#!/usr/bin/python3 stopword = '' stri = '' try: for line in iter(input, stopword): stri += line + '\n' except EOFError: pass stri = stri[0:-1] # do something... int1_count = 0 str_count = 0 other_count = 0 kong_count = 0 for i in stri: if i.isdigit(): int1_count += 1 elif i.isalnum(): str_count += 1 elif i == ' ': kong_count += 1 else: other_count += 1 print('%d spaces, %d numbers, %d letters, %d other characters.' %(kong_count, int1_count ,str_count,other_count))里面try-except
时间: 2024-03-11 11:44:36 浏览: 9
`try-except`是Python中异常处理机制的一种形式。在`try`代码块中,我们编写可能会引发异常的代码,如果没有异常发生,则跳过`except`代码块,继续执行后面的代码;如果发生异常,则跳转到`except`代码块进行异常处理。
在这个脚本中,`try-except`语句用于实现输入循环,即循环读取用户输入的字符串,直到遇到停止符为止。如果用户输入结束,没有更多的字符串可以读取,则会引发`EOFError`异常,此时跳转到`except`代码块,使用`pass`语句跳过异常处理,程序继续执行。否则,如果用户输入了有效的字符串,则跳过`except`代码块,继续执行后面的代码。
所以,在这个脚本中,`try-except`语句的作用是保证用户输入的字符串能够被正确读取,即使用户提前结束输入或者输入的内容不符合要求。
相关问题
#!/usr/bin/python3 stopword = '' stri = '' try: for line in iter(input, stopword): stri += line + '\n' except EOFError: pass stri = stri[0:-1] # do something... int1_count = 0 str_count = 0 other_count = 0 kong_count = 0 for i in stri: if i.isdigit(): int1_count += 1 elif i.isalnum(): str_count += 1 elif i == ' ': kong_count += 1 else: other_count += 1 print('%d spaces, %d numbers, %d letters, %d other characters.' %(kong_count, int1_count ,str_count,other_count))注释一下
这是一个Python脚本,主要功能是统计用户输入的字符串中包含的空格、数字、字母和其他字符的数量,并输出统计结果。以下是代码注释:
```python
#!/usr/bin/python3 # 声明解释器类型为Python3
stopword = '' # 停止输入的标志字符串
stri = '' # 存储用户输入的字符串
try: # 开始输入循环,直到遇到停止符为止
for line in iter(input, stopword):
stri += line + '\n'
except EOFError: # 如果输入结束,则跳过输入循环
pass
stri = stri[0:-1] # 去掉字符串末尾的换行符
# 统计空格、数字、字母和其他字符的数量
int1_count = 0 # 记录数字的数量
str_count = 0 # 记录字母的数量
other_count = 0 # 记录其他字符的数量
kong_count = 0 # 记录空格的数量
for i in stri:
if i.isdigit(): # 如果是数字,则数字数量加1
int1_count += 1
elif i.isalnum(): # 如果是字母,则字母数量加1
str_count += 1
elif i == ' ': # 如果是空格,则空格数量加1
kong_count += 1
else: # 否则是其他字符,则其他字符数量加1
other_count += 1
# 输出统计结果
print('%d spaces, %d numbers, %d letters, %d other characters.' %(kong_count, int1_count ,str_count,other_count))
```
在脚本中,我们使用`try...except`语句来实现输入循环,通过`iter()`函数来实现每次输入一行,并将输入的字符串拼接到`stri`变量中。然后,我们使用`isdigit()`和`isalnum()`方法来判断字符是数字还是字母,如果是空格则直接判断,其他字符则使用`else`语句进行判断。最后,我们使用`print()`函数输出统计结果。
#!/usr/local/bin/python3 有何不足
引用中提到,#!/usr/local/bin/python3 是用来指定 python 解释器路径的。然而,这种写法存在一些不足之处。首先,使用固定路径的写法会导致在不同的机器上可能找不到正确的 python 解释器路径,从而导致脚本无法正常执行。其次,如果项目需要在多个环境中运行,每个环境的 python 解释器路径可能不同,这种写法就会变得不灵活。
相反,使用 #!/usr/bin/env python3 的写法更具灵活性。这种写法会根据系统的环境变量 PATH 去查找 python3 解释器路径,因此可以适应不同机器和不同环境的情况。这样一来,无论是在开发环境还是在部署环境,都可以确保脚本能够正常执行。
相关推荐
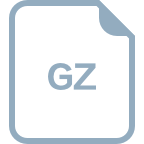
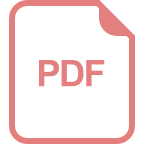
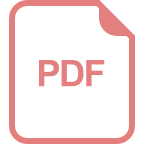













