写一段stm32控制麦轮的代码
时间: 2023-09-24 07:05:27 浏览: 96
### 回答1:
#include "stm32f4xx_hal.h"void Motor_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
/* GPIO Ports Clock Enable */
__HAL_RCC_GPIOE_CLK_ENABLE();
/*Configure GPIO pin */
GPIO_InitStruct.Pin = GPIO_PIN_7;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(GPIOE, &GPIO_InitStruct);
/*Set initial state*/
HAL_GPIO_WritePin(GPIOE, GPIO_PIN_7, GPIO_PIN_RESET);
}void Motor_Control(uint8_t direction)
{
if (direction == 0)
HAL_GPIO_WritePin(GPIOE, GPIO_PIN_7, GPIO_PIN_RESET);
else
HAL_GPIO_WritePin(GPIOE, GPIO_PIN_7, GPIO_PIN_SET);
}答案:stm32控制麦轮的代码示例如下:
#include "stm32f4xx_hal.h"void Motor_Init()
{
GPIO_InitTypeDef GPIO_InitStruct;
/* GPIO端口时钟使能*/
__HAL_RCC_GPIOE_CLK_ENABLE();
/*配置GPIO端口*/
GPIO_InitStruct.Pin = GPIO_PIN_7;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(GPIOE,& GPIO_InitStruct);
/*设置初始状态*/
HAL_GPIO_WritePin(GPIOE,GPIO_PIN_7,GPIO_PIN_RESET);
}void Motor_Control(uint8_t direction)
{
if(direction == 0)
HAL_GPIO_WritePin(GPIOE,GPIO_PIN_7,GPIO_PIN_RESET);
else
HAL_GPIO_WritePin(GPIOE,GPIO_PIN_7,GPIO_PIN_SET);
}
### 回答2:
下面是一个简单的麦轮控制代码示例,使用STM32微控制器编写:
```c
#include "stm32f4xx.h"
#define MOT1_PWM_PIN GPIO_Pin_0
#define MOT1_DIR1_PIN GPIO_Pin_1
#define MOT1_DIR2_PIN GPIO_Pin_2
#define MOT2_PWM_PIN GPIO_Pin_3
#define MOT2_DIR1_PIN GPIO_Pin_4
#define MOT2_DIR2_PIN GPIO_Pin_5
#define MOT3_PWM_PIN GPIO_Pin_6
#define MOT3_DIR1_PIN GPIO_Pin_7
#define MOT3_DIR2_PIN GPIO_Pin_8
#define MOT4_PWM_PIN GPIO_Pin_9
#define MOT4_DIR1_PIN GPIO_Pin_10
#define MOT4_DIR2_PIN GPIO_Pin_11
void motor_init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
// Enable the GPIO clocks
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
// Configure motor control pins as outputs
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
// Motor 1
GPIO_InitStructure.GPIO_Pin = MOT1_PWM_PIN | MOT1_DIR1_PIN | MOT1_DIR2_PIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Motor 2
GPIO_InitStructure.GPIO_Pin = MOT2_PWM_PIN | MOT2_DIR1_PIN | MOT2_DIR2_PIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Motor 3
GPIO_InitStructure.GPIO_Pin = MOT3_PWM_PIN | MOT3_DIR1_PIN | MOT3_DIR2_PIN;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// Motor 4
GPIO_InitStructure.GPIO_Pin = MOT4_PWM_PIN | MOT4_DIR1_PIN | MOT4_DIR2_PIN;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void motor_control(uint8_t motorNum, uint8_t speed, uint8_t direction)
{
GPIO_TypeDef* GPIOx;
uint16_t pwmPin, dir1Pin, dir2Pin;
switch(motorNum) {
case 1:
GPIOx = GPIOA;
pwmPin = MOT1_PWM_PIN;
dir1Pin = MOT1_DIR1_PIN;
dir2Pin = MOT1_DIR2_PIN;
break;
case 2:
GPIOx = GPIOA;
pwmPin = MOT2_PWM_PIN;
dir1Pin = MOT2_DIR1_PIN;
dir2Pin = MOT2_DIR2_PIN;
break;
case 3:
GPIOx = GPIOB;
pwmPin = MOT3_PWM_PIN;
dir1Pin = MOT3_DIR1_PIN;
dir2Pin = MOT3_DIR2_PIN;
break;
case 4:
GPIOx = GPIOB;
pwmPin = MOT4_PWM_PIN;
dir1Pin = MOT4_DIR1_PIN;
dir2Pin = MOT4_DIR2_PIN;
break;
default:
// Invalid motor number
return;
}
// Set speed
TIM_SetCompareX(TIMx, pwmPin, speed);
// Set direction
if (direction == 1) {
GPIO_SetBits(GPIOx, dir1Pin);
GPIO_ResetBits(GPIOx, dir2Pin);
} else if (direction == 2) {
GPIO_ResetBits(GPIOx, dir1Pin);
GPIO_SetBits(GPIOx, dir2Pin);
} else {
GPIO_ResetBits(GPIOx, dir1Pin);
GPIO_ResetBits(GPIOx, dir2Pin);
}
}
int main(void)
{
// 初始化麦轮电机
motor_init();
// 控制麦轮电机
motor_control(1, 50, 1); // 控制 motorNum=1 的麦轮以速度 50 和方向 1 运行
while(1) {
// 循环执行其他任务
}
}
```
以上是一个简单的示例代码,展示了如何使用STM32控制麦轮电机。代码中通过初始化GPIO管脚并设置时钟,然后通过`motor_control`函数来控制每个麦轮的速度和方向。在`main`函数中,我们首先初始化了麦轮电机,然后通过`motor_control`函数来控制特定麦轮运行。在`while`循环中,您可以添加其他任务或逻辑。请根据实际情况适应代码。
### 回答3:
下面是一段基于STM32的控制麦轮的简单代码示例:
首先,需要引入STM32 HAL库和相关的头文件。在主函数中,初始化GPIO引脚作为输出,用于控制麦轮的旋转方向。初始化定时器和PWM通道来控制麦轮的速度。代码如下:
```c
#include "stm32f4xx_hal.h"
#include "stm32f4xx_hal_gpio.h"
#include "stm32f4xx_hal_tim.h"
// 定义引脚
#define M1_Pin GPIO_PIN_0
#define M1_GPIO_Port GPIOA
#define M2_Pin GPIO_PIN_1
#define M2_GPIO_Port GPIOA
// 定义定时器和PWM通道
#define TIMx TIM2
#define M1_PWM_Channel TIM_CHANNEL_1
#define M2_PWM_Channel TIM_CHANNEL_2
int main(void)
{
// 初始化STM32 HAL库
HAL_Init();
// 初始化GPIO引脚
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Pin = M1_Pin|M2_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
// 初始化定时器和PWM通道
TIM_HandleTypeDef htim;
TIM_OC_InitTypeDef sConfigOC;
htim.Instance = TIMx;
htim.Init.Prescaler = 0;
htim.Init.CounterMode = TIM_COUNTERMODE_UP;
htim.Init.Period = 1000;
htim.Init.ClockDivision = TIM_CLOCKDIVISION_DIV1;
HAL_TIM_PWM_Init(&htim);
sConfigOC.OCMode = TIM_OCMODE_PWM1;
sConfigOC.Pulse = 0;
sConfigOC.OCPolarity = TIM_OCPOLARITY_HIGH;
sConfigOC.OCFastMode = TIM_OCFAST_DISABLE;
HAL_TIM_PWM_ConfigChannel(&htim, &sConfigOC, M1_PWM_Channel);
HAL_TIM_PWM_ConfigChannel(&htim, &sConfigOC, M2_PWM_Channel);
// 启动定时器
HAL_TIM_PWM_Start(&htim, M1_PWM_Channel);
HAL_TIM_PWM_Start(&htim, M2_PWM_Channel);
while (1)
{
// 控制麦轮的旋转方向和速度
HAL_GPIO_WritePin(M1_GPIO_Port, M1_Pin, GPIO_PIN_SET); // M1正转
HAL_GPIO_WritePin(M2_GPIO_Port, M2_Pin, GPIO_PIN_RESET); // M2反转
__HAL_TIM_SET_COMPARE(&htim, M1_PWM_Channel, 800); // 设置M1的PWM占空比为80%
__HAL_TIM_SET_COMPARE(&htim, M2_PWM_Channel, 500); // 设置M2的PWM占空比为50%
HAL_Delay(1000); // 延时1秒
}
}
```
这段代码中,通过控制GPIO引脚的高低电平来控制麦轮的旋转方向,通过PWM占空比来控制麦轮的速度。在这个示例中,M1正转、M2反转,M1的PWM占空比为80%,M2的PWM占空比为50%。
相关推荐
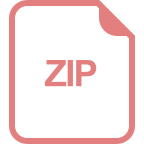
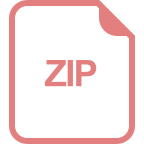











