdr) + sizeof(struct udphdr));
iph->id = htons(0);
iph->frag_off = htons(0);
iph->ttl = 64;
iph->protocol = IPPROTO_UDP;
iph->check = htons(0);
iph->saddr = inet_addr("192.168.1.100");
iph->daddr = inet_addr("192.168.1.1");
// 构造UDP数据包
char udp_packet[ETH_FRAME_LEN];
struct udphdr *udph = (struct udphdr *)udp_packet;
udph->source = htons(1234);
udph->dest = htons(5678);
udph->len = htons(sizeof(struct udphdr));
udph->check = htons(0);
// 将IP头和UDP数据拷贝到数据包中
memcpy(ip_packet + sizeof(struct iphdr), udph, sizeof(struct udphdr));
// 将802.1Q VLAN标签和IP/UDP数据拷贝到数据包中
char packet[ETH_FRAME_LEN];
memcpy(packet, "\xff\xff\xff\xff\xff\xff", ETH_ALEN);
memcpy(packet + ETH_ALEN, "\x00\x11\x22\x33\x44\x55", ETH_ALEN);
memcpy(packet + 2 * ETH_ALEN, &vlan, sizeof(struct vlan_tag));
memcpy(packet + 2 * ETH_ALEN + sizeof(struct vlan_tag), ip_packet, sizeof(struct iphdr) + sizeof(struct udphdr));
// 发送数据包
if (sendto(sock, packet, 2 * ETH_ALEN + sizeof(struct vlan_tag) + sizeof(struct iphdr) + sizeof(struct udphdr), 0, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("sendto");
exit(1);
}
close(sock);
return 0;
}
```
该程序使用socket API创建了一个PF_PACKET类型的socket,并将其绑定到网络接口上。然后,程序构造了一个带有802.1Q VLAN标签的IP/UDP数据包,并将其封装成一个以太网帧。在构造802.1Q VLAN标签时,程序使用了vlan_tag结构体来定义标签格式,并将其添加到数据包的头部。最后,程序调用sendto函数将数据包发送到目标MAC地址。
阅读全文
相关推荐
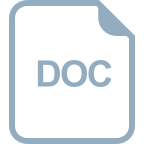
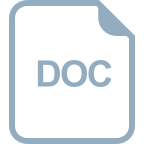
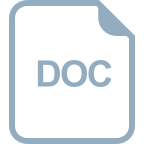
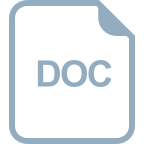
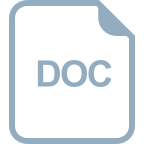
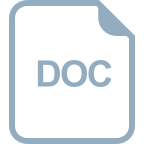
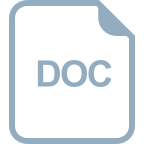
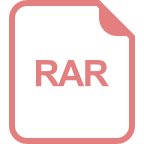
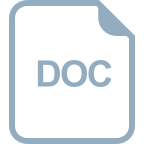
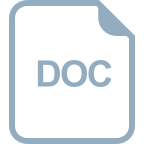
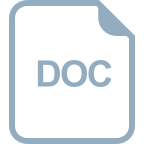
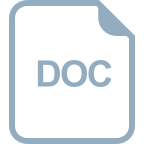
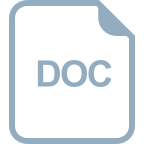