利用消息缓冲通信方式,可实现消息的发送与接收,编写发送进程sndfile.c将要发送的消息从键盘输入,每输入一行就作为一条消息发送,用end结束消息,编写接收进程rcvfile.c从消息队里逐个接收信息并显示,用end结束消息。原来的好像有问题
时间: 2024-02-23 20:01:08 浏览: 25
好的,我理解了你的问题。下面是利用消息缓冲通信方式,实现消息的发送与接收的代码示例:
1. 发送进程sndfile.c
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAX_MSG_SIZE 1024
typedef struct {
long type;
char text[MAX_MSG_SIZE];
} message;
int main() {
int msqid;
key_t key;
message msg;
char buffer[MAX_MSG_SIZE];
// 生成键值
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 获取消息队列
if ((msqid = msgget(key, 0666 | IPC_CREAT)) == -1) {
perror("msgget");
exit(1);
}
// 输入消息并发送
printf("Input message: ");
while (fgets(buffer, MAX_MSG_SIZE, stdin) != NULL) {
// 去掉换行符
buffer[strlen(buffer) - 1] = '\0';
// 将消息类型设置为1
msg.type = 1;
// 将消息内容复制到msg.text中
strcpy(msg.text, buffer);
// 发送消息
if (msgsnd(msqid, &msg, strlen(msg.text) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
// 如果输入的消息内容为"end",则表示输入结束
if (strcmp(buffer, "end") == 0) {
break;
}
// 继续输入下一条消息
printf("Input message: ");
}
// 删除消息队列
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
2. 接收进程rcvfile.c
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MAX_MSG_SIZE 1024
typedef struct {
long type;
char text[MAX_MSG_SIZE];
} message;
int main() {
int msqid;
key_t key;
message msg;
// 生成键值
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 获取消息队列
if ((msqid = msgget(key, 0666 | IPC_CREAT)) == -1) {
perror("msgget");
exit(1);
}
// 循环接收消息并显示
while (1) {
// 接收消息
if (msgrcv(msqid, &msg, MAX_MSG_SIZE, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
// 如果接收到的消息内容为"end",则表示接收结束
if (strcmp(msg.text, "end") == 0) {
break;
}
// 显示消息内容
printf("Received message: %s\n", msg.text);
}
return 0;
}
```
这两个程序分别编译后,在两个不同的终端中运行即可。在发送进程中,输入要发送的消息,每输入一行就作为一条消息发送,用"end"结束消息。在接收进程中,逐个接收消息并显示,用"end"结束消息。
相关推荐
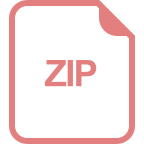
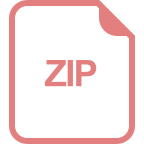















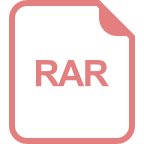