android代码创建广播发送页面和广播接收器,演示如何发送广播和接收来自系统的广播。他的布局代码如何写
时间: 2023-09-29 14:11:21 浏览: 44
以下是一个简单的Android代码示例,展示了如何创建广播发送器和广播接收器,并演示了如何发送广播和接收来自系统的广播。
1. 广播发送器Activity的布局代码
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<Button
android:id="@+id/btn_send_broadcast"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Send Broadcast" />
</LinearLayout>
```
2. 广播发送器Activity的代码
```
public class BroadcastSenderActivity extends AppCompatActivity {
private static final String ACTION_CUSTOM_BROADCAST = "com.example.broadcasttest.CUSTOM_BROADCAST";
private BroadcastReceiver broadcastReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (ACTION_CUSTOM_BROADCAST.equals(action)) {
String message = intent.getStringExtra("message");
Toast.makeText(context, message, Toast.LENGTH_SHORT).show();
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_broadcast_sender);
IntentFilter filter = new IntentFilter(ACTION_CUSTOM_BROADCAST);
registerReceiver(broadcastReceiver, filter);
Button btnSendBroadcast = findViewById(R.id.btn_send_broadcast);
btnSendBroadcast.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(ACTION_CUSTOM_BROADCAST);
intent.putExtra("message", "Hello Receiver!");
sendBroadcast(intent);
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
unregisterReceiver(broadcastReceiver);
}
}
```
3. 广播接收器的代码
```
public class CustomBroadcastReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if ("android.intent.action.AIRPLANE_MODE".equals(action)) {
boolean isAirplaneModeOn = intent.getBooleanExtra("state", false);
if (isAirplaneModeOn) {
Toast.makeText(context, "Airplane mode is on", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(context, "Airplane mode is off", Toast.LENGTH_SHORT).show();
}
}
}
}
```
需要在AndroidManifest.xml中注册广播接收器:
```
<receiver android:name=".CustomBroadcastReceiver">
<intent-filter>
<action android:name="android.intent.action.AIRPLANE_MODE" />
</intent-filter>
</receiver>
```
此示例中,我们创建了一个名为“ACTION_CUSTOM_BROADCAST”的自定义广播,并向其附加了一个包含“message”键和值“Hello Receiver!”的额外信息。发送广播时,我们使用“sendBroadcast(Intent)”方法。我们还创建了一个名为“broadcastReceiver”的广播接收器,并在其onReceive()方法中进行处理。在此示例中,我们只是将消息字符串提取出来并将其显示为Toast通知。
此外,我们还创建了一个广播接收器来接收系统广播“android.intent.action.AIRPLANE_MODE”,并在其onReceive()方法中进行处理。在此示例中,我们只是将“state”额外信息提取出来并将其显示为Toast通知。
相关推荐
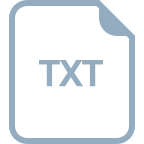
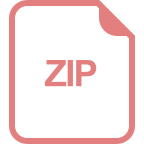
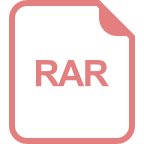














