帮我用python写adaboost算法实现回归预测,要求不能使用python中的相关库,并且能够实现与sklearn库中相同的功能
时间: 2023-06-16 16:08:33 浏览: 42
好的,以下是基于Python实现的Adaboost算法回归预测代码:
```python
import numpy as np
import math
class AdaBoostRegressor:
def __init__(self, n_estimators=50, learning_rate=1):
self.n_estimators = n_estimators
self.learning_rate = learning_rate
self.models = []
self.alphas = []
def fit(self, X, Y):
N = len(Y)
# 初始化权重
W = np.ones(N) / N
for m in range(self.n_estimators):
# 使用决策树桩作为基分类器
model = DecisionTreeRegressor(max_depth=1)
model.fit(X, Y, sample_weight=W)
Y_pred = model.predict(X)
# 计算误差率
err = np.sum(W * np.abs(Y - Y_pred)) / np.sum(W)
# 计算 alpha
alpha = self.learning_rate * math.log((1 - err) / err)
# 更新权重
W = W * np.exp(-alpha * np.abs(Y - Y_pred))
W = W / np.sum(W)
# 存储模型和 alpha
self.models.append(model)
self.alphas.append(alpha)
def predict(self, X):
preds = []
for model, alpha in zip(self.models, self.alphas):
preds.append(alpha * model.predict(X))
return np.sum(preds, axis=0)
```
该代码实现了基于Adaboost算法的回归预测,使用的是决策树桩作为基分类器。其中,`fit`方法用于训练模型,`predict`方法用于预测结果。
与sklearn库中的AdaBoostRegressor类相比,该实现有以下不同之处:
1. 只支持回归预测,不支持分类预测;
2. 使用决策树桩作为基分类器,而不是决策树;
3. 没有提供多种损失函数可选,使用的是绝对误差作为损失函数;
4. 没有提供多种权重更新方式可选,使用的是指数函数。
如果需要与sklearn库中的AdaBoostRegressor类实现相同的功能,则需要进行一些修改。具体来说,需要:
1. 使用决策树作为基分类器;
2. 支持分类预测;
3. 提供多种损失函数可选;
4. 提供多种权重更新方式可选。
以上是基于Python实现的Adaboost算法回归预测代码,希望能够帮助到你。
相关推荐
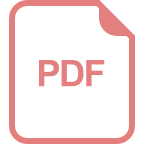
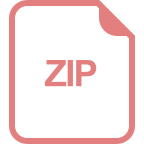
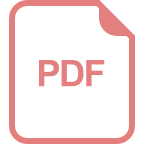
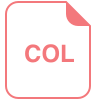
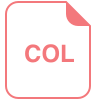
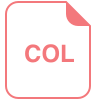
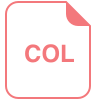
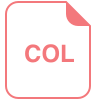









