drawcontours()函数解析 c++
时间: 2024-04-08 16:29:22 浏览: 12
`drawContours()`函数是OpenCV中用于在图像上绘制轮廓的函数。它的函数原型如下:
```cpp
void cv::drawContours(cv::InputOutputArray image, cv::InputArrayOfArrays contours, int contourIdx, const cv::Scalar &color, int thickness = 1, int lineType = LINE_8, cv::InputArray hierarchy = noArray(), int maxLevel = INT_MAX, cv::Point offset = Point())
```
参数解析如下:
- `image`:要绘制轮廓的图像,必须是CV_8UC3类型的。
- `contours`:包含轮廓点集的向量,每个轮廓是一个点集。
- `contourIdx`:绘制的轮廓索引,为-1时表示绘制所有轮廓。
- `color`:绘制轮廓的颜色,为cv::Scalar类型,可以是BGR颜色或灰度值。
- `thickness`:轮廓线的粗细,默认为1。
- `lineType`:轮廓线的类型,默认为LINE_8,表示8连接线。
- `hierarchy`:可选参数,包含轮廓层次结构信息的可选输入数组。
- `maxLevel`:可选参数,绘制的轮廓的最大层级,默认为INT_MAX。
- `offset`:可选参数,轮廓坐标的偏移量。
注意,`drawContours()`函数会在传入的图像上直接绘制轮廓,并不会返回绘制了轮廓的新图像。
下面是一个使用`drawContours()`函数的示例:
```cpp
#include <opencv2/opencv.hpp>
int main() {
// 读取图像
cv::Mat image = cv::imread("test.jpg");
// 转换为灰度图像
cv::Mat gray;
cv::cvtColor(image, gray, cv::COLOR_BGR2GRAY);
// 二值化处理
cv::Mat binary;
cv::threshold(gray, binary, 127, 255, cv::THRESH_BINARY);
// 查找轮廓
std::vector<std::vector<cv::Point>> contours;
cv::findContours(binary, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
// 在图像上绘制轮廓
cv::drawContours(image, contours, -1, cv::Scalar(0, 0, 255), 2);
// 显示图像
cv::imshow("image", image);
cv::waitKey(0);
cv::destroyAllWindows();
return 0;
}
```
在这个例子中,我们首先读取图像,然后将其转换为灰度图像并进行二值化处理。接下来,使用`findContours()`函数找到图像中的轮廓,并将轮廓存储在`contours`向量中。最后,使用`drawContours()`函数在原始图像上绘制轮廓,并显示结果。
需要注意的是,在C++中,需要使用`cv::Mat`和`std::vector<cv::Point>`等OpenCV的数据结构来处理图像和轮廓。
相关推荐
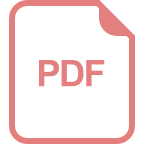
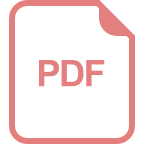














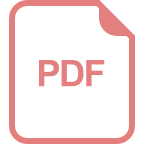