帮我解释这段代码:#include <cstdio> #include <cstring> #include <string> #include <iostream> #include <algorithm> #include <queue> using namespace std; string s; // http://www.cplusplus.com/reference/queue/priority_queue/ // min heap; if default (the 3rd parameter), max heap priority_queue <int, vector<int>, greater<int> > q; int main() { while(getline(cin, s) && s != "END"){ // create the priority_queue int t = 1; sort(s.begin(), s.end()); // for frequency t (times) for(int i = 1; i < s.length(); i++){ if(s[i] != s[i-1]){ q.push(t); t = 1; } else t++; } q.push(t); // only one type of character if(q.size() == 1) { printf("%d %d 8.0\n", s.length()*8, s.length()); q.pop(); continue; } int ans = 0; // the length by using Huffman Coding while(q.size() > 1){ int a = q.top(); q.pop(); int b = q.top(); q.pop(); q.push(a+b); ans += a+b; } q.pop(); printf("%d %d %.1lf\n", s.length()*8, ans, (double)s.length()*8.0/(double)ans); } }
时间: 2024-01-25 21:03:07 浏览: 109
这段代码实现了哈夫曼编码,用于数据压缩。哈夫曼编码是一种前缀编码,它将频率较高的字符用较短的编码表示,频率较低的字符用较长的编码表示,从而减少了数据传输的总长度。以下是代码的主要步骤:
1. 读入字符串 s。
2. 对字符串 s 进行字符排序,统计每个字符出现的次数,并将次数存入一个小根堆 priority_queue 中。
3. 如果字符串中只有一种字符,则直接输出字符串长度和 8.0,即每个字符均用 8 位二进制表示。
4. 否则,不断从小根堆中取出两个出现次数最少的字符,合并它们的出现次数,然后将新的出现次数压入小根堆中。重复上述步骤,直到小根堆中只剩下一个元素,此时该元素的出现次数即为哈夫曼编码后的总长度。
5. 输出字符串长度、哈夫曼编码后的总长度和压缩比。
需要注意的是,本代码使用 STL 中的 priority_queue 实现小根堆,其中第二个参数 vector<int> 表示使用 vector 存储堆的元素,第三个参数 greater<int> 表示使用小于号作为堆的比较函数。
相关问题
c++map#include<iostream> #include<cstdio> #include<cstring> #include<map> #include<sstream>请解释以上头文件
这段代码包含了多个C++标准库的头文件,它们的作用如下:
1. `<iostream>`: 这是输入输出流库,用于处理基本的I/O操作,如输入输出、流对象等,常用于与用户交互或读写数据。
2. `<cstdio>`: 也称为stdio.h,这是C语言的标准输入输出库,在C++中被编译器链接为同名的头文件,提供了文件输入输出、字符处理等功能。
3. `<cstring>`: 这个库包含处理字符串的函数,比如复制字符串、查找子串等,对C风格的字符串操作非常有用。
4. `<map>`: 这是一个关联容器,属于C++ Standard Template Library (STL),用来存储键值对,通常以模板形式提供通用的数据结构。`std::map`使用红黑树(Red-Black Tree)实现,提供了高效的查找、插入和删除操作,键通常是唯一的。
5. `<sstream>`: 字符串流库,允许开发者将数据转换成字符串流(stringstream)进行操作,这对于处理格式化字符串和输入输出特别有用,特别是当需要复杂的文本格式转换时。
#include <iostream> #include <fstream> #include <cstdio> #include <cstdlib> #include <cstring> #include <cmath> #include <climits> #include <string> #include <algorithm> #include <vector> #include <map> using namespace std; struct Value { char str[10000]; }; void structSort(Value *a, int n) { @你的代码 } int n; Value a[5000]; int main() { scanf("%d", &n); for (int i = 0; i<n; i++) { scanf("%s", a[i].str); } structSort(a, n); for (int i = 0; i<n; i++) { printf("%s\n", a[i].str); } return 0; }
这段代码给出了一个结构体数组 `a`,其中每个结构体包含一个字符串成员 `str`。接下来有一个函数 `structSort`,它需要你来实现。你需要使用任何一种排序算法对 `a` 数组进行排序,排序的依据是字符串的字典序(即按照字母顺序进行比较)。最后将排序后的字符串数组依次输出即可。
以下是可能的 `structSort` 实现方式之一,使用了标准库中的 `qsort` 函数:
```c++
int compare(const void *a, const void *b) {
return strcmp(((Value *)a)->str, ((Value *)b)->str);
}
void structSort(Value *a, int n) {
qsort(a, n, sizeof(Value), compare);
}
```
其中,`compare` 函数用于比较两个字符串的大小,将其作为参数传递给 `qsort` 函数进行排序。
阅读全文
相关推荐
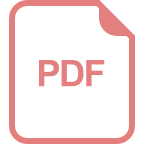
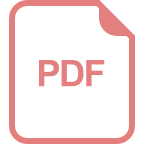
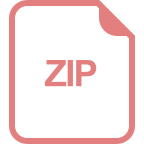











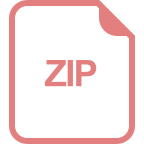