用python制作text文件词云图的代码
时间: 2024-05-11 16:15:05 浏览: 92
很高兴为您解答!以下是用Python制作text文件词云图的代码:
```python
# 导入需要的库
from wordcloud import WordCloud
import jieba
import matplotlib.pyplot as plt
# 读取文本内容
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词并去除停用词
text_list = jieba.cut(text)
stopwords = [line.strip() for line in open('stopwords.txt',encoding='UTF-8').readlines()]
words = [word for word in text_list if word not in stopwords]
# 拼接成字符串
words_str = " ".join(words)
# 生成词云图
wc = WordCloud(background_color="white",width=1000, height=600, font_path='msyh.ttc')
wc.generate(words_str)
# 显示词云图
plt.imshow(wc)
plt.axis('off')
plt.show()
```
希望对您有帮助!如果您还有其它问题,欢迎随时提出。
相关问题
python制作txt文件词云图代码模板
Sure, here's a basic code template to create a word cloud from a text file in Python:
```python
import matplotlib.pyplot as plt
from wordcloud import WordCloud
from pathlib import Path
# path to the text file
file_path = "path/to/your/text/file.txt"
# read the text file
text = Path(file_path).read_text()
# create the word cloud
wordcloud = WordCloud().generate(text)
# plot the word cloud
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
You can customize this code template by adding more options to the `WordCloud` constructor, such as changing the font, background color, and word frequency threshold. Hope this helps!
制作python代码实现词云图制作
好的,制作词云图可以使用Python中的wordcloud库。首先需要用Python读取需要生成词云的文本文件,然后使用wordcloud库生成词云图。以下是一个简单的示例代码:
```python
import jieba
from wordcloud import WordCloud
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
words = jieba.cut(text)
# 合并分词结果为字符串
words_str = ' '.join(words)
# 生成词云图
wordcloud = WordCloud(font_path='msyh.ttc', width=800, height=600, background_color='white').generate(words_str)
# 保存词云图
wordcloud.to_file('wordcloud.png')
```
以上代码使用jieba库对文本进行分词,然后使用WordCloud类生成词云图,最后保存为PNG格式的图片。其中,font_path参数指定了字体文件路径,width和height参数指定了词云图的宽和高,background_color参数指定了词云图的背景颜色。你可以根据需要修改这些参数。
阅读全文
相关推荐
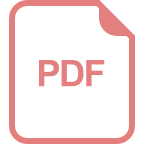
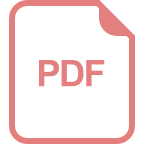
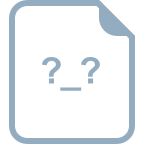


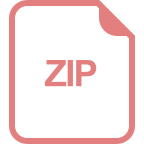
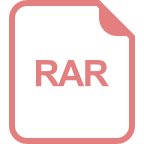








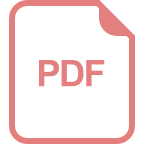