通过java的if..else条件语句找出3个数 a,b,c中最小的数
时间: 2024-02-01 09:15:03 浏览: 69
可以使用 if..else 条件语句来实现该功能,具体代码如下:
```java
if (a <= b && a <= c) {
System.out.println("最小值为:" + a);
} else if (b <= a && b <= c) {
System.out.println("最小值为:" + b);
} else {
System.out.println("最小值为:" + c);
}
```
首先判断 a 是否为最小值,如果是则输出 a,否则继续判断 b 是否为最小值,如果是则输出 b,否则说明 c 是最小值,输出 c。
相关问题
java 用if else 语句实现从键盘上输入三个数字 并且从大到小输出
在Java中,你可以使用`Scanner`类从键盘获取用户输入,并结合`if-else`语句判断并排序这三个数。以下是一个简单的示例代码:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入三个整数:");
int num1 = scanner.nextInt();
int num2 = scanner.nextInt();
int num3 = scanner.nextInt();
// 使用 if-else 判断并输出
if (num1 >= num2 && num1 >= num3) {
System.out.println("最大的数字是:" + num1);
} else if (num2 >= num1 && num2 >= num3) {
System.out.println("最大的数字是:" + num2);
} else {
System.out.println("最大的数字是:" + num3);
}
if (num1 <= num2 && num1 <= num3) {
System.out.println("最小的数字是:" + num1);
} else if (num2 <= num1 && num2 <= num3) {
System.out.println("最小的数字是:" + num2);
} else {
System.out.println("最小的数字是:" + num3);
}
scanner.close();
}
}
```
在这个代码里,首先读取三个整数,然后通过两个嵌套的`if-else`结构分别找出最大值和最小值并输出。
package wzybf; import java.util.Arrays; public class IntSort { // 对两个数进行排序输出 public static void sort(int a, int b) { if (a > b) { System.out.println(b + " " + a); } else { System.out.println(a + " " + b); } } // 对三个数进行排序输出 public static void sort(int a, int b, int c) { if (a > b) { int temp = a; a = b; b = temp; } if (a > c) { int temp = a; a = c; c = temp; } if (b > c) { int temp = b; b = c; c = temp; } System.out.println(a + " " + b + " " + c); } // 对数组进行排序输出 public static void sort(int[] arr) { Arrays.sort(arr); for (int num : arr) { System.out.print(num + " "); } System.out.println(); } public static void main(String[] args) { IntSort.sort(5, 3); IntSort.sort(2, 4, 1); IntSort.sort(new int[]{5, 4, 6, 2, 8, 1}); } }程序设计过程中一般遇到的问题及解决过程;
在编写程序时,经常会遇到一些问题,下面列举一些可能出现的问题及解决过程:
1. 语法错误:在编写程序时,可能会出现语法错误,如拼写错误、缺少分号等。解决方法是仔细检查代码,逐行排查错误,也可以使用IDE工具自动检查语法错误。
2. 运行错误:在程序运行时,可能会出现各种错误,如数组越界、空指针异常等。解决方法是使用try-catch语句捕获异常,或者在编写程序时进行判断,避免出现异常。
3. 性能问题:在处理大量数据时,程序可能会出现性能问题,如运行缓慢、内存泄漏等。解决方法是优化程序,使用合适的数据结构和算法,减少不必要的计算和内存占用。
4. 逻辑错误:在编写程序时,可能会出现逻辑错误,即程序输出结果与预期不符。解决方法是仔细分析问题,检查代码逻辑,进行调试和测试,找出问题所在。
总之,在编写程序时,需要耐心细致地思考和排查问题,不断完善程序,才能达到预期的效果。
阅读全文
相关推荐
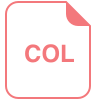
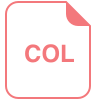
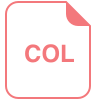
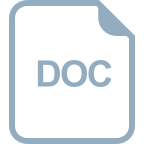
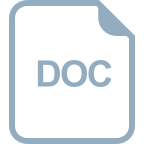
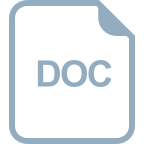
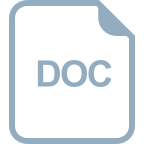
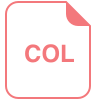
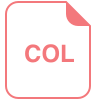




