栈的实现及应用代码 c语言代码
时间: 2024-01-02 20:03:27 浏览: 81
栈是一种数据结构,它具有后进先出(LIFO)的特点。栈的实现可以使用数组或链表,下面是使用数组实现的栈的示例代码:
```
#include <stdio.h>
#define MAX_SIZE 100
int stack[MAX_SIZE];
int top = -1;
void push(int item) {
if (top >= MAX_SIZE - 1) {
printf("Stack Overflow\n");
return;
}
top++;
stack[top] = item;
}
int pop() {
if (top < 0) {
printf("Stack Underflow\n");
return -1;
}
int item = stack[top];
top--;
return item;
}
int main() {
push(1);
push(2);
push(3);
printf("%d\n", pop());
printf("%d\n", pop());
printf("%d\n", pop());
printf("%d\n", pop());
return 0;
}
```
上述代码中,`push` 函数将元素压入栈中,`pop` 函数将元素弹出栈。如果栈已满,`push` 函数将返回“栈溢出”,如果栈为空,`pop` 函数将返回“栈下溢”。
栈可以应用于很多场景,例如:
1. 表达式求值
2. 括号匹配
3. 进制转换
4. 递归函数调用
5. 浏览器前进后退历史记录等
以上是一些常见的应用,栈还可以应用于更多领域。
阅读全文
相关推荐
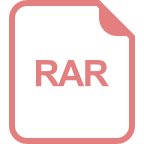
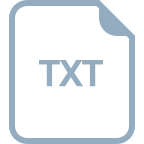
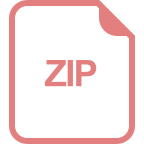














