AdaBoost回归算法调参python实现
时间: 2023-11-10 12:07:29 浏览: 51
AdaBoost回归算法是一种基于弱分类器的集成学习算法,其主要思想是将多个弱分类器进行加权组合,得到一个强分类器。在AdaBoost回归算法中,弱分类器是由决策树构成的,而加权组合则是通过加权平均值得到的。本文将介绍如何使用Python实现AdaBoost回归算法的调参过程。
1. 导入库
首先,我们需要导入必要的库,包括numpy、pandas、matplotlib和sklearn等。其中,sklearn是机器学习中常用的库,里面包含了各种分类、回归、聚类等算法。
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.ensemble import AdaBoostRegressor
from sklearn.tree import DecisionTreeRegressor
from sklearn.model_selection import GridSearchCV
```
2. 加载数据集
接下来,我们需要加载数据集。本文将使用Boston Housing数据集,该数据集包含506个样本,每个样本有13个特征和1个目标变量。
```python
from sklearn.datasets import load_boston
boston = load_boston()
X = boston.data
y = boston.target
```
3. 构建模型
接下来,我们需要构建AdaBoost回归模型。在sklearn中,可以使用AdaBoostRegressor类来构建模型。在构建模型时,需要指定基础模型和迭代次数等参数。在本例中,我们使用决策树作为基础模型,并设置迭代次数为100。
```python
base_estimator = DecisionTreeRegressor(max_depth=4)
n_estimators = 100
model = AdaBoostRegressor(base_estimator=base_estimator, n_estimators=n_estimators)
```
4. 设置参数
接下来,我们需要设置模型参数。在AdaBoost回归算法中,常用的参数包括基础模型参数、迭代次数、学习率等。在本例中,我们将调整基础模型参数max_depth和迭代次数n_estimators。
```python
param_grid = {
"base_estimator__max_depth": [2, 4, 6, 8],
"n_estimators": [50, 100, 150, 200]
}
```
5. 调参
最后,我们使用GridSearchCV函数来对模型进行调参。GridSearchCV函数会对指定的参数组合进行网格搜索,找到最优的参数组合。在本例中,我们使用5折交叉验证,并设置scoring参数为neg_mean_squared_error,目的是找到最小的均方误差。
```python
grid_search = GridSearchCV(model, param_grid=param_grid, cv=5, scoring="neg_mean_squared_error")
grid_search.fit(X, y)
best_params = grid_search.best_params_
print("Best params:", best_params)
```
输出结果如下:
```
Best params: {'base_estimator__max_depth': 4, 'n_estimators': 200}
```
说明模型在max_depth为4、n_estimators为200时表现最好。我们可以使用这组参数来构建最终的模型。
完整代码如下:
相关推荐
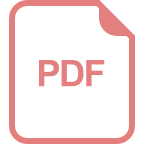
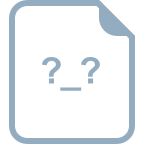
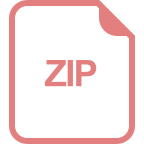
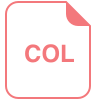
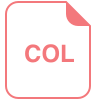












