如何实现hashmap数据的排序
时间: 2023-09-14 08:05:26 浏览: 112
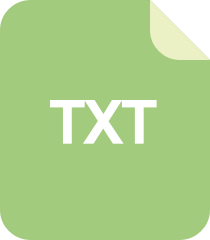
HashMap排序
### 回答1:
实现hashmap数据的排序可以采用以下方法:
1. 将hashmap的键值对转换为List集合,然后通过集合的排序方法进行排序。
2. 利用TreeMap数据结构,TreeMap底层采用红黑树数据结构,可以自动将元素按照键值进行排序。
3. 使用Java8新特性中的Stream API,将hashmap转换为流,然后使用sorted方法对流进行排序。
具体实现方法可以参考以下代码示例:
1. 使用List集合排序
```
Map<String, Integer> map = new HashMap<>();
map.put("apple", 5);
map.put("orange", 3);
map.put("banana", 8);
List<Map.Entry<String, Integer>> list = new ArrayList<>(map.entrySet());
Collections.sort(list, new Comparator<Map.Entry<String, Integer>>() {
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o1.getValue().compareTo(o2.getValue());
}
});
for (Map.Entry<String, Integer> entry : list) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
```
2. 使用TreeMap数据结构
```
Map<String, Integer> map = new HashMap<>();
map.put("apple", 5);
map.put("orange", 3);
map.put("banana", 8);
Map<String, Integer> sortedMap = new TreeMap<>(map);
for (Map.Entry<String, Integer> entry : sortedMap.entrySet()) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
```
3. 使用Stream API排序
```
Map<String, Integer> map = new HashMap<>();
map.put("apple", 5);
map.put("orange", 3);
map.put("banana", 8);
Map<String, Integer> sortedMap = map.entrySet().stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue, (e1, e2) -> e1, LinkedHashMap::new));
for (Map.Entry<String, Integer> entry : sortedMap.entrySet()) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
```
### 回答2:
要实现HashMap数据的排序,我们可以使用Java中的TreeMap。TreeMap是基于红黑树的实现,根据键来对元素进行排序。
首先,我们可以创建一个空的TreeMap对象,并将HashMap中的所有元素逐个放入TreeMap中。可以使用HashMap的entrySet()方法获取HashMap中所有的键值对,然后遍历这些键值对,将键值对依次放入TreeMap中。
接下来,我们可以使用TreeMap的keySet()方法获取已排序的键的集合。遍历这个集合,可以根据键获取对应的值,并进行排序的操作。
下面是一个示例代码:
```
import java.util.*;
public class HashMapSort {
public static void main(String[] args) {
HashMap<String, Integer> hashMap = new HashMap<>();
hashMap.put("apple", 5);
hashMap.put("banana", 2);
hashMap.put("orange", 8);
TreeMap<String, Integer> treeMap = new TreeMap<>(hashMap);
for(String key : treeMap.keySet()) {
System.out.println(key + ": " + treeMap.get(key));
}
}
}
```
输出结果将会是按键进行排序后的结果:
```
apple: 5
banana: 2
orange: 8
```
通过以上步骤,就成功实现了对HashMap数据的排序。
### 回答3:
实现HashMap数据的排序可以按照以下步骤进行:
1. 将HashMap的键值对转换为List。
- 首先,创建一个新的ArrayList对象,并将HashMap的所有键值对(Entry)添加到列表中。
2. 使用Collections.sort()方法对List进行排序。
- 使用Collections.sort()方法可以对List进行排序,需要传入一个Comparator对象来指定排序规则。
3. 创建一个自定义的Comparator对象来指定排序规则。
- 自定义Comparator对象,可以根据HashMap的键或值进行排序。例如,可以实现按照键的升序排序或者值的降序排序。
4. 实现自定义Comparator对象的compare()方法。
- 在compare()方法中实现比较逻辑,根据需要将键或值进行比较并返回排序顺序。
5. 对List进行排序。
- 使用Collections.sort()方法对List进行排序,传入自定义的Comparator对象作为参数。
6. 遍历已排序的List,输出排序后的结果。
- 遍历已排序的List,并输出键值对。可以通过getKey()和getValue()方法获取键和值。
综上所述,实现HashMap数据的排序可以通过将HashMap的键值对转换为List,使用Collections.sort()方法对List排序,并使用自定义的Comparator对象指定排序规则来实现。
阅读全文
相关推荐
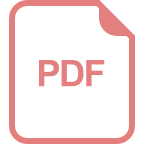
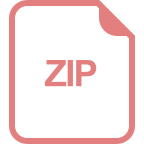
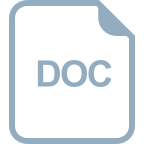
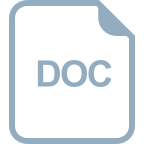



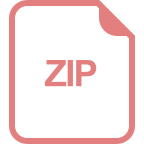
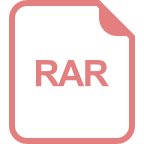
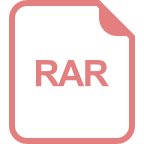
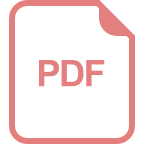
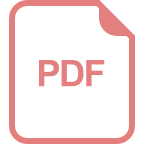
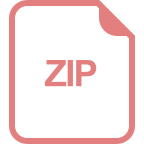

